How to share internal storage file with Gmail Client(如何与 Gmail 客户端共享内部存储文件)
问题描述
我正在尝试通过我的 Moto Razr 上的 Gmail 客户端共享我的内部存储文件,但每次我发送到我的测试 gmail 帐户时,我都得到了除附件之外的所有内容.
I am trying to share my internal storage file via Gmail client on my Moto Razr, but every time I sent to my test gmail account, I got everything except attachment.
这就是我调用和启动 gmail 的方式,同时将文件添加为附件.
This is how I invoke and start gmail, while add file as attachment.
private void saveDaily() {
Intent intent = new Intent(android.content.Intent.ACTION_SEND_MULTIPLE);
intent.setType("text/plain");
intent.putExtra(Intent.EXTRA_EMAIL, new String[] { loadEmailAddress() });
intent.putExtra(Intent.EXTRA_SUBJECT, "Daily");
intent.putExtra(Intent.EXTRA_TEXT, "Daily Log");
intent.addFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION);
ArrayList<Uri> uris = new ArrayList<Uri>();
uris.add(saveDaily2File("dailyRecord.txt"));
Log.d(TAG_D, "Size: " + uris.size());
intent.putParcelableArrayListExtra(Intent.EXTRA_STREAM, uris);
startActivity(Intent.createChooser(intent, "Send email"));
}
这就是我实现自定义内容提供程序的方式.
This is how I implement my customized content provider.
public class SavedFileProvider extends ContentProvider {
private static final String TAG_D = "ContentProvider";
private static final HashMap<String, String> MIME_TYPES = new HashMap<String, String>();
static {
MIME_TYPES.put(".txt", "text/plain");
}
@Override
public String getType(Uri uri) {
String path = uri.toString();
for (String extension : MIME_TYPES.keySet()) {
if (path.endsWith(extension)) {
return (MIME_TYPES.get(extension));
}
}
return (null);
}
@Override
public ParcelFileDescriptor openFile(Uri uri, String mode)
throws FileNotFoundException {
Log.d(TAG_D, "openFile()");
File f = new File(getContext().getFilesDir(), uri.getPath());
Log.d(TAG_D, f.getAbsolutePath());
if (f.exists()) {
return (ParcelFileDescriptor.open(f,
ParcelFileDescriptor.MODE_READ_ONLY));
}
throw new FileNotFoundException(uri.getPath());
}
@Override
public Cursor query(Uri url, String[] projection, String selection,
String[] selectionArgs, String sort) {
throw new RuntimeException("Operation not supported");
}
@Override
public Uri insert(Uri uri, ContentValues initialValues) {
throw new RuntimeException("Operation not supported");
}
@Override
public int update(Uri uri, ContentValues values, String where,
String[] whereArgs) {
throw new RuntimeException("Operation not supported");
}
@Override
public int delete(Uri uri, String where, String[] whereArgs) {
throw new RuntimeException("Operation not supported");
}
private void copy(InputStream in, File dst) throws IOException {
FileOutputStream out = new FileOutputStream(dst);
byte[] buf = new byte[1024];
int len;
while ((len = in.read(buf)) > 0) {
out.write(buf, 0, len);
}
in.close();
out.close();
}
@Override
public boolean onCreate() {
Log.d(TAG_D, "onCreate()");
File f = new File(getContext().getFilesDir(), "dailyRecord.txt");
if (!f.exists()) {
AssetManager assets = getContext().getResources().getAssets();
try {
copy(assets.open("dailyRecord.txt"), f);
} catch (IOException e) {
Log.e("FileProvider", "Exception copying from assets", e);
return (false);
}
}
return (true);
}
}
然后,我在我的 AndroidManifest.xml 文件中添加以下行.
Then, I add the following lines in my AndroidManifest.xml File.
<provider
android:name=".SavedFileProvider"
android:authorities="Package Path here"
android:exported="true"
android:grantUriPermissions="true"
android:multiprocess="true" >
</provider>
我想知道我在这里缺少什么.
I wonder what I am missing here.
