How to ensure make sure I#180;m not accessing data until it#180;s loaded in?(如何确保在加载数据之前我不会访问数据?)
问题描述
I´m new at programming and I my code gets the error: fatal error: unexpectedly found nil while unwrapping an Optional value 2017-10-27 16:06:16.755817+0200 Inspireme1.0[836:85307] fatal error: unexpectedly found nil while unwrapping an Optional value (lldb)
New error: fatal error: Index out of range 2017-10-27 19:08:05.488502+0200 Inspireme1.0[1262:771572] fatal error: Index out of range (lldb)
I already looked it up here but I don´t know how to apply this in my case: How to ensure I'm not accessing outlets before they're loaded in
Here is my code:
var quotes: RandomItems! = RandomItems([
"Jonas",
"Mary",
"Michael",
"Jeff",
"Sarah",
])
@IBAction func PresentText(_ sender: Any) {
PresentingLabel.text = quotes.next() //<-- Error
}
struct RandomItems: Codable
{
var items : [String]
var seen = 0
init(items:[String], seen: Int)
{
self.items = items
self.seen = seen
}
init(_ items:[String])
{ self.init(items: items, seen: 0) }
mutating func next() -> String
{
let index = Int(arc4random_uniform(UInt32(items.count - seen)))
let item = items.remove(at:index) //<--Error
items.append(item)
seen = (seen + 1) % items.count
return item
}
func toPropertyList() -> [String: Any] {
return [
"items": items,
"seen": seen
]
}
}
var randomItems: RandomItems?
override func viewDidAppear(_ animated: Bool) {
// Code to load the struct again after the view appears.
let defaults = UserDefaults.standard
quotes = defaults.codable(RandomItems.self, forKey: "quotes")
}
override func viewWillDisappear(_ animated: Bool) {
// Code to save struct before the view disappears.
let defaults = UserDefaults.standard
if let quotes = quotes {
defaults.set(codable: quotes, forKey: "quotes")
}
}
}
Quotes should not be optional.
You need to handle the optional outcome in viewDidAppear rather than assuming quotes will always unwrap.
quotes = defaults.codable(RandomItems.self, forKey: "quotes") ?? RandomItems([])
In viewDidLoad:
defaults.set(codable: quotes, forKey: "quotes")
这篇关于如何确保在加载数据之前我不会访问数据?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何确保在加载数据之前我不会访问数据?
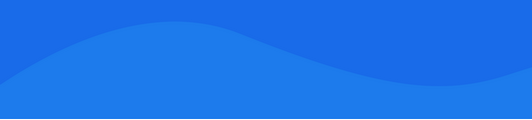
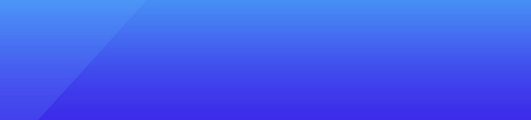
基础教程推荐
- 如何在 iPhone 上显示来自 API 的 HTML 文本? 2022-01-01
- android 应用程序已发布,但在 google play 中找不到 2022-01-01
- UIWebView 委托方法 shouldStartLoadWithRequest:在 WKWebView 中等效? 2022-01-01
- 如何让对象对 Cocos2D 中的触摸做出反应? 2022-01-01
- 在 gmail 中为 ios 应用程序检索朋友的朋友 2022-01-01
- Kivy Buildozer 无法构建 apk,命令失败:./distribute.sh -m “kivy"d 2022-01-01
- 当从同一个组件调用时,两个 IBAction 触发的顺序是什么? 2022-01-01
- Android:对话框关闭而不调用关闭 2022-01-01
- 如何在没有IB的情况下将2个按钮添加到右侧的UINavigationbar? 2022-01-01
- 如何在 UIImageView 中异步加载图像? 2022-01-01