UITableViewCell With UIWebView Dynamic Height(UITableViewCell 与 UIWebView 动态高度)
问题描述
我有一个表格视图,其中包含有 webView 的单元格,我希望单元格的高度与 webView 的高度相匹配.
I have a table view with cells that have a webView in them, I want the height of the cell to match the height of the webView.
这是我使用的代码:
func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCellWithIdentifier("newsCell") as! NewsTableViewCell
cell.webView.loadHTMLString("test<br>test<br>test<br>test<br>test<br>test", baseURL: nil)
cell.webView.delegate = self
var webFrame = cell.webView.frame
var cellFrame = cell.frame
cell.frame = CGRectMake(cellFrame.origin.x, cellFrame.origin.y, cellFrame.width, webFrame.height + 20)
cell.backgroundColor = UIColor.redColor()
return cell
}
func webViewDidFinishLoad(webView: UIWebView) {
println("finished loading")
var frame = webView.frame
frame.size.height = 1
webView.frame = frame
var fittingSize = webView.sizeThatFits(CGSizeZero)
frame.size = fittingSize
webView.frame = frame
var height: CGFloat = frame.height
println(height)
webView.frame = CGRectMake(frame.origin.x, frame.origin.x, frame.size.width, frame.size.height)
newsTable.beginUpdates()
newsTable.endUpdates()
}
结果如下:https://postimg.cc/image/8qew1lqjj/
webView 是正确的高度,但单元格不是,我该如何解决这个问题?
The webView is the correct height but the cell isn't, How can I fix this problem?
推荐答案
TableView 会自己调整单元格大小,你只需要实现 tableView(tableView: UITableView, heightForRowAtIndexPath indexPath: NSIndexPath) ->CGFloat
委托方法.
TableView will resize cells itself, you just need implement tableView(tableView: UITableView, heightForRowAtIndexPath indexPath: NSIndexPath) -> CGFloat
delegate method.
是的,你最初并不知道WebView的高度,但是你可以计算出来然后让TableView重新加载cell.像这样的:
Yes, you don't know the height of WebView initially, but you can calculate it and then ask TableView to reload cell. Something like this:
class TableViewController: UITableViewController, UIWebViewDelegate
{
var content : [String] = ["test1<br>test1<br>test1<br>test1<br>test1<br>test1", "test22<br>test22<br>test22<br>test22<br>test22<br>test22"]
var contentHeights : [CGFloat] = [0.0, 0.0]
// ...
override func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell
{
let cell = tableView.dequeueReusableCellWithIdentifier("newsCell", forIndexPath: indexPath) as! NewsTableViewCell
let htmlString = content[indexPath.row]
let htmlHeight = contentHeights[indexPath.row]
cell.webView.tag = indexPath.row
cell.webView.delegate = self
cell.webView.loadHTMLString(htmlString, baseURL: nil)
cell.webView.frame = CGRectMake(0, 0, cell.frame.size.width, htmlHeight)
return cell
}
override func tableView(tableView: UITableView, heightForRowAtIndexPath indexPath: NSIndexPath) -> CGFloat
{
return contentHeights[indexPath.row]
}
func webViewDidFinishLoad(webView: UIWebView)
{
if (contentHeights[webView.tag] != 0.0)
{
// we already know height, no need to reload cell
return
}
contentHeights[webView.tag] = webView.scrollView.contentSize.height
tableView.reloadRowsAtIndexPaths([NSIndexPath(forRow: webView.tag, inSection: 0)], withRowAnimation: .Automatic)
}
// ...
}
这篇关于UITableViewCell 与 UIWebView 动态高度的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:UITableViewCell 与 UIWebView 动态高度
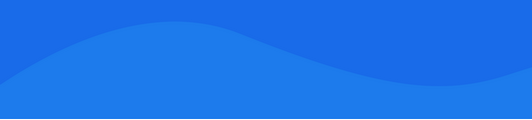
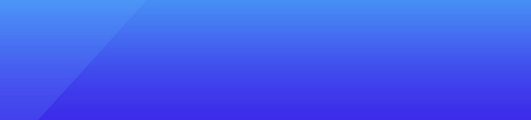
基础教程推荐
- 如何在没有IB的情况下将2个按钮添加到右侧的UINavigationbar? 2022-01-01
- Kivy Buildozer 无法构建 apk,命令失败:./distribute.sh -m “kivy"d 2022-01-01
- Android:对话框关闭而不调用关闭 2022-01-01
- 如何在 iPhone 上显示来自 API 的 HTML 文本? 2022-01-01
- 在 gmail 中为 ios 应用程序检索朋友的朋友 2022-01-01
- 如何在 UIImageView 中异步加载图像? 2022-01-01
- 如何让对象对 Cocos2D 中的触摸做出反应? 2022-01-01
- android 应用程序已发布,但在 google play 中找不到 2022-01-01
- 当从同一个组件调用时,两个 IBAction 触发的顺序是什么? 2022-01-01
- UIWebView 委托方法 shouldStartLoadWithRequest:在 WKWebView 中等效? 2022-01-01