Java seek a method with specific annotation and its annotation element(Java 寻找具有特定注解的方法及其注解元素)
问题描述
Suppose I have this annotation class
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface MethodXY {
public int x();
public int y();
}
public class AnnotationTest {
@MethodXY(x=5, y=5)
public void myMethodA(){ ... }
@MethodXY(x=3, y=2)
public void myMethodB(){ ... }
}
So is there a way to look into an object, "seek" out the method with the @MethodXY annotation, where its element x = 3, y = 2, and invoke it?
Thanks
Here is a method, which returns methods with specific annotations:
public static List<Method> getMethodsAnnotatedWith(final Class<?> type, final Class<? extends Annotation> annotation) {
final List<Method> methods = new ArrayList<Method>();
Class<?> klass = type;
while (klass != Object.class) { // need to iterated thought hierarchy in order to retrieve methods from above the current instance
// iterate though the list of methods declared in the class represented by klass variable, and add those annotated with the specified annotation
for (final Method method : klass.getDeclaredMethods()) {
if (method.isAnnotationPresent(annotation)) {
Annotation annotInstance = method.getAnnotation(annotation);
// TODO process annotInstance
methods.add(method);
}
}
// move to the upper class in the hierarchy in search for more methods
klass = klass.getSuperclass();
}
return methods;
}
It can be easily modified to your specific needs. Pls note that the provided method traverses class hierarchy in order to find methods with required annotations.
Here is a method for your specific needs:
public static List<Method> getMethodsAnnotatedWithMethodXY(final Class<?> type) {
final List<Method> methods = new ArrayList<Method>();
Class<?> klass = type;
while (klass != Object.class) { // need to iterated thought hierarchy in order to retrieve methods from above the current instance
// iterate though the list of methods declared in the class represented by klass variable, and add those annotated with the specified annotation
for (final Method method : klass.getDeclaredMethods()) {
if (method.isAnnotationPresent(MethodXY.class)) {
MethodXY annotInstance = method.getAnnotation(MethodXY.class);
if (annotInstance.x() == 3 && annotInstance.y() == 2) {
methods.add(method);
}
}
}
// move to the upper class in the hierarchy in search for more methods
klass = klass.getSuperclass();
}
return methods;
}
For invocation of the found method(s) pls refer a tutorial. One of the potential difficulties here is the number of method arguments, which could vary between found methods and thus requiring some additional processing.
这篇关于Java 寻找具有特定注解的方法及其注解元素的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:Java 寻找具有特定注解的方法及其注解元素
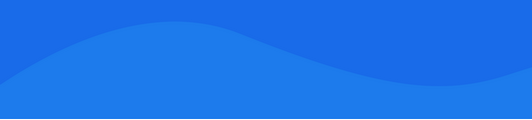
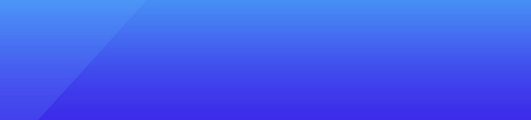
基础教程推荐
- 在 Libgdx 中处理屏幕的正确方法 2022-01-01
- FirebaseListAdapter 不推送聊天应用程序的单个项目 - Firebase-Ui 3.1 2022-01-01
- 降序排序:Java Map 2022-01-01
- 减少 JVM 暂停时间 >1 秒使用 UseConcMarkSweepGC 2022-01-01
- “未找到匹配项"使用 matcher 的 group 方法时 2022-01-01
- Java Keytool 导入证书后出错,"keytool error: java.io.FileNotFoundException &拒绝访问" 2022-01-01
- 无法使用修饰符“public final"访问 java.util.Ha 2022-01-01
- Java:带有char数组的println给出乱码 2022-01-01
- 如何使用 Java 创建 X509 证书? 2022-01-01
- 设置 bean 时出现 Nullpointerexception 2022-01-01