Why Comparator.comparing doesn#39;t work with String::toLowerCase method reference?(为什么 Comparator.comparing 不适用于 String::toLowerCase 方法参考?)
问题描述
I am trying to sort an array of Strings by reverse order (ignoring case), without modifying it, and just printing it. So I am using Java8 stream. But I can't manage to do it.
Here is my attempt :
package experimentations.chapter02;
import java.util.Arrays;
import java.util.Comparator;
import java.util.stream.Collectors;
public class StringStream {
public static void main(String[] args) {
sortStrings();
}
public static void sortStrings(){
String[] stringsArray = "The quick brown fox has a dirty ladder".split("\s+");
System.out.println(
Arrays.stream(stringsArray)
.sorted(Comparator.comparing(String::toLowerCase).reversed())
.collect(Collectors.toList())
);
}
}
The problem here is that String::toLowerCase
is not accepted in the static method Comparator.comparing
.
Meanwhile, I managed to sort the array, but modifying it:
public static void sortStrings(){
String[] stringsArray = "The quick brown fox has a dirty ladder".split("\s+");
System.out.println(
Arrays.stream(stringsArray)
.map(String::toLowerCase)
.sorted(Comparator.reverseOrder())
.collect(Collectors.toList())
);
}
So, what is the simpliest workaround?
The problem is, that Java can not deduce the generic types for some complex expressions. The first statement works, whereas the second statement leads to a compile-time error:
Comparator<String> comparator = Comparator.comparing(String::toLowerCase);
Comparator<String> comparator = Comparator.comparing(String::toLowerCase).reversed();
There are several ways to solve the problem. Here are three of them:
Store the intermediate Comparator in a variable:
Comparator<String> comparator = Comparator.comparing(String::toLowerCase);
System.out.println(
Arrays.stream(stringsArray)
.sorted(comparator.reversed())
.collect(Collectors.toList()));
Use String.CASE_INSENSITIVE_ORDER
:
System.out.println(
Arrays.stream(stringsArray)
.sorted(String.CASE_INSENSITIVE_ORDER.reversed())
.collect(Collectors.toList()));
Add explicit type parameters:
System.out.println(
Arrays.stream(stringsArray)
.sorted(Comparator.<String,String>comparing(String::toLowerCase).reversed())
.collect(Collectors.toList()));
这篇关于为什么 Comparator.comparing 不适用于 String::toLowerCase 方法参考?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:为什么 Comparator.comparing 不适用于 String::toLowerCase 方法参考?
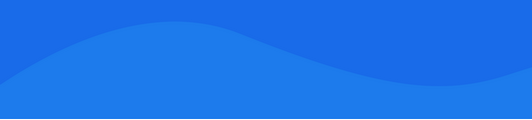
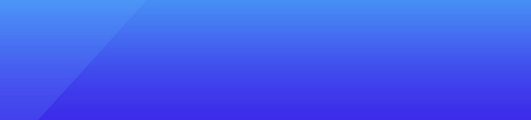
基础教程推荐
- 降序排序:Java Map 2022-01-01
- “未找到匹配项"使用 matcher 的 group 方法时 2022-01-01
- 设置 bean 时出现 Nullpointerexception 2022-01-01
- FirebaseListAdapter 不推送聊天应用程序的单个项目 - Firebase-Ui 3.1 2022-01-01
- 无法使用修饰符“public final"访问 java.util.Ha 2022-01-01
- 如何使用 Java 创建 X509 证书? 2022-01-01
- Java:带有char数组的println给出乱码 2022-01-01
- Java Keytool 导入证书后出错,"keytool error: java.io.FileNotFoundException &拒绝访问" 2022-01-01
- 在 Libgdx 中处理屏幕的正确方法 2022-01-01
- 减少 JVM 暂停时间 >1 秒使用 UseConcMarkSweepGC 2022-01-01