How to use addHeaderView to add a simple ImageView to a ListView(如何使用 addHeaderView 将简单的 ImageView 添加到 ListView)
问题描述
My target is simple. I want to add a "Red Rectangle" as a headerview to a ListView
.
so I create a simple activity
public class MainActivity extends Activity {
private String[] adapterData = new String[] { "Afghanistan", "Albania", "Algeria",
"American Samoa", "Andorra", "Angola"};
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ListView lv;
lv = (ListView)findViewById(R.id.list);
LayoutInflater lf;
View headerView;
lf = this.getLayoutInflater();
headerView = (View)lf.inflate(R.layout.header, null, false);
lv.addHeaderView(headerView, null, false);
lv.setAdapter(new ArrayAdapter<String>(this, R.layout.list_item, adapterData));
}
}
activity_main.xml
<ListView xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/list"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#FF0"
android:cacheColorHint="#0000"
android:paddingLeft="20dip"
android:paddingRight="20dip"
android:divider="#0000"
android:scrollbarStyle="outsideOverlay" />
list_item.xml
<TextView xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@android:id/text1"
android:layout_width="match_parent"
android:layout_height="0dip"
android:minHeight="40dip"
android:singleLine="true"
android:textAppearance="?android:attr/textAppearanceLarge"
android:gravity="center_vertical"
/>
most important, header.xml
<?xml version="1.0" encoding="utf-8"?>
<ImageView xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="100dip"
android:background="#F00"/>
But it doesn't seem to work. Nothing is displayed.
Can any give some suggestions on this? Your help is highly appreciated.
Old question, but I just copied it and run it and indeed the red doesn't show up.
You're missing one simple thing: Regardless of how you call inflate
you always1 have to pass a parent view (second param) so that it can figure out which LayoutParams
subclass to instantiate.
1: there are always1 exceptions
.inflate(R.layout.header, lv, false)
For more on the subject I highly suggest: http://www.doubleencore.com/2013/05/layout-inflation-as-intended/ (worth reading even a few times)
Your full code modified (xml files can stay as is):
public class MainActivity extends Activity {
private static final String[] ADAPTER_DATA = {
"Afghanistan", "Albania", "Algeria", "American Samoa", "Andorra", "Angola"
};
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ListView list = (ListView) findViewById(R.id.list);
View header = getLayoutInflater().inflate(R.layout.header, list, false);
list.addHeaderView(header, null, false);
list.setAdapter(new ArrayAdapter<String>(this, R.layout.list_item, ADAPTER_DATA));
}
}
这篇关于如何使用 addHeaderView 将简单的 ImageView 添加到 ListView的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何使用 addHeaderView 将简单的 ImageView 添加到 ListView
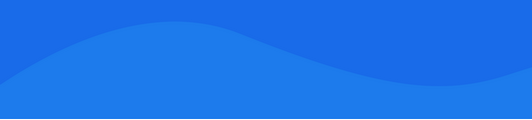
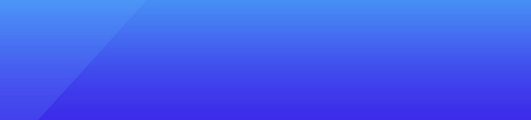
基础教程推荐
- 如何在 UIImageView 中异步加载图像? 2022-01-01
- android 应用程序已发布,但在 google play 中找不到 2022-01-01
- 如何让对象对 Cocos2D 中的触摸做出反应? 2022-01-01
- 在 gmail 中为 ios 应用程序检索朋友的朋友 2022-01-01
- 当从同一个组件调用时,两个 IBAction 触发的顺序是什么? 2022-01-01
- UIWebView 委托方法 shouldStartLoadWithRequest:在 WKWebView 中等效? 2022-01-01
- Kivy Buildozer 无法构建 apk,命令失败:./distribute.sh -m “kivy"d 2022-01-01
- Android:对话框关闭而不调用关闭 2022-01-01
- 如何在 iPhone 上显示来自 API 的 HTML 文本? 2022-01-01
- 如何在没有IB的情况下将2个按钮添加到右侧的UINavigationbar? 2022-01-01