How to create a hex color string UIColor initializer in Swift?(如何在 Swift 中创建十六进制颜色字符串 UIColor 初始化程序?)
问题描述
我正在使用此代码从十六进制值创建 UIColor.它工作得很好.
I am using this code for create UIColor from hex value. Its working perfectly.
extension UIColor {
convenience init(red: Int, green: Int, blue: Int) {
assert(red >= 0 && red <= 255, "Invalid red component")
assert(green >= 0 && green <= 255, "Invalid green component")
assert(blue >= 0 && blue <= 255, "Invalid blue component")
self.init(red: CGFloat(red) / 255.0, green: CGFloat(green) / 255.0, blue: CGFloat(blue) / 255.0, alpha: 1.0)
}
convenience init(netHex:Int) {
self.init(red:(netHex >> 16) & 0xff, green:(netHex >> 8) & 0xff, blue:netHex & 0xff)
}
}
用法:
var textColor = UIColor(netHex: 0xffffff)
此代码非常适用于 Int 十六进制代码.但它需要十六进制代码 0xffffff 作为 Int 类型.我有来自网络服务的十六进制代码.它就像#ffffff"(字符串不是 Int).我可以将此字符串转换为0xffffff".但我无法从0xffffff"(字符串)转换为 0xffffff(整数).
This code works perfectly for Int hex code. But It needs hex code 0xffffff as Int type. I am having the hex code from web service. It will be like "#ffffff" (String not Int). I can convert this string like "0xffffff". But I can't convert from "0xffffff"(String) to 0xffffff (Int).
我需要这样的东西
var textColor = UIColor(netHex: "0xffffff")
或者更好的是:
var textColor = UIColor(netHex: "#ffffff")
提前致谢.
推荐答案
Xcode 9 • Swift 4 或更高版本
extension UIColor {
convenience init?(hexaRGB: String, alpha: CGFloat = 1) {
var chars = Array(hexaRGB.hasPrefix("#") ? hexaRGB.dropFirst() : hexaRGB[...])
switch chars.count {
case 3: chars = chars.flatMap { [$0, $0] }
case 6: break
default: return nil
}
self.init(red: .init(strtoul(String(chars[0...1]), nil, 16)) / 255,
green: .init(strtoul(String(chars[2...3]), nil, 16)) / 255,
blue: .init(strtoul(String(chars[4...5]), nil, 16)) / 255,
alpha: alpha)
}
convenience init?(hexaRGBA: String) {
var chars = Array(hexaRGBA.hasPrefix("#") ? hexaRGBA.dropFirst() : hexaRGBA[...])
switch chars.count {
case 3: chars = chars.flatMap { [$0, $0] }; fallthrough
case 6: chars.append(contentsOf: ["F","F"])
case 8: break
default: return nil
}
self.init(red: .init(strtoul(String(chars[0...1]), nil, 16)) / 255,
green: .init(strtoul(String(chars[2...3]), nil, 16)) / 255,
blue: .init(strtoul(String(chars[4...5]), nil, 16)) / 255,
alpha: .init(strtoul(String(chars[6...7]), nil, 16)) / 255)
}
convenience init?(hexaARGB: String) {
var chars = Array(hexaARGB.hasPrefix("#") ? hexaARGB.dropFirst() : hexaARGB[...])
switch chars.count {
case 3: chars = chars.flatMap { [$0, $0] }; fallthrough
case 6: chars.append(contentsOf: ["F","F"])
case 8: break
default: return nil
}
self.init(red: .init(strtoul(String(chars[2...3]), nil, 16)) / 255,
green: .init(strtoul(String(chars[4...5]), nil, 16)) / 255,
blue: .init(strtoul(String(chars[6...7]), nil, 16)) / 255,
alpha: .init(strtoul(String(chars[0...1]), nil, 16)) / 255)
}
}
<小时>
if let textColor = UIColor(hexa: "00F") {
print(textColor) // r 0.0 g 0.0 b 1.0 a 1.0
}
<小时>
if let textColor = UIColor(hexaRGB: "00F") {
print(textColor) // r 0.0 g 0.0 b 1.0 a 1.0
}
UIColor(hexaRGB: "#00F") // r 0.0 g 0.0 b 1.0 a 1.0
UIColor(hexaRGB: "#00F", alpha: 0.5) // r 0.0 g 0.0 b 1.0 a 0.5
UIColor(hexaRGB: "#0000FF") // r 0.0 g 0.0 b 1.0 a 1.0
UIColor(hexaRGB: "#0000FF", alpha: 0.5) // r 0.0 g 0.0 b 1.0 a 0.5
UIColor(hexaRGBA: "#0000FFFF") // r 0.0 g 0.0 b 1.0 a 1.0
UIColor(hexaRGBA: "#0000FF7F") // r 0.0 g 0.0 b 1.0 a 0.498
UIColor(hexaARGB: "#FF0000FF") // r 0.0 g 0.0 b 1.0 a 1.0
UIColor(hexaARGB: "#7F0000FF") // r 0.0 g 0.0 b 1.0 a 0.498
这篇关于如何在 Swift 中创建十六进制颜色字符串 UIColor 初始化程序?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何在 Swift 中创建十六进制颜色字符串 UIColor 初
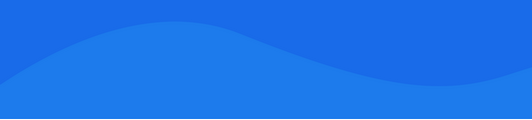
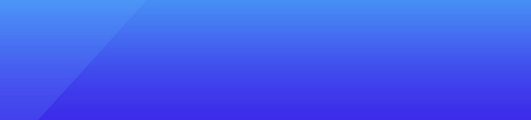
基础教程推荐
- 如何在 iPhone 上显示来自 API 的 HTML 文本? 2022-01-01
- 如何在没有IB的情况下将2个按钮添加到右侧的UINavigationbar? 2022-01-01
- 在 gmail 中为 ios 应用程序检索朋友的朋友 2022-01-01
- Android:对话框关闭而不调用关闭 2022-01-01
- 如何让对象对 Cocos2D 中的触摸做出反应? 2022-01-01
- 如何在 UIImageView 中异步加载图像? 2022-01-01
- Kivy Buildozer 无法构建 apk,命令失败:./distribute.sh -m “kivy"d 2022-01-01
- 当从同一个组件调用时,两个 IBAction 触发的顺序是什么? 2022-01-01
- android 应用程序已发布,但在 google play 中找不到 2022-01-01
- UIWebView 委托方法 shouldStartLoadWithRequest:在 WKWebView 中等效? 2022-01-01