How to use an NSAttributedString with a ScrollView in SwiftUI?(如何在 SwiftUI 中使用 NSAttributedString 和 ScrollView?)
问题描述
我已经能够通过 UIViewRepresentable
呈现 NSAttributedString
,这在我将视图包装在 ScrollView
之前效果很好.
I've been able to render NSAttributedString
s via UIViewRepresentable
which works great until I wrap the view in a ScrollView
.
当放置在 ScrollView
内时,NSAttributedString
视图会停止渲染.
When placed inside the ScrollView
, the NSAttributedString
view stops rendering.
我尝试了一些其他方法来替换 NSAttributedString
并添加多个 Text()
视图以获得在 ScrollView
并支持斜体和等宽字体
.不幸的是,这对于文本块内的 links 不起作用,这意味着我仍然需要一个 NSAttributedString
.
I've tried some other methods that replace an NSAttributedString
with adding multiple Text()
views together to get formatting which works inside the ScrollView
and supports italics and monospace font
. Unfortunately this doesn't work for links inside text blocks, which means I still need an NSAttributedString
.
import SwiftUI
struct TextWithAttributedString: UIViewRepresentable {
var attributedString: NSAttributedString
init(_ attributedString: NSAttributedString) {
self.attributedString = attributedString
}
func makeUIView(context: Context) -> UITextView {
let textView = UITextView(frame: .zero)
textView.attributedText = self.attributedString
textView.isEditable = false
return textView
}
func updateUIView(_ textView: UITextView, context: Context) {
textView.attributedText = self.attributedString
}
}
let exampleText = """
Fugiat id blanditiis et est culpa voluptas. Vivamus aliquet enim eu blandit blandit. Sit eget praesentium maxime sit molestiae et alias aut.
"""
struct NSAttributedStringView: View {
var body: some View {
// Note: when uncommented, the view breaks
// ScrollView {
TextWithAttributedString(NSAttributedString(string: exampleText))
// }
}
}
struct NSAttributedStringView_Previews: PreviewProvider {
static var previews: some View {
NSAttributedStringView()
.previewLayout(.sizeThatFits)
}
}
我尝试使用包装的 UITextView
并设置 text
属性而不是 attributeText
属性,但这也无法在 ScrollView
中呈现,所以问题似乎是 UITextView
,而不是 NSAttributedString
.
I tried using the wrapped UITextView
with the text
property set instead of the attributeText
property, but this also fails to render in the ScrollView
, so the issue seems to be the UITextView
, not the NSAttributedString
.
所以问题是,我们如何让 UITextView
在 ScrollView
中工作?
So the question is, how do we get the UITextView
to work in a ScrollView
?
推荐答案
原因是SwiftUI ScrollView
需要定义内容大小,但是使用的UITextView
本身就是一个UIScrollView
并根据父视图中的可用空间检测内容.因此,它发生了未定义大小的循环.
The reason is that SwiftUI ScrollView
requires defined content size, but used UITextView
is itself a UIScrollView
and detects content based on available space in parent view. Thus it happens cycle of undefined sizes.
以下是解决此问题的可能方法的简化演示.这个想法是计算 UITextView
的内容大小并将其传递给 SwiftUI...
Here is a simplified demo of possible approach how to solve this. The idea is to calculate content size of UITextView
and pass it to SwiftUI...
struct TextWithAttributedString: UIViewRepresentable {
@Binding var height: CGFloat
var attributedString: NSAttributedString
func makeUIView(context: Context) -> UITextView {
let textView = UITextView(frame: .zero)
textView.isEditable = false
return textView
}
func updateUIView(_ textView: UITextView, context: Context) {
textView.attributedText = self.attributedString
// calculate height based on main screen, but this might be
// improved for more generic cases
DispatchQueue.main.async { // << fixed
height = textView.sizeThatFits(UIScreen.main.bounds.size).height
}
}
}
struct NSAttributedStringView: View {
@State private var textHeight: CGFloat = .zero
var body: some View {
ScrollView {
TextWithAttributedString(height: $textHeight, attributedString: NSAttributedString(string: exampleText))
.frame(height: textHeight) // << specify height explicitly !
}
}
}
这篇关于如何在 SwiftUI 中使用 NSAttributedString 和 ScrollView?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何在 SwiftUI 中使用 NSAttributedString 和 ScrollView?
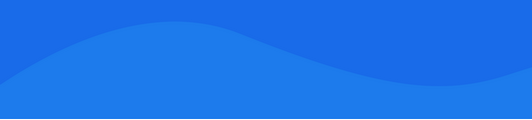
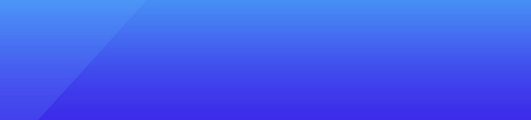
基础教程推荐
- 当从同一个组件调用时,两个 IBAction 触发的顺序是什么? 2022-01-01
- Android:对话框关闭而不调用关闭 2022-01-01
- 如何在 iPhone 上显示来自 API 的 HTML 文本? 2022-01-01
- 如何在 UIImageView 中异步加载图像? 2022-01-01
- android 应用程序已发布,但在 google play 中找不到 2022-01-01
- 在 gmail 中为 ios 应用程序检索朋友的朋友 2022-01-01
- 如何在没有IB的情况下将2个按钮添加到右侧的UINavigationbar? 2022-01-01
- 如何让对象对 Cocos2D 中的触摸做出反应? 2022-01-01
- UIWebView 委托方法 shouldStartLoadWithRequest:在 WKWebView 中等效? 2022-01-01
- Kivy Buildozer 无法构建 apk,命令失败:./distribute.sh -m “kivy"d 2022-01-01