How To preload AdMob interstitial ad and send to another android activity using intent(如何使用 Intent 预加载 AdMob 插页式广告并发送到另一个 android 活动)
问题描述
I need some help regarding AdMob interstitial ad.
I want to preload the interstitial ad in one activity. this is straight forward.
// Create an ad.
interstitialAd = new InterstitialAd(this);
interstitialAd.setAdUnitId(AD_UNIT_ID);
AdRequest adRequest = new AdRequest.Builder()
.addTestDevice(AdRequest.DEVICE_ID_EMULATOR)
.addTestDevice(TEST_DEVICE_ID).build();
// Load the interstitial ad.
interstitialAd.loadAd(adRequest);
Now I want to send the interstitial Ad to another activity using intent. I don't know how to send it using
intent.putExtra("myAd", interstitialAd);
Thanks in advance.
Interstitial ads are not meant or built to be passed around like that using intents' extras.
It's better to
- recreate & reload an ad in the next activity
- make an extra public class that holds the interstitial ad, put it there in activity A and retrieve it from there in activity B
Example for 2nd case (semi pseudo code):
public class AdManager {
// Static fields are shared between all instances.
static InterstitialAd ad;
private Context ctx;
public AdManager(Context ctx) {
this.ctx = ctx;
createAd();
}
public void createAd() {
// Create an ad.
ad = new InterstitialAd(ctx);
ad.setAdUnitId(AD_UNIT_ID);
final AdRequest adRequest = new AdRequest.Builder()
.addTestDevice(AdRequest.DEVICE_ID_EMULATOR)
.addTestDevice(TEST_DEVICE_ID).build();
// Load the interstitial ad.
ad.loadAd(adRequest);
}
public InterstitialAd getAd() {
return ad;
}
}
Using
Activity A
AdManager adManager = new AdManager();
adManager.createAd();
Activity B
AdManager adManager = new AdManager();
InterstitialAd ad = adManager.getAd();
if (ad.isLoaded()) {
ad.show();
}
这篇关于如何使用 Intent 预加载 AdMob 插页式广告并发送到另一个 android 活动的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何使用 Intent 预加载 AdMob 插页式广告并发送到另一个 android 活动
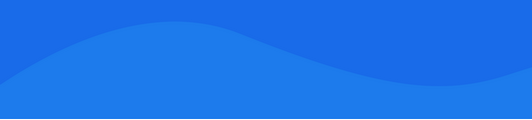
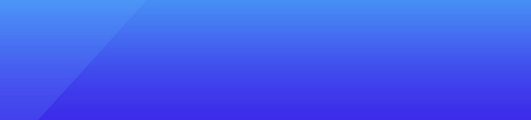
基础教程推荐
- android 应用程序已发布,但在 google play 中找不到 2022-01-01
- 如何让对象对 Cocos2D 中的触摸做出反应? 2022-01-01
- UIWebView 委托方法 shouldStartLoadWithRequest:在 WKWebView 中等效? 2022-01-01
- 当从同一个组件调用时,两个 IBAction 触发的顺序是什么? 2022-01-01
- Kivy Buildozer 无法构建 apk,命令失败:./distribute.sh -m “kivy"d 2022-01-01
- Android:对话框关闭而不调用关闭 2022-01-01
- 如何在 iPhone 上显示来自 API 的 HTML 文本? 2022-01-01
- 如何在没有IB的情况下将2个按钮添加到右侧的UINavigationbar? 2022-01-01
- 在 gmail 中为 ios 应用程序检索朋友的朋友 2022-01-01
- 如何在 UIImageView 中异步加载图像? 2022-01-01