How to map elements of the list to their indices using Java 8 streams?(如何使用 Java 8 流将列表的元素映射到它们的索引?)
问题描述
有一个字符串列表,我需要构造一个对象列表,它们实际上是对(字符串,它在列表中的位置)
.目前我有这样的代码使用谷歌收藏:
Having a list of strings, I need to construct a list of objects which are effectively pairs (string, its position in the list)
. Currently I have such code using google collections:
public Robots(List<String> names) {
ImmutableList.Builder<Robot> builder = ImmutableList.builder();
for (int i = 0; i < names.size(); i++) {
builder.add(new Robot(i, names.get(i)));
}
this.list = builder.build();
}
我想使用 Java 8 流来执行此操作.如果没有索引,我可以这样做:
I would like to do this using Java 8 streams. If there was no index, I could just do:
public Robots(List<String> names) {
this.list = names.stream()
.map(Robot::new) // no index here
.collect(collectingAndThen(
Collectors.toList(),
Collections::unmodifiableList
));
}
要获得索引,我必须这样做:
To get the index, I would have to do something like this:
public Robots(List<String> names) {
AtomicInteger integer = new AtomicInteger(0);
this.list = names.stream()
.map(string -> new Robot(integer.getAndIncrement(), string))
.collect(collectingAndThen(
Collectors.toList(),
Collections::unmodifiableList
));
}
但是,文档说映射函数应该是无状态的,但 AtomicInteger
实际上是它的状态.
However, the documentation says that mapping function should be stateless, but the AtomicInteger
is effectively its state.
有没有办法将顺序流的元素映射到它们在流中的位置?
Is there a way to map elements of the sequential stream to their positions in the stream?
推荐答案
你可以这样做:
public Robots(List<String> names) {
this.list = IntStream.range(0, names.size())
.mapToObj(i -> new Robot(i, names.get(i)))
.collect(collectingAndThen(toList(), Collections::unmodifiableList));
}
但是,根据列表的底层实现,它可能效率不高.你可以从 IntStream
中获取一个迭代器;然后在 mapToObj
中调用 next()
.
However it may not be as efficient depending on the underlying implementation of the list. You could grab an iterator from the IntStream
; then calling next()
in the mapToObj
.
作为替代方案,proton-pack 库为流定义了 zipWithIndex
功能:
As an alternative, the proton-pack library defines the zipWithIndex
functionality for streams:
this.list = StreamUtils.zipWithIndex(names.stream())
.map(i -> new Robot(i.getIndex(), i.getValue()))
.collect(collectingAndThen(toList(), Collections::unmodifiableList));
这篇关于如何使用 Java 8 流将列表的元素映射到它们的索引?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何使用 Java 8 流将列表的元素映射到它们的索引?
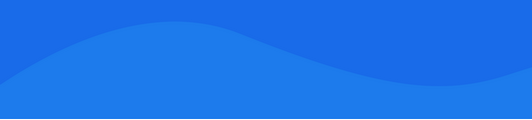
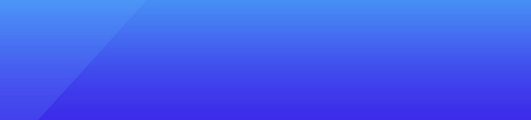
基础教程推荐
- 降序排序:Java Map 2022-01-01
- FirebaseListAdapter 不推送聊天应用程序的单个项目 - Firebase-Ui 3.1 2022-01-01
- Java Keytool 导入证书后出错,"keytool error: java.io.FileNotFoundException &拒绝访问" 2022-01-01
- “未找到匹配项"使用 matcher 的 group 方法时 2022-01-01
- 无法使用修饰符“public final"访问 java.util.Ha 2022-01-01
- Java:带有char数组的println给出乱码 2022-01-01
- 减少 JVM 暂停时间 >1 秒使用 UseConcMarkSweepGC 2022-01-01
- 设置 bean 时出现 Nullpointerexception 2022-01-01
- 在 Libgdx 中处理屏幕的正确方法 2022-01-01
- 如何使用 Java 创建 X509 证书? 2022-01-01