UITableview buttons getting highlighted on scroll(UITableview 按钮在滚动时突出显示)
问题描述
I have toggle buttons in my tableview cells and I click them on for some cells but when I scroll down, those same buttons are selected for the bottom cells as well even though I didn't select them yet. I know this is happening because of the tableview reusing cells...is there any way I can fix this?
The cells are dynamic, not static.
what the tableview looks like
** EDIT: Also, lemme know if my logic seems alright: I tried creating a mutable array in my viewcontroller class and then setting all it's values to @"0". Then, in my tableviewcell's class, I set the value in the array to @"1" at the index of the current cell if I select the button, so then back in my viewcontroller class, I can tell if I have already selected a button at that cell or not. The only flaw is that I can't access the array in my tableviewcell's class, it is coming out at null...i guess that it because of the mvc pattern in objective c. Any advice?
EDIT
I am still unable to resolve my issue. Can someone please help me? I have been stuck on it for a while now!
I am trying to create a tableview where the cells have a check and cross button and when I click the check button, it should turn green, but the same button in other cells should remain gray, however, when I scroll down, some cells that I didn't select buttons in still turn green...because of cell recycling.
I am using delegates and protocols right now but it isn't working; perhaps I am using it wrong?
I am setting yesChecked value in IBaction functions in my cell class, and in my viewcontroller class, I am using that yesChecked value to see what color to give to the button based on whether it says "yes" or "no".
Kindly help! Thanks!
@protocol DetailsTableViewCellDelegate <NSObject>
- (void) customCell:(DetailsTableViewCell *)cell yesBtnPressed:(bool)yes;
@property (nonatomic, retain) NSString * yesChecked;
You'd have to select or deselect them in cellForRowAt
. For example if your cell had a leftButton
property and you had a model like this, you could do something like the following:
@interface Model : NSObject
@property (nonatomic, assign) BOOL selected;
@end
@protocol CustomCellDelegate <NSObject>
- (void)cellActionTapped:(UITableViewCell *)cell;
@end
@interface CustomCell : UITableViewCell
@property (nonatomic, assign) BOOL leftButtonSelected;
@property (weak, nonatomic, nullable) id<CustomCellDelegate> delegate;
@end
// ModelViewController.h
@interface ModelViewController : UIViewController<CustomCellDelegate>
@end
// ModelViewController.m
@interface ViewController () {
NSArray<Model*>* models;
}
@end
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath {
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:@"reuseIdentifier"];
((CustomCell *)cell).delegate = self;
((CustomCell *)cell).leftButtonSelected = models[indexPath.row].selected;
return cell;
}
- (void)cellActionTapped:(UITableViewCell *)cell {
NSIndexPath *indexPath = [tableView indexPathForCell:cell];
// Update data source using (maybe) indexPath.row
}
这篇关于UITableview 按钮在滚动时突出显示的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:UITableview 按钮在滚动时突出显示
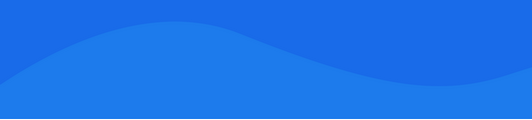
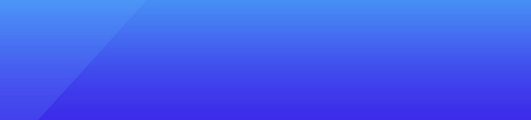
基础教程推荐
- 如何在没有IB的情况下将2个按钮添加到右侧的UINavigationbar? 2022-01-01
- 如何让对象对 Cocos2D 中的触摸做出反应? 2022-01-01
- UIWebView 委托方法 shouldStartLoadWithRequest:在 WKWebView 中等效? 2022-01-01
- Android:对话框关闭而不调用关闭 2022-01-01
- 如何在 iPhone 上显示来自 API 的 HTML 文本? 2022-01-01
- 当从同一个组件调用时,两个 IBAction 触发的顺序是什么? 2022-01-01
- android 应用程序已发布,但在 google play 中找不到 2022-01-01
- Kivy Buildozer 无法构建 apk,命令失败:./distribute.sh -m “kivy"d 2022-01-01
- 在 gmail 中为 ios 应用程序检索朋友的朋友 2022-01-01
- 如何在 UIImageView 中异步加载图像? 2022-01-01