Synchronized Array (for likes/followers) Best Practice [Firebase Swift](同步数组(针对喜欢/关注者)最佳实践 [Firebase Swift])
问题描述
我正在尝试使用 Swift 和 Firebase 创建一个基本的跟踪算法.我目前的实现如下:
static func follow(user: FIRUser, userToFollow: FIRUser) {database.child("users").child(user.uid).observeSingleEventOfType(.Value, withBlock: { (snapshot) invar dbFollowing:NSMutableArray!= snapshot.value!["Following"] as!NSMutableArray!dbFollowing?.addObject(userToFollow.uid)self.database.child("users/"+(user.uid)+"/").updateChildValues(["Following":dbFollowing!])//以类似的方式将用户uid添加到userToFollows关注者数组}) {(错误)在print("follow - 无法检索数据 - 例外:" + error.localizedDescription)}}
这会从 Firebase 节点 Following
检索数组,添加 userToFollow
的 uid,并将新数组发布到节点 Following
.这有几个问题:
- 它是不同步的,因此如果在两个设备上同时调用它,一个数组将覆盖另一个数组,并且不会保存跟随者.
- 如果没有追随者,它就无法处理 nil 数组,程序将崩溃(不是主要问题,我可能可以通过选项来解决).
我想知道为用户关注者或帖子点赞创建一个同步的 uid/tokens 数组的最佳做法是什么.我找到了以下链接,但似乎没有一个可以直接解决我的问题并且似乎还存在其他问题.我认为向有经验的社区询问是明智的做法,而不是把一堆解决方案一起弗兰肯斯坦.
<块引用>https://firebase.googleblog.com/2014/05/handling-synchronized-arrays-with-real.htmlhttps://firebase.google.com/docs/database/ios/save-data(将数据保存为事务部分)
感谢您的帮助!
感谢 Frank,我找到了一个使用 runTransactionBlock
的解决方案.这里是:
static func follow(user: FIRUser, userToFollow: FIRUser) {self.database.child("users/"+(user.uid)+"/Following").runTransactionBlock({ (currentData: FIRMutableData!) -> FIRTransactionResult invar value = currentData?.value as?数组<字符串>如果(值 == 零){值 = [userToFollow.uid]} 别的 {如果!(值!.contains(userToFollow.uid)){价值!.append(userToFollow.uid)}}currentData.value = 值!返回 FIRTransactionResult.successWithValue(currentData)}) {(错误,提交,快照)在如果让错误=错误{print("follow - 更新后续事务 - 例外:" + error.localizedDescription)}}}
这会将 userToFollow
的 uid 添加到 user
的数组 Following
中.它可以处理 nil 值并相应地进行初始化,并且如果用户已经关注 userToFollow
的 uid,它将忽略请求.如果您有任何问题,请告诉我!
一些有用的链接:
<块引用>- firebase runTransactionBlock 的评论
- 通过 Firebase 在 Swift 中支持/反对系统的答案
- 我在上面发布的第二个链接
I'm trying to create a basic following algorithm using Swift and Firebase. My current implementation is the following:
static func follow(user: FIRUser, userToFollow: FIRUser) {
database.child("users").child(user.uid).observeSingleEventOfType(.Value, withBlock: { (snapshot) in
var dbFollowing: NSMutableArray! = snapshot.value!["Following"] as! NSMutableArray!
dbFollowing?.addObject(userToFollow.uid)
self.database.child("users/"+(user.uid)+"/").updateChildValues(["Following":dbFollowing!])
//add user uid to userToFollows followers array in similar way
}) { (error) in
print("follow - data could not be retrieved - EXCEPTION: " + error.localizedDescription)
}
}
This retrieves the array of from Firebase node Following
, adds the uid of userToFollow
, and posts the new array to node Following
. This has a few problems:
- It is not synchronized so if it is called at the same time on two devices one array will overwrite the other and followers will not be saved.
- If there are no followers it cannot deal with a nil array, the program will crash (not the main concern, I can probably address with optionals).
I was wondering what the best practice might be to created a synchronized array of uid/tokens for user followers or post likes. I found the following links, but none seem to directly address my problem and seem to carry other problems with it. I figured it would be wise to ask the community with experience instead of Frankensteining a bunch of solutions together.
https://firebase.googleblog.com/2014/05/handling-synchronized-arrays-with-real.html https://firebase.google.com/docs/database/ios/save-data (the save data as transaction section)
Thanks for your help!
Thanks to Frank, I figured out a solution using runTransactionBlock
. Here it is:
static func follow(user: FIRUser, userToFollow: FIRUser) {
self.database.child("users/"+(user.uid)+"/Following").runTransactionBlock({ (currentData: FIRMutableData!) -> FIRTransactionResult in
var value = currentData?.value as? Array<String>
if (value == nil) {
value = [userToFollow.uid]
} else {
if !(value!.contains(userToFollow.uid)) {
value!.append(userToFollow.uid)
}
}
currentData.value = value!
return FIRTransactionResult.successWithValue(currentData)
}) { (error, committed, snapshot) in
if let error = error {
print("follow - update following transaction - EXCEPTION: " + error.localizedDescription)
}
}
}
This adds the uid of userToFollow
to the array Following
of user
. It can handle nil values and will initialize accordingly, as well as will disregard the request if the user is already following the uid of userToFollow
. Let me know if you have any questions!
Some useful links:
- The comments of firebase runTransactionBlock
- The answer to Upvote/Downvote system within Swift via Firebase
- The second link I posted above
这篇关于同步数组(针对喜欢/关注者)最佳实践 [Firebase Swift]的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:同步数组(针对喜欢/关注者)最佳实践 [Firebase Swift]
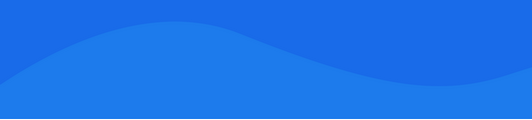
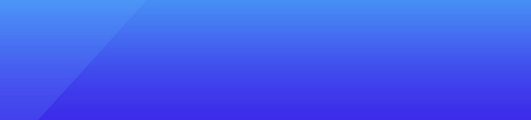
基础教程推荐
- Kivy Buildozer 无法构建 apk,命令失败:./distribute.sh -m “kivy"d 2022-01-01
- 如何在 iPhone 上显示来自 API 的 HTML 文本? 2022-01-01
- 如何在没有IB的情况下将2个按钮添加到右侧的UINavigationbar? 2022-01-01
- 当从同一个组件调用时,两个 IBAction 触发的顺序是什么? 2022-01-01
- android 应用程序已发布,但在 google play 中找不到 2022-01-01
- UIWebView 委托方法 shouldStartLoadWithRequest:在 WKWebView 中等效? 2022-01-01
- 如何让对象对 Cocos2D 中的触摸做出反应? 2022-01-01
- Android:对话框关闭而不调用关闭 2022-01-01
- 如何在 UIImageView 中异步加载图像? 2022-01-01
- 在 gmail 中为 ios 应用程序检索朋友的朋友 2022-01-01