Getting an element from a Set(从 Set 中获取元素)
问题描述
Set
为什么不提供一个操作来获取一个等于另一个元素的元素?
Why doesn't Set
provide an operation to get an element that equals another element?
Set<Foo> set = ...;
...
Foo foo = new Foo(1, 2, 3);
Foo bar = set.get(foo); // get the Foo element from the Set that equals foo
我可以问 Set
是否包含一个等于 bar
的元素,那为什么我不能得到那个元素呢?:(
I can ask whether the Set
contains an element equal to bar
, so why can't I get that element? :(
为了澄清,equals
方法被覆盖,但它只检查其中一个字段,而不是全部.所以两个被认为相等的 Foo
对象实际上可以有不同的值,这就是为什么我不能只使用 foo
.
To clarify, the equals
method is overridden, but it only checks one of the fields, not all. So two Foo
objects that are considered equal can actually have different values, that's why I can't just use foo
.
推荐答案
如果元素相等,那么获取元素就没有意义了.Map
更适合这个用例.
There would be no point of getting the element if it is equal. A Map
is better suited for this usecase.
如果你仍然想找到元素,你别无选择,只能使用迭代器:
If you still want to find the element you have no other option but to use the iterator:
public static void main(String[] args) {
Set<Foo> set = new HashSet<Foo>();
set.add(new Foo("Hello"));
for (Iterator<Foo> it = set.iterator(); it.hasNext(); ) {
Foo f = it.next();
if (f.equals(new Foo("Hello")))
System.out.println("foo found");
}
}
static class Foo {
String string;
Foo(String string) {
this.string = string;
}
@Override
public int hashCode() {
return string.hashCode();
}
@Override
public boolean equals(Object obj) {
return string.equals(((Foo) obj).string);
}
}
这篇关于从 Set 中获取元素的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:从 Set 中获取元素
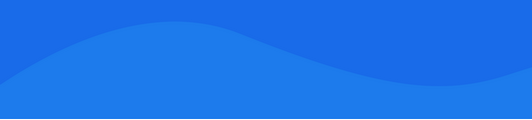
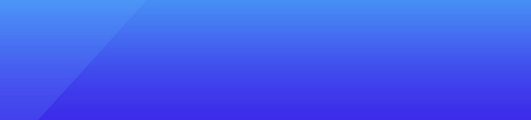
基础教程推荐
- 降序排序:Java Map 2022-01-01
- FirebaseListAdapter 不推送聊天应用程序的单个项目 - Firebase-Ui 3.1 2022-01-01
- Java:带有char数组的println给出乱码 2022-01-01
- 设置 bean 时出现 Nullpointerexception 2022-01-01
- “未找到匹配项"使用 matcher 的 group 方法时 2022-01-01
- 在 Libgdx 中处理屏幕的正确方法 2022-01-01
- 无法使用修饰符“public final"访问 java.util.Ha 2022-01-01
- 如何使用 Java 创建 X509 证书? 2022-01-01
- 减少 JVM 暂停时间 >1 秒使用 UseConcMarkSweepGC 2022-01-01
- Java Keytool 导入证书后出错,"keytool error: java.io.FileNotFoundException &拒绝访问" 2022-01-01