Unselecting RadioButtons in Java Swing(在 Java Swing 中取消选择单选按钮)
问题描述
当显示一组 JRadioButtons 时,最初没有选择它们(除非您以编程方式强制执行).即使在用户已经选择了一个按钮之后,我也希望能够将按钮恢复到该状态,即不应选择任何按钮.
When displaying a group of JRadioButtons, initially none of them is selected (unless you programmatically enforce that). I would like to be able to put buttons back into that state even after the user already selected one, i.e., none of the buttons should be selected.
但是,使用通常的嫌疑人并不能提供所需的效果:在每个按钮上调用setSelected(false)"不起作用.有趣的是,当按钮未放入 ButtonGroup 时,它确实起作用 - 不幸的是,后者是 JRadioButtons 互斥所必需的.
However, using the usual suspects doesn't deliver the required effect: calling 'setSelected(false)' on each button doesn't work. Interestingly, it does work when the buttons are not put into a ButtonGroup - unfortunately, the latter is required for JRadioButtons to be mutually exclusive.
另外,使用 setSelected(ButtonModel, boolean) - javax.swing.ButtonGroup 的方法并不能满足我的要求.
Also, using the setSelected(ButtonModel, boolean) - method of javax.swing.ButtonGroup doesn't do what I want.
我编写了一个小程序来演示效果:两个单选按钮和一个 JButton.单击 JButton 应取消选择单选按钮,以便窗口看起来与第一次弹出时完全相同.
I've put together a small program to demonstrate the effect: two radio buttons and a JButton. Clicking the JButton should unselect the radio buttons so that the window looks exactly as it does when it first pops up.
import java.awt.Container;
import java.awt.GridLayout;
import java.awt.event.*;
import javax.swing.*;
/**
* This class creates two radio buttons and a JButton. Initially, none
* of the radio buttons is selected. Clicking on the JButton should
* always return the radio buttons into that initial state, i.e.,
* should disable both radio buttons.
*/
public class RadioTest implements ActionListener {
/* create two radio buttons and a group */
private JRadioButton button1 = new JRadioButton("button1");
private JRadioButton button2 = new JRadioButton("button2");
private ButtonGroup group = new ButtonGroup();
/* clicking this button should unselect both button1 and button2 */
private JButton unselectRadio = new JButton("Unselect radio buttons.");
/* In the constructor, set up the group and event listening */
public RadioTest() {
/* put the radio buttons in a group so they become mutually
* exclusive -- without this, unselecting actually works! */
group.add(button1);
group.add(button2);
/* listen to clicks on 'unselectRadio' button */
unselectRadio.addActionListener(this);
}
/* called when 'unselectRadio' is clicked */
public void actionPerformed(ActionEvent e) {
/* variant1: disable both buttons directly.
* ...doesn't work */
button1.setSelected(false);
button2.setSelected(false);
/* variant2: disable the selection via the button group.
* ...doesn't work either */
group.setSelected(group.getSelection(), false);
}
/* Test: create a JFrame which displays the two radio buttons and
* the unselect-button */
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
RadioTest test = new RadioTest();
Container contentPane = frame.getContentPane();
contentPane.setLayout(new GridLayout(3,1));
contentPane.add(test.button1);
contentPane.add(test.button2);
contentPane.add(test.unselectRadio);
frame.setSize(400, 400);
frame.setVisible(true);
}
}
有什么想法吗?谢谢!
推荐答案
你可以这样做 buttonGroup.clearSelection()
.
You can do buttonGroup.clearSelection()
.
但是这种方法只在 Java 6 之后才可用.
But this method is available only since Java 6.
这篇关于在 Java Swing 中取消选择单选按钮的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:在 Java Swing 中取消选择单选按钮
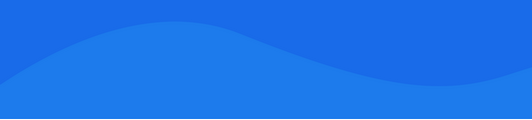
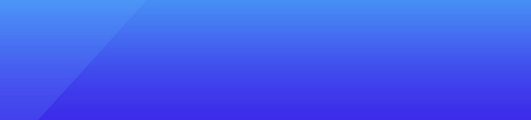
基础教程推荐
- FirebaseListAdapter 不推送聊天应用程序的单个项目 - Firebase-Ui 3.1 2022-01-01
- 如何使用 Java 创建 X509 证书? 2022-01-01
- 无法使用修饰符“public final"访问 java.util.Ha 2022-01-01
- 减少 JVM 暂停时间 >1 秒使用 UseConcMarkSweepGC 2022-01-01
- 降序排序:Java Map 2022-01-01
- Java Keytool 导入证书后出错,"keytool error: java.io.FileNotFoundException &拒绝访问" 2022-01-01
- “未找到匹配项"使用 matcher 的 group 方法时 2022-01-01
- Java:带有char数组的println给出乱码 2022-01-01
- 设置 bean 时出现 Nullpointerexception 2022-01-01
- 在 Libgdx 中处理屏幕的正确方法 2022-01-01