How to mock FileInputStream and other *Streams(如何模拟 FileInputStream 和其他 *Streams)
问题描述
我有一个获取 GenericFile 作为输入参数的类,它读取数据并进行一些额外的处理.我需要测试一下:
I have class that gets GenericFile as input argument reads data and does some additional processing. I need to test it:
public class RealCardParser {
public static final Logger l = LoggerFactory.getLogger(RealCardParser.class);
@Handler
public ArrayList<String> handle(GenericFile genericFile) throws IOException {
ArrayList<String> strings = new ArrayList<String>();
FileInputStream fstream = new FileInputStream((File) genericFile.getFile());
DataInputStream in = new DataInputStream(fstream);
BufferedReader br = new BufferedReader(new InputStreamReader(in));
String strLine = br.readLine();//skip header
while ((strLine = br.readLine()) != null) {
l.info("handling in parser: {}", strLine);
strings.add(strLine);
}
br.close();
return strings;
}
}
问题在于新的 FileInputStream.我可以模拟 GenericFile 但它没有用,因为 FileInputStream 检查文件是否存在.我改变了我的班级:
The issue is with new FileInputStream. I can mock GenericFile but it is useless cause FileInputStream checks if file exists. I changed my class so:
public class RealCardParser {
public static final Logger l = LoggerFactory.getLogger(RealCardParser.class);
protected BufferedReader getBufferedReader(GenericFile genericFile) throws FileNotFoundException {
FileInputStream fstream = new FileInputStream((File) genericFile.getFile());
DataInputStream in = new DataInputStream(fstream);
return new BufferedReader(new InputStreamReader(in));
}
@Handler
public ArrayList<String> handle(GenericFile genericFile) throws IOException {
ArrayList<String> strings = new ArrayList<String>();
BufferedReader br = getBufferedReader(genericFile);
String strLine = br.readLine();//skip header
while ((strLine = br.readLine()) != null) {
l.info("handling in parser: {}", strLine);
strings.add(strLine);
}
br.close();
return strings;
}
}
所以现在我可以重写方法 getBufferedReader 和测试方法处理程序:
So now I can override method getBufferedReader and test method handler:
@RunWith(MockitoJUnitRunner.class)
public class RealCardParserTest {
RealCardParser parser;
@Mock
GenericFile genericFile;
@Mock
BufferedReader bufferedReader;
@Mock
File file;
@Before
public void setUp() throws Exception {
parser = new RealCardParser() {
@Override
public BufferedReader getBufferedReader(GenericFile genericFile) throws FileNotFoundException {
return bufferedReader;
}
};
when(genericFile.getFile()).thenReturn(file);
when(bufferedReader.readLine()).thenReturn("header").thenReturn("1,2,3").thenReturn(null);
}
@Test
public void testParser() throws Exception {
parser.handle(genericFile);
//do some asserts
}
}
Handler 方法现在已经被测试覆盖了,但我仍然发现了导致 cobertura 问题的方法 getBufferedReader.如何测试方法 getBufferedReader 或者是否有其他解决方案?
Handler method now is covered with tests, but I still have uncovered method getBufferedReader that leads to cobertura problems. How to test method getBufferedReader or maybe there is another solution of the problem?
推荐答案
您可以使用 PowerMockRunner 和 PowerMockito 模拟 FileInputStream.请参阅下面的模拟代码-
You can mock FileInputStream by using PowerMockRunner and PowerMockito. See the below code for mocking-
@RunWith(PowerMockRunner.class)
@PrepareForTest({
FileInputStream.class
})
public class A{
@Test
public void testFileInputStream ()
throws Exception
{
final FileInputStream fileInputStreamMock = PowerMockito.mock(FileInputStream.class);
PowerMockito.whenNew(FileInputStream.class).withArguments(Matchers.anyString())
.thenReturn(fileInputStreamMock);
//Call the actual method containing the new constructor of FileInputStream
}
}
这篇关于如何模拟 FileInputStream 和其他 *Streams的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何模拟 FileInputStream 和其他 *Streams
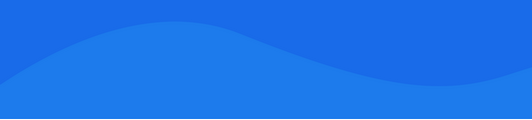
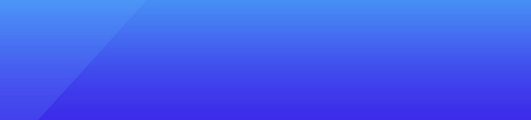
基础教程推荐
- Java:带有char数组的println给出乱码 2022-01-01
- 设置 bean 时出现 Nullpointerexception 2022-01-01
- 减少 JVM 暂停时间 >1 秒使用 UseConcMarkSweepGC 2022-01-01
- 在 Libgdx 中处理屏幕的正确方法 2022-01-01
- 降序排序:Java Map 2022-01-01
- “未找到匹配项"使用 matcher 的 group 方法时 2022-01-01
- Java Keytool 导入证书后出错,"keytool error: java.io.FileNotFoundException &拒绝访问" 2022-01-01
- 无法使用修饰符“public final"访问 java.util.Ha 2022-01-01
- 如何使用 Java 创建 X509 证书? 2022-01-01
- FirebaseListAdapter 不推送聊天应用程序的单个项目 - Firebase-Ui 3.1 2022-01-01