How do I copy a stack in Java?(如何在 Java 中复制堆栈?)
问题描述
我有一个堆栈 A,我想创建一个与堆栈 A 相同的堆栈 B.我不希望堆栈 B 只是指向 A 的指针——我实际上想创建一个新的堆栈 B,其中包含与堆栈 A 相同的元素,顺序与堆栈 A 相同.堆栈 A 是一个字符串堆栈.
I have a stack A and I want to create a stack B that is identical to stack A. I don't want stack B to simply be a pointer to A -- I actually want to create a new stack B that contains the same elements as stack A in the same order as stack A. Stack A is a stack of strings.
谢谢!
推荐答案
只需使用 Stack-class 的 clone() 方法(它实现了 Cloneable).
Just use the clone() -method of the Stack-class (it implements Cloneable).
这是一个使用 JUnit 的简单测试用例:
Here's a simple test-case with JUnit:
@Test
public void test()
{
Stack<Integer> intStack = new Stack<Integer>();
for(int i = 0; i < 100; i++)
{
intStack.push(i);
}
Stack<Integer> copiedStack = (Stack<Integer>)intStack.clone();
for(int i = 0; i < 100; i++)
{
Assert.assertEquals(intStack.pop(), copiedStack.pop());
}
}
tmsimont:这会为我创建一个未经检查或不安全的操作"警告.任何如何在不产生此问题的情况下执行此操作?
tmsimont: This creates a "unchecked or unsafe operations" warning for me. Any way to do this without generating this problem?
我起初回答说警告是不可避免的,但实际上使用 <?>
(wildcard) -typing 是可以避免的:
I at first responded that the warning would be unavoidable, but actually it is avoidable using <?>
(wildcard) -typing:
@Test
public void test()
{
Stack<Integer> intStack = new Stack<Integer>();
for(int i = 0; i < 100; i++)
{
intStack.push(i);
}
//No warning
Stack<?> copiedStack = (Stack<?>)intStack.clone();
for(int i = 0; i < 100; i++)
{
Integer value = (Integer)copiedStack.pop(); //Won't cause a warning, no matter to which type you cast (String, Float...), but will throw ClassCastException at runtime if the type is wrong
Assert.assertEquals(intStack.pop(), value);
}
}
基本上我会说您仍在进行从 ?
(未知类型)到 Integer
的未经检查的强制转换,但没有警告.就个人而言,我仍然更喜欢直接转换为 Stack<Integer>
并使用 @SuppressWarnings("unchecked")
抑制警告.
Basically I'd say you're still doing an unchecked cast from ?
(unknown type) to Integer
, but there's no warning. Personally, I'd still prefer to cast directly into Stack<Integer>
and suppress the warning with @SuppressWarnings("unchecked")
.
这篇关于如何在 Java 中复制堆栈?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何在 Java 中复制堆栈?
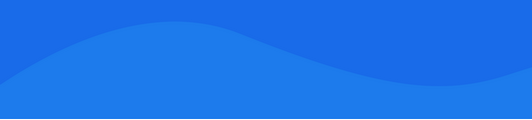
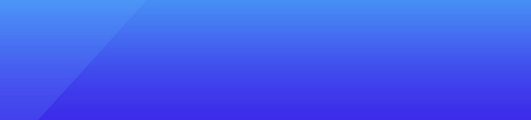
基础教程推荐
- “未找到匹配项"使用 matcher 的 group 方法时 2022-01-01
- 设置 bean 时出现 Nullpointerexception 2022-01-01
- Java Keytool 导入证书后出错,"keytool error: java.io.FileNotFoundException &拒绝访问" 2022-01-01
- 如何使用 Java 创建 X509 证书? 2022-01-01
- Java:带有char数组的println给出乱码 2022-01-01
- 在 Libgdx 中处理屏幕的正确方法 2022-01-01
- 减少 JVM 暂停时间 >1 秒使用 UseConcMarkSweepGC 2022-01-01
- 无法使用修饰符“public final"访问 java.util.Ha 2022-01-01
- 降序排序:Java Map 2022-01-01
- FirebaseListAdapter 不推送聊天应用程序的单个项目 - Firebase-Ui 3.1 2022-01-01