我检查了链接:Link1, Link2
推荐答案
我用这个:
AndroidManifest.xml
AndroidManifest.xml
<provider android:name="com.myapp.main.MyContentProvider" android:authorities="com.myapp.main"></provider>
按钮点击:
public void onClick(View v) {
List<Intent> targetedShareIntents = new ArrayList<Intent>();
Intent shareIntent = new Intent(Intent.ACTION_SEND);
shareIntent.setType("image/jpg");
Uri theUri = Uri.parse("content://com.myapp.main/"+srcImage);
List<ResolveInfo> resInfo = getPackageManager().queryIntentActivities(shareIntent, 0);
int i=0;
List<ResolveInfo> reInfoToDelete = new ArrayList<ResolveInfo>();
if (!resInfo.isEmpty()){
for (ResolveInfo resolveInfo : resInfo) {
String packageName = resolveInfo.activityInfo.packageName;
Intent targetedShareIntent = new Intent(android.content.Intent.ACTION_SEND);
targetedShareIntent.setType("image/jpg");
targetedShareIntent.putExtra(android.content.Intent.EXTRA_SUBJECT, "Share file");
if (packageName.equals("com.google.android.gm")){
targetedShareIntent.setType("image/png");
targetedShareIntent.putExtra(Intent.EXTRA_TEXT, "some text");
targetedShareIntent.putExtra(Intent.EXTRA_STREAM, theUri);
targetedShareIntent.setPackage(packageName);
targetedShareIntents.add(targetedShareIntent);
}
}
startActivity(targetedShareIntents.remove(0));
}
}
我的内容提供者类
public class MyContentProvider extends ContentProvider implements PipeDataWriter<InputStream>{
@Override
public AssetFileDescriptor openAssetFile(Uri uri, String mode) throws FileNotFoundException {
//Adapt this to your code
AssetManager am = getContext().getAssets();
String file_name = "path/"+uri.getLastPathSegment();
if(file_name == null)
throw new FileNotFoundException();
AssetFileDescriptor afd = null;
try {
afd = am.openFd(file_name);
} catch (IOException e) {
e.printStackTrace();
}
return afd;//super.openAssetFile(uri, mode);
}
@Override
public int delete(Uri uri, String selection, String[] selectionArgs) {
// TODO Auto-generated method stub
return 0;
}
@Override
public String getType(Uri uri) {
// TODO Auto-generated method stub
return null;
}
@Override
public Uri insert(Uri uri, ContentValues values) {
// TODO Auto-generated method stub
return null;
}
@Override
public boolean onCreate() {
// TODO Auto-generated method stub
return false;
}
@Override
public Cursor query(Uri uri, String[] projection, String selection,
String[] selectionArgs, String sortOrder) {
// TODO Auto-generated method stub
return null;
}
@Override
public int update(Uri uri, ContentValues values, String selection,
String[] selectionArgs) {
// TODO Auto-generated method stub
return 0;
}
public void writeDataToPipe(ParcelFileDescriptor arg0, Uri arg1,
String arg2, Bundle arg3, InputStream arg4) {
// Transfer data from the asset to the pipe the client is reading.
byte[] buffer = new byte[8192];
int n;
FileOutputStream fout = new FileOutputStream(arg0.getFileDescriptor());
try {
while ((n=arg4.read(buffer)) >= 0) {
fout.write(buffer, 0, n);
}
} catch (IOException e) {
Log.i("InstallApk", "Failed transferring", e);
} finally {
try {
arg4.close();
} catch (IOException e) {
}
try {
fout.close();
} catch (IOException e) {
}
}
}
}
这篇关于如何与 Gmail 客户端共享内部存储文件的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何与 Gmail 客户端共享内部存储文件
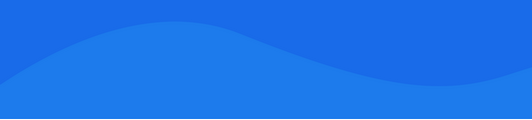
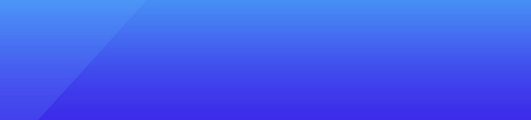
基础教程推荐
- android 应用程序已发布,但在 google play 中找不到 2022-01-01
- UIWebView 委托方法 shouldStartLoadWithRequest:在 WKWebView 中等效? 2022-01-01
- 如何在 UIImageView 中异步加载图像? 2022-01-01
- 如何在 iPhone 上显示来自 API 的 HTML 文本? 2022-01-01
- Android:对话框关闭而不调用关闭 2022-01-01
- 如何让对象对 Cocos2D 中的触摸做出反应? 2022-01-01
- Kivy Buildozer 无法构建 apk,命令失败:./distribute.sh -m “kivy"d 2022-01-01
- 在 gmail 中为 ios 应用程序检索朋友的朋友 2022-01-01
- 当从同一个组件调用时,两个 IBAction 触发的顺序是什么? 2022-01-01
- 如何在没有IB的情况下将2个按钮添加到右侧的UINavigationbar? 2022-01-01