count relation of relation in laravel(Laravel 中关系的计数关系)
问题描述
假设我有一个像这样的 Conversation
模型:
Suppose I have a Conversation
model like this :
class Conversation extends Model
{
public function questions (){
return $this->hasMany('AppQuestion','conversation_id','conversation_id');
}
public function category ()
{
return $this->belongsTo('AppCategory', 'cat', 'cat_id');
}
}
还有一个像这样的 Question
模型:
And a Question
model like this:
class Question extends Model
{
public function conversation ()
{
return $this->belongsTo('AppConversation', 'conversation_id', 'conversation_id');
}
}
如您所见,这两者之间存在 hasMany
关系.
As you can see there is a hasMany
relation between those two.
另一方面,有一个像下面这样的 Category
与 Conversation
模型有关系:
In the other hand there is a Category
like below that has a relation with Conversation
model :
class Category extends Node
{
public function conversations (){
return $this->hasMany('AppConversation','cat','cat_id');
}
}
现在我想将一个名为 question_count
的属性附加到 Category
来计算每个类别的对话的所有问题.为此,我添加了这个:
Now I want to append an attribute named question_count
to Category
that counts all questions of conversations of each category. for that I added this :
public function getQuestionsCountAttribute ()
{
return $this->conversations->questions->count();
}
但是在获取类别时出现此错误:
But when fetch a category I got this error :
ErrorException in Category.php line 59:
Undefined property: IlluminateDatabaseEloquentCollection::$questions
我做了什么?如何在最小服务器过载的情况下计算关系的关系?
What did I do? how can I count relations of a relation with minimum server overloading?
我使用的是 Laravel 5.3.4.
I am using laravel 5.3.4.
推荐答案
我认为这里你需要一个有很多的关系.
I think that you need a has many through relationship here.
你做错了什么:
当你写$this->conversations->questions
时,这是行不通的,因为questions
是单个对话的关系 而不是对话的集合(这里,$this->conversations
是一个集合)
When you write $this->conversations->questions
, this can't work, because the questions
are a relation of a single conversation and not of a collection of conversations (here, $this->conversations
is a Collection)
解决办法:
使用 hasManyThrough 关系:
Using hasManyThrough relation:
您可以在在此页面上找到有关此关系的文档,如果我的解释不好
You can find the documentation for this relation on this page, if my explanation is bad
基础是,你需要在你的 Category
模型上定义一个关系:
The basics are, you need to define a relation on your Category
model:
class Category extends Node
{
public function conversations ()
{
return $this->hasMany('AppConversation');
}
public function questions ()
{
return $this->hasManyThrough('AppQuestion', 'AppConversation');
}
}
(我会让你查看非标准外键的文档)
(I will let your look into the documentation for your non standards foreign keys)
然后你应该可以使用:$category->questions->count()
这篇关于Laravel 中关系的计数关系的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:Laravel 中关系的计数关系
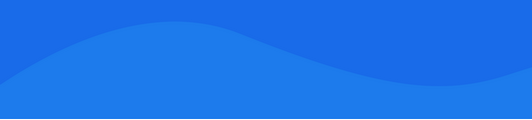
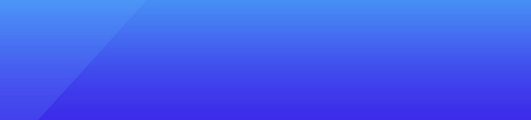
基础教程推荐
- phpmyadmin 错误“#1062 - 密钥 1 的重复条目‘1’" 2022-01-01
- 在 CakePHP 2.0 中使用 Html Helper 时未定义的变量 2021-01-01
- 如何在 XAMPP 上启用 mysqli? 2021-01-01
- 找不到类“AppHttpControllersDB",我也无法使用新模型 2022-01-01
- HTTP 与 FTP 上传 2021-01-01
- 在 yii2 中迁移时出现异常“找不到驱动程序" 2022-01-01
- PHP 守护进程/worker 环境 2022-01-01
- Doctrine 2 - 在多对多关系中记录更改 2022-01-01
- 如何在 Symfony 和 Doctrine 中实现多对多和一对多? 2022-01-01
- 使用 PDO 转义列名 2021-01-01