Swift: what is the right way to split up a [String] resulting in a [[String]] with a given subarray size?(Swift:拆分 [String] 得到具有给定子数组大小的 [[String]] 的正确方法是什么?)
问题描述
从一个大的 [String] 和给定的子数组大小开始,我可以将这个数组拆分为更小的数组的最佳方法是什么?(最后一个数组将小于给定的子数组大小).
Starting with a large [String] and a given subarray size, what is the best way I could go about splitting up this array into smaller arrays? (The last array will be smaller than the given subarray size).
具体例子:
使用最大拆分大小 2 拆分 [1"、2"、3"、4"、5"、6"、7"]
Split up ["1","2","3","4","5","6","7"] with max split size 2
代码将产生 [[1"、2"]、[3"、4"]、[5"、6"]、[7"]]
The code would produce [["1","2"],["3","4"],["5","6"],["7"]]
显然我可以更手动地执行此操作,但我觉得像 map() 或 reduce() 这样的快速操作可能会非常漂亮地完成我想要的操作.
Obviously I could do this a little more manually, but I feel like in swift something like map() or reduce() may do what I want really beautifully.
推荐答案
我不会说它漂亮,但这里有一个使用 map
的方法:
I wouldn't call it beautiful, but here's a method using map
:
let numbers = ["1","2","3","4","5","6","7"]
let splitSize = 2
let chunks = numbers.startIndex.stride(to: numbers.count, by: splitSize).map {
numbers[$0 ..< $0.advancedBy(splitSize, limit: numbers.endIndex)]
}
stride(to:by:)
方法为您提供每个块的第一个元素的索引,因此您可以使用 advancedBy(距离:限制:)
.
The stride(to:by:)
method gives you the indices for the first element of each chunk, so you can map those indices to a slice of the source array using advancedBy(distance:limit:)
.
一种更功能性"的方法就是对数组进行递归,如下所示:
A more "functional" approach would simply be to recurse over the array, like so:
func chunkArray<T>(s: [T], splitSize: Int) -> [[T]] {
if countElements(s) <= splitSize {
return [s]
} else {
return [Array<T>(s[0..<splitSize])] + chunkArray(Array<T>(s[splitSize..<s.count]), splitSize)
}
}
这篇关于Swift:拆分 [String] 得到具有给定子数组大小的 [[String]] 的正确方法是什么?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:Swift:拆分 [String] 得到具有给定子数组大小的 [[String]] 的正确方法是什么?
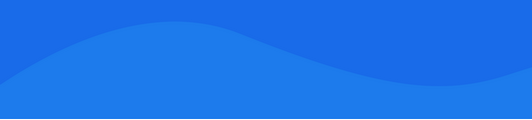
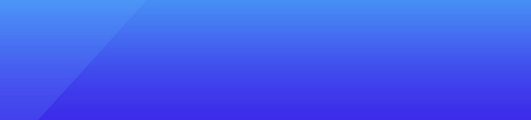
基础教程推荐
- 如何让对象对 Cocos2D 中的触摸做出反应? 2022-01-01
- UIWebView 委托方法 shouldStartLoadWithRequest:在 WKWebView 中等效? 2022-01-01
- 如何在 iPhone 上显示来自 API 的 HTML 文本? 2022-01-01
- android 应用程序已发布,但在 google play 中找不到 2022-01-01
- Android:对话框关闭而不调用关闭 2022-01-01
- 如何在没有IB的情况下将2个按钮添加到右侧的UINavigationbar? 2022-01-01
- 如何在 UIImageView 中异步加载图像? 2022-01-01
- Kivy Buildozer 无法构建 apk,命令失败:./distribute.sh -m “kivy"d 2022-01-01
- 当从同一个组件调用时,两个 IBAction 触发的顺序是什么? 2022-01-01
- 在 gmail 中为 ios 应用程序检索朋友的朋友 2022-01-01