Converting Text to Image on iOS(在 iOS 上将文本转换为图像)
问题描述
如何将文本转换为图像并在 UIImageview 中显示.有谁知道从文本到图像的转换?
How to convert Text to Image and show in UIImageview. Can anyone know the conversion from Text to Image?
推荐答案
使用 Swift 5 和 iOS 12,您可以选择以下6种方式中的一种来解决您的问题.
With Swift 5 and iOS 12, you can choose one the 6 following ways in order to solve your problem.
在您想将 String
转换为具有某些属性的 UIImage
的最简单情况下,您可以使用 draw(at:withAttributes:)
.以下 Playground 代码展示了如何使用 draw(at:withAttributes:)
从 String
获取 UIImage
:
In the simplest case where you want to convert a String
to a UIImage
with some attributes, you can use draw(at:withAttributes:)
. The following Playground codes show how to get an UIImage
from a String
using draw(at:withAttributes:)
:
import UIKit
import PlaygroundSupport
let text = "Hello, world"
let attributes = [
NSAttributedString.Key.foregroundColor: UIColor.yellow,
NSAttributedString.Key.font: UIFont.systemFont(ofSize: 22)
]
let textSize = text.size(withAttributes: attributes)
UIGraphicsBeginImageContextWithOptions(textSize, true, 0)
text.draw(at: CGPoint.zero, withAttributes: attributes)
let image = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
PlaygroundPage.current.liveView = UIImageView(image: image)
import UIKit
import PlaygroundSupport
let text = "Hello, world"
let attributes = [
NSAttributedString.Key.foregroundColor: UIColor.yellow,
NSAttributedString.Key.font: UIFont.systemFont(ofSize: 22)
]
let textSize = text.size(withAttributes: attributes)
let renderer = UIGraphicsImageRenderer(size: textSize)
let image = renderer.image(actions: { context in
text.draw(at: CGPoint.zero, withAttributes: attributes)
})
PlaygroundPage.current.liveView = UIImageView(image: image)
请注意,NSAttributedString
有一个类似的方法,称为 draw(at:)
.
Note that NSAttributedString
has a similar method called draw(at:)
.
作为 draw(at:withAttributes:)
的替代方法,您可以使用 draw(in:withAttributes:)
.
As an alternative to draw(at:withAttributes:)
, you can use draw(in:withAttributes:)
.
import UIKit
import PlaygroundSupport
let text = "Hello, world"
let attributes = [
NSAttributedString.Key.foregroundColor: UIColor.yellow,
NSAttributedString.Key.font: UIFont.systemFont(ofSize: 22)
]
let textSize = text.size(withAttributes: attributes)
UIGraphicsBeginImageContextWithOptions(textSize, true, 0)
let rect = CGRect(origin: .zero, size: textSize)
text.draw(in: rect, withAttributes: attributes)
let image = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
PlaygroundPage.current.liveView = UIImageView(image: image)
import UIKit
import PlaygroundSupport
let text = "Hello, world"
let attributes = [
NSAttributedString.Key.foregroundColor: UIColor.yellow,
NSAttributedString.Key.font: UIFont.systemFont(ofSize: 22)
]
let textSize = text.size(withAttributes: attributes)
let renderer = UIGraphicsImageRenderer(size: textSize)
let image = renderer.image(actions: { context in
let rect = CGRect(origin: .zero, size: textSize)
text.draw(in: rect, withAttributes: attributes)
})
PlaygroundPage.current.liveView = UIImageView(image: image)
请注意,NSAttributedString
有一个类似的方法,称为 draw(in:)
.
Note that NSAttributedString
has a similar method called draw(in:)
.
作为 draw(at:withAttributes:)
和 draw(in:)
的替代方法,您可以使用 draw(with:options:attributes:context:)
.请注意,Apple 对 draw(with:options:attributes:context:)
:
As an alternative to draw(at:withAttributes:)
and draw(in:)
, you can use draw(with:options:attributes:context:)
. Note that Apple has some recommendations for draw(with:options:attributes:context:)
:
此方法默认使用基线原点.如果没有指定usesLineFragmentOrigin
,矩形的高度将被忽略,该操作被认为是单线渲染.
This method uses the baseline origin by default. If
usesLineFragmentOrigin
is not specified, the rectangle’s height will be ignored and the operation considered to be single-line rendering.
import UIKit
import PlaygroundSupport
let text = "Hello, world"
let attributes = [
NSAttributedString.Key.foregroundColor: UIColor.yellow,
NSAttributedString.Key.font: UIFont.systemFont(ofSize: 22)
]
let textSize = text.size(withAttributes: attributes)
UIGraphicsBeginImageContextWithOptions(textSize, true, 0)
let rect = CGRect(origin: .zero, size: textSize)
text.draw(with: rect, options: [.usesLineFragmentOrigin], attributes: attributes, context: nil)
let image = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
PlaygroundPage.current.liveView = UIImageView(image: image)
import UIKit
import PlaygroundSupport
let text = "Hello, world"
let attributes = [
NSAttributedString.Key.foregroundColor: UIColor.yellow,
NSAttributedString.Key.font: UIFont.systemFont(ofSize: 22)
]
let textSize = text.size(withAttributes: attributes)
let renderer = UIGraphicsImageRenderer(size: textSize)
let image = renderer.image(actions: { context in
text.draw(with: .zero, options: [.usesLineFragmentOrigin], attributes: attributes, context: nil)
})
PlaygroundPage.current.liveView = UIImageView(image: image)
请注意,NSAttributedString
有一个类似的方法,称为 draw(with:options:context:)
.
Note that NSAttributedString
has a similar method called draw(with:options:context:)
.
