How do I use DrawerLayout to display over the ActionBar/Toolbar and under the status bar?(如何使用 DrawerLayout 在 ActionBar/Toolbar 上方和状态栏下方显示?)
问题描述
I've seen in the new material design Side Nav spec that you can display the drawer over the action bar and behind the status bar. How can I implement this?
New functionality in the framework and support libs allow exactly this. There are three 'pieces of the puzzle':
- Using Toolbar so that you can embed your action bar into your view hierarchy.
- Making DrawerLayout
fitsSystemWindows
so that it is layed out behind the system bars. - Disabling
Theme.Material
's normal status bar coloring so that DrawerLayout can draw there instead.
I'll assume that you will use the new appcompat.
First, your layout should look like this:
<!-- The important thing to note here is the added fitSystemWindows -->
<android.support.v4.widget.DrawerLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/my_drawer_layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:fitsSystemWindows="true">
<!-- Your normal content view -->
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<!-- We use a Toolbar so that our drawer can be displayed
in front of the action bar -->
<android.support.v7.widget.Toolbar
android:id="@+id/my_awesome_toolbar"
android:layout_height="wrap_content"
android:layout_width="match_parent"
android:minHeight="?attr/actionBarSize"
android:background="?attr/colorPrimary" />
<!-- The rest of your content view -->
</LinearLayout>
<!-- Your drawer view. This can be any view, LinearLayout
is just an example. As we have set fitSystemWindows=true
this will be displayed under the status bar. -->
<LinearLayout
android:layout_width="304dp"
android:layout_height="match_parent"
android:layout_gravity="left|start"
android:fitsSystemWindows="true">
<!-- Your drawer content -->
</LinearLayout>
</android.support.v4.widget.DrawerLayout>
Then in your Activity/Fragment:
public void onCreate(Bundled savedInstanceState) {
super.onCreate(savedInstanceState);
// Your normal setup. Blah blah ...
// As we're using a Toolbar, we should retrieve it and set it
// to be our ActionBar
Toolbar toolbar = (...) findViewById(R.id.my_awesome_toolbar);
setSupportActionBar(toolbar);
// Now retrieve the DrawerLayout so that we can set the status bar color.
// This only takes effect on Lollipop, or when using translucentStatusBar
// on KitKat.
DrawerLayout drawerLayout = (...) findViewById(R.id.my_drawer_layout);
drawerLayout.setStatusBarBackgroundColor(yourChosenColor);
}
Then you need to make sure that the DrawerLayout is visible behind the status bar. You do that by changing your values-v21 theme:
values-v21/themes.xml
<style name="Theme.MyApp" parent="Theme.AppCompat.Light.NoActionBar">
<item name="android:windowDrawsSystemBarBackgrounds">true</item>
<item name="android:statusBarColor">@android:color/transparent</item>
<item name="android:windowTranslucentStatus">true</item>
</style>
Note:
If a <fragment android:name="fragments.NavigationDrawerFragment">
is used instead of
<LinearLayout
android:layout_width="304dp"
android:layout_height="match_parent"
android:layout_gravity="left|start"
android:fitsSystemWindows="true">
<!-- Your drawer content -->
</LinearLayout>
the actual layout, the desired effect will be achieved if you call fitsSystemWindows(boolean)
on a view that you return from onCreateView
method.
@Override
public View onCreateView(LayoutInflater inflater,
ViewGroup container,
Bundle savedInstanceState) {
View mDrawerListView = inflater.inflate(
R.layout.fragment_navigation_drawer, container, false);
mDrawerListView.setFitsSystemWindows(true);
return mDrawerListView;
}
这篇关于如何使用 DrawerLayout 在 ActionBar/Toolbar 上方和状态栏下方显示?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何使用 DrawerLayout 在 ActionBar/Toolbar 上方和状态栏下方显示?
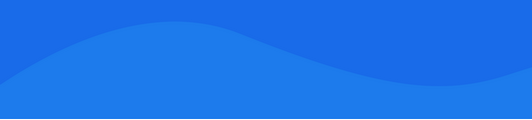
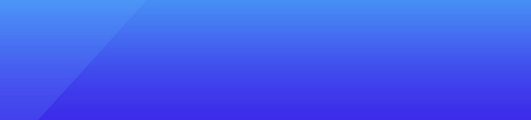
基础教程推荐
- 如何在 iPhone 上显示来自 API 的 HTML 文本? 2022-01-01
- 如何让对象对 Cocos2D 中的触摸做出反应? 2022-01-01
- 当从同一个组件调用时,两个 IBAction 触发的顺序是什么? 2022-01-01
- android 应用程序已发布,但在 google play 中找不到 2022-01-01
- 如何在没有IB的情况下将2个按钮添加到右侧的UINavigationbar? 2022-01-01
- Kivy Buildozer 无法构建 apk,命令失败:./distribute.sh -m “kivy"d 2022-01-01
- UIWebView 委托方法 shouldStartLoadWithRequest:在 WKWebView 中等效? 2022-01-01
- Android:对话框关闭而不调用关闭 2022-01-01
- 在 gmail 中为 ios 应用程序检索朋友的朋友 2022-01-01
- 如何在 UIImageView 中异步加载图像? 2022-01-01