Laravel Model with Two Primary Keys update(带有两个主键的 Laravel 模型更新)
问题描述
我正在尝试更新具有两个主键的模型.
I'm trying to update Model which has two primary keys.
型号
namespace App;
use IlluminateDatabaseEloquentModel;
class Inventory extends Model
{
/**
* The table associated with the model.
*/
protected $table = 'inventories';
/**
* Indicates model primary keys.
*/
protected $primaryKey = ['user_id', 'stock_id'];
...
迁移
Schema::create('inventories', function (Blueprint $table) {
$table->integer('user_id')->unsigned();
$table->integer('stock_id')->unsigned();
$table->bigInteger('quantity');
$table->primary(['user_id', 'stock_id']);
$table->foreign('user_id')->references('id')->on('users')
->onUpdate('restrict')
->onDelete('cascade');
$table->foreign('stock_id')->references('id')->on('stocks')
->onUpdate('restrict')
->onDelete('cascade');
});
这是应该更新库存模型的代码,但它没有.
This is code which should update Inventory model, but it doesn't.
$inventory = Inventory::where('user_id', $user->id)->where('stock_id', $order->stock->id)->first();
$inventory->quantity += $order->quantity;
$inventory->save();
我收到此错误:
Illegal offset type
我也尝试使用 updateOrCreate() 方法.它不起作用(我得到同样的错误).
I also tried to use updateOrCreate() method. It doesn't work (I get same error).
谁能告诉我们应该如何更新具有两个主键的模型?
Can anyone tell how Model with two primary key should be updated?
推荐答案
这个问题我遇到过好几次了.您需要覆盖一些属性:
I've run into this problem a couple of times. You need to override some properties:
protected $primaryKey = ['user_id', 'stock_id'];
public $incrementing = false;
和方法(信用):
/**
* Set the keys for a save update query.
*
* @param IlluminateDatabaseEloquentBuilder $query
* @return IlluminateDatabaseEloquentBuilder
*/
protected function setKeysForSaveQuery(Builder $query)
{
$keys = $this->getKeyName();
if(!is_array($keys)){
return parent::setKeysForSaveQuery($query);
}
foreach($keys as $keyName){
$query->where($keyName, '=', $this->getKeyForSaveQuery($keyName));
}
return $query;
}
/**
* Get the primary key value for a save query.
*
* @param mixed $keyName
* @return mixed
*/
protected function getKeyForSaveQuery($keyName = null)
{
if(is_null($keyName)){
$keyName = $this->getKeyName();
}
if (isset($this->original[$keyName])) {
return $this->original[$keyName];
}
return $this->getAttribute($keyName);
}
记住这段代码需要引用 Eloquent Builder 类
Remember this code needs to reference Eloquent Builder class with
use IlluminateDatabaseEloquentBuilder;
我建议将这些方法放在 HasCompositePrimaryKey
Trait 中,这样你就可以在任何需要它的模型中使用
它.
I suggest putting those methods in a HasCompositePrimaryKey
Trait so you can just use
it in any of your models that need it.
这篇关于带有两个主键的 Laravel 模型更新的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:带有两个主键的 Laravel 模型更新
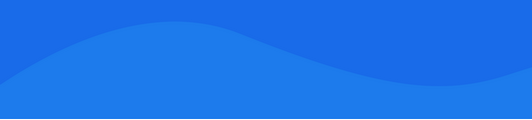
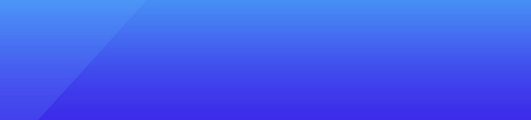
基础教程推荐
- phpmyadmin 错误“#1062 - 密钥 1 的重复条目‘1’" 2022-01-01
- Doctrine 2 - 在多对多关系中记录更改 2022-01-01
- 使用 PDO 转义列名 2021-01-01
- HTTP 与 FTP 上传 2021-01-01
- 如何在 XAMPP 上启用 mysqli? 2021-01-01
- 找不到类“AppHttpControllersDB",我也无法使用新模型 2022-01-01
- 在 CakePHP 2.0 中使用 Html Helper 时未定义的变量 2021-01-01
- PHP 守护进程/worker 环境 2022-01-01
- 在 yii2 中迁移时出现异常“找不到驱动程序" 2022-01-01
- 如何在 Symfony 和 Doctrine 中实现多对多和一对多? 2022-01-01