如果您想将 UILabel
、UITextField
或 UITextView
的文本捕获到 UIImage
,您可以使用 render(in:)
.以下 Playground 代码展示了如何使用 render(in:)
对 UILabel
的内容文本进行快照:
If you want to capture the text of a UILabel
, UITextField
or UITextView
to a UIImage
, you can use render(in:)
. The following Playground codes show how to snapshot the content text of a UILabel
using render(in:)
:
import UIKit
import PlaygroundSupport
let label = UILabel(frame: .zero)
label.textColor = .yellow
label.font = UIFont.systemFont(ofSize: 22)
label.text = "Hello, world"
label.sizeToFit()
UIGraphicsBeginImageContextWithOptions(label.frame.size, true, 0)
guard let context = UIGraphicsGetCurrentContext() else { exit(0) }
label.layer.render(in: context)
let image = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
PlaygroundPage.current.liveView = UIImageView(image: image)
import UIKit
import PlaygroundSupport
let label = UILabel(frame: .zero)
label.textColor = .yellow
label.font = UIFont.systemFont(ofSize: 22)
label.text = "Hello, world"
label.sizeToFit()
let renderer = UIGraphicsImageRenderer(size: label.frame.size)
let image = renderer.image(actions: { context in
label.layer.render(in: context.cgContext)
})
PlaygroundPage.current.liveView = UIImageView(image: image)
<小时>
#5.使用 UIView
的 drawHierarchy(in:afterScreenUpdates:)
方法
如果您想将 UILabel
、UITextField
或 UITextView
的文本捕获到 UIImage
,您可以使用 drawHierarchy(in:afterScreenUpdates:)
.请注意,Apple 对 drawHierarchy(in:afterScreenUpdates:)
有一些建议:
#5. Using UIView
's drawHierarchy(in:afterScreenUpdates:)
method
If you want to capture the text of a UILabel
, UITextField
or UITextView
to a UIImage
, you can use drawHierarchy(in:afterScreenUpdates:)
. Note that Apple has some recommendations for drawHierarchy(in:afterScreenUpdates:)
:
当您想要将图形效果(例如模糊)应用到视图快照时,请使用此方法.此方法不如 snapshotView(afterScreenUpdates:)
方法快.
Use this method when you want to apply a graphical effect, such as a blur, to a view snapshot. This method is not as fast as the
snapshotView(afterScreenUpdates:)
method.
import UIKit
import PlaygroundSupport
let label = UILabel(frame: .zero)
label.textColor = .yellow
label.font = UIFont.systemFont(ofSize: 22)
label.text = "Hello, world"
label.sizeToFit()
UIGraphicsBeginImageContextWithOptions(label.frame.size, true, 0)
_ = label.drawHierarchy(in: label.bounds, afterScreenUpdates: true)
let image = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
PlaygroundPage.current.liveView = UIImageView(image: image)
import UIKit
import PlaygroundSupport
let label = UILabel(frame: .zero)
label.textColor = .yellow
label.font = UIFont.systemFont(ofSize: 22)
label.text = "Hello, world"
label.sizeToFit()
let renderer = UIGraphicsImageRenderer(size: label.frame.size)
let image = renderer.image(actions: { context in
_ = label.drawHierarchy(in: label.bounds, afterScreenUpdates: true)
})
PlaygroundPage.current.liveView = UIImageView(image: image)
<小时>
#6.使用 UIView
的 snapshotView(afterScreenUpdates:)
方法
如果您可以从快照操作中获取 UIView
而不是 UIImage
,则可以使用 snapshotView(afterScreenUpdates:)
.以下 Playground 代码展示了如何使用 snapshotView(afterScreenUpdates:)
将 UILabel
的内容文本快照到 UIView
中:
#6. Using UIView
's snapshotView(afterScreenUpdates:)
method
If it's OK for you to get a UIView
instead of a UIImage
from your snapshot operation, you can use snapshotView(afterScreenUpdates:)
. The following Playground code shows how to snapshot the content text of a UILabel
into a UIView
using snapshotView(afterScreenUpdates:)
:
import UIKit
import PlaygroundSupport
let label = UILabel(frame: .zero)
label.textColor = .yellow
label.font = UIFont.systemFont(ofSize: 22)
label.text = "Hello, world"
label.sizeToFit()
let view = label.snapshotView(afterScreenUpdates: true)
PlaygroundPage.current.liveView = view
这篇关于在 iOS 上将文本转换为图像的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:在 iOS 上将文本转换为图像
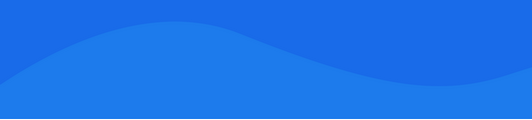
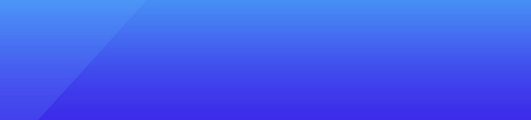
基础教程推荐
- 如何在 UIImageView 中异步加载图像? 2022-01-01
- 如何让对象对 Cocos2D 中的触摸做出反应? 2022-01-01
- 如何在没有IB的情况下将2个按钮添加到右侧的UINavigationbar? 2022-01-01
- UIWebView 委托方法 shouldStartLoadWithRequest:在 WKWebView 中等效? 2022-01-01
- Android:对话框关闭而不调用关闭 2022-01-01
- 当从同一个组件调用时,两个 IBAction 触发的顺序是什么? 2022-01-01
- android 应用程序已发布,但在 google play 中找不到 2022-01-01
- 在 gmail 中为 ios 应用程序检索朋友的朋友 2022-01-01
- Kivy Buildozer 无法构建 apk,命令失败:./distribute.sh -m “kivy"d 2022-01-01
- 如何在 iPhone 上显示来自 API 的 HTML 文本? 2022-01-01