How to Save images to ImageView using Shared Preferences(如何使用共享首选项将图像保存到 ImageView)
问题描述
我有一个活动可以打开另一个活动以获取下载图片.图片回到我原来的活动并在 imageView 中休息.这工作正常.如何保存图像,以便用户稍后返回或杀死应用程序时图像仍然存在.我知道我应该使用共享首选项来获取图像路径而不是保存图像本身,但我只是不知道该怎么做.
I have an activity that opens another activity to get a download pic. The picture comes back to my original activity and rest in an imageView. That's working fine. How do I save the image so when the user comes back later, or kills to app the image is still there. I know I am supposed the use Shared Preferences to get the image path and not save the image itself but I just don't know how do that.
主要活动
public class MainActivity extends Activity {
private static final int REQUEST_CODE = 1;
private Bitmap bitmap;
Button button;
ImageView imageView;
String selectedImagePath;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
button=(Button) findViewById(R.id.click);
imageView=(ImageView) findViewById(R.id.image);
imageView.setOnLongClickListener(new View.OnLongClickListener() {
@Override
public boolean onLongClick(View v) {
Switch();
return true;
}
});
}
public void Switch(){
imageView = (ImageView) findViewById(R.id.image);
Intent intent = new Intent(Intent.ACTION_PICK, MediaStore.Images.Media.EXTERNAL_CONTENT_URI);
startActivityForResult(intent, REQUEST_CODE);
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
if (requestCode==REQUEST_CODE&&resultCode== Activity.RESULT_OK){
try {
Uri selectedImage = data.getData();
String[] filePathColumn = {MediaStore.Images.Media.DATA};
Cursor cursor = getContentResolver().query(selectedImage, filePathColumn, null, null, null);
cursor.moveToFirst();
int columnIndex = cursor.getColumnIndex(filePathColumn[0]);
String filePath = cursor.getString(columnIndex);
Log.v("roni", filePath);
cursor.close();
if(bitmap != null && !bitmap.isRecycled())
{
bitmap = null;
}
bitmap = BitmapFactory.decodeFile(filePath);
//imageView.setBackgroundResource(0);
imageView.setImageBitmap(bitmap);
} catch (Exception e){
e.printStackTrace();
}
}
}
@Override
protected void onPause() {
super.onPause();
save();
}
public void save() {
SharedPreferences sp = getSharedPreferences("AppSharedPref", 1); // Open SharedPreferences with name AppSharedPref
SharedPreferences.Editor editor = sp.edit();
editor.putString("ImagePath", selectedImagePath); // Store selectedImagePath with key "ImagePath". This key will be then used to retrieve data.
editor.commit();
}
@Override
protected void onResume() {
super.onResume();
restore();
}
public void restore(){
SharedPreferences sp = getSharedPreferences("AppSharedPref", 1);
selectedImagePath = sp.getString("ImagePath", "");
bitmap = BitmapFactory.decodeFile(selectedImagePath);
imageView.setImageBitmap(bitmap);
}
}
查看活动
public class ViewActivity extends ActionBarActivity {
ImageButton imageViews;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_view);
imageViews = (ImageButton) findViewById(R.id.image);
Intent intent = getIntent();
Uri data = intent.getData();
if (intent.getType().indexOf("image/") != -1)
{
imageViews.setImageURI(data);
}
}
推荐答案
编写方法将您的位图编码为字符串 base64
Write Method to encode your Bitmap into string base64
public static String encodeToBase64(Bitmap image) {
Bitmap immage = image;
ByteArrayOutputStream baos = new ByteArrayOutputStream();
immage.compress(Bitmap.CompressFormat.PNG, 100, baos);
byte[] b = baos.toByteArray();
String imageEncoded = Base64.encodeToString(b, Base64.DEFAULT);
Log.d("Image Log:", imageEncoded);
return imageEncoded;
}
在此方法中传递 yourBitmap,就像您偏好的 encodeTobase64
一样
Pass yourBitmap inside this method like something encodeTobase64
in your preference
SharedPreferences myPrefrence = getPreferences(MODE_PRIVATE);
SharedPreferences.Editor editor = myPrefrence.edit();
editor.putString("namePreferance", itemNAme);
editor.putString("imagePreferance", encodeToBase64(yourBitmap));
editor.commit();
当你想在任何地方显示你的图像时,只需使用 decodeToBase64
方法再次将其转换为 Bitmap
And when you want any where display your image just convert it into Bitmap
again using decodeToBase64
method
public static Bitmap decodeToBase64(String input) {
byte[] decodedByte = Base64.decode(input, 0);
return BitmapFactory.decodeByteArray(decodedByte, 0, decodedByte.length);
}
这里是再次获取 Bitmap 的代码
here is code to get Bitmap again
SharedPreferences myPrefrence = getPreferences(MODE_PRIVATE);
String imageS = myPrefrence.getString("imagePreferance", "");
Bitmap imageB;
if(!imageS.equals("")) imageB = decodeToBase64(imageS);
但在我看来,您应该保留在共享首选项中的唯一位图路径.
But in my opinion you should keep inside shared preference only path to bitmap.
这篇关于如何使用共享首选项将图像保存到 ImageView的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何使用共享首选项将图像保存到 ImageView
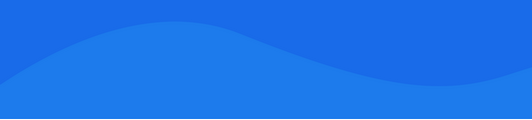
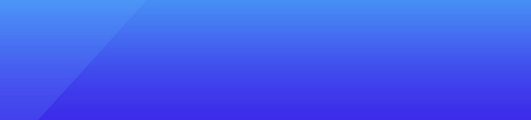
基础教程推荐
- 如何在 iPhone 上显示来自 API 的 HTML 文本? 2022-01-01
- android 应用程序已发布,但在 google play 中找不到 2022-01-01
- 当从同一个组件调用时,两个 IBAction 触发的顺序是什么? 2022-01-01
- 在 gmail 中为 ios 应用程序检索朋友的朋友 2022-01-01
- Kivy Buildozer 无法构建 apk,命令失败:./distribute.sh -m “kivy"d 2022-01-01
- 如何让对象对 Cocos2D 中的触摸做出反应? 2022-01-01
- 如何在没有IB的情况下将2个按钮添加到右侧的UINavigationbar? 2022-01-01
- Android:对话框关闭而不调用关闭 2022-01-01
- 如何在 UIImageView 中异步加载图像? 2022-01-01
- UIWebView 委托方法 shouldStartLoadWithRequest:在 WKWebView 中等效? 2022-01-01