Laravel 5 eloquent hasManyThrough / belongsToManyThrough relationships(Laravel 5 雄辩的 hasManyThrough/belongsToManyThrough 关系)
问题描述
在 Laravel 5.2 应用程序中,我有三个模型:User
、Role
和 Task
.一个User
关联多个Roles
,一个Role
关联多个Tasks
.因此,每个用户都通过他们的角色与多个任务相关联.
In a Laravel 5.2 application I have three models: User
, Role
and Task
.
A User
is associated with multiple Roles
, and a Role
is associated with multiple Tasks
.
Therefore each user is associated to multiple tasks, through their roles.
我正在尝试通过他们的角色访问与 User
关联的所有 Tasks
.
I am trying to access all Tasks
associated with a User
, through their Roles.
我的模型的相关部分如下所示:
The relevant parts of my models look like:
class User extends Authenticatable
{
public function roles()
{
return $this->belongsToMany('AppRole');
}
public function tasks()
{
return $this->hasManyThrough('AppTask', 'AppRole');
}
}
class Role extends Model
{
public function tasks()
{
return $this->belongsToMany('AppTask');
}
public function users()
{
return $this->belongsToMany('AppUser');
}
}
class Task extends Model
{
public function roles()
{
return $this->belongsToMany('AppRole');
}
}
以下返回SQL错误;
Column not found: 1054 Unknown column 'roles.user_id'
它似乎试图通过 Role 模型中的(不存在的)外键访问关系,而不是通过数据透视表.
It seems to be trying to access the relationship through a (non-existent) foreign key in the Role model, rather than through the pivot table.
$user = Auth::user;
$tasks = $user->tasks;
如何通过这些关系访问与用户相关的所有任务?
How can I access all tasks related to a user through these relationships?
推荐答案
我开发了一个自定义BelongsToManyThrough
关系,您可能会感兴趣.您需要添加新的关系类(在我的要点中给出;粘贴在这里太长了),并且还需要按照要点中的描述覆盖您的基本 Model
类以实现 belongsToManyThrough
.
I have developed a custom BelongsToManyThrough
relationship which might interest you. You would need to add the new relation class (as given in my gist; it is too long to paste here), and also override your base Model
class as described in the gist to implement belongsToManyThrough
.
然后(假设您使用 Laravel 的默认表命名方案 - 如果没有,您也可以指定连接表),您可以将关系定义为:
Then (assuming you are using Laravel's default table naming schemes - if not, you can specify the joining tables as well), you would define your relationship as:
public function tasks()
{
return $this->belongsToManyThrough(
'AppTask',
'AppRole');
}
belongsToManyThrough
不仅会为您提供用户的任务列表,还会告诉您每个用户通过其拥有每个任务的角色.例如,如果您有:
belongsToManyThrough
will not only give you a list of Tasks for your User(s), it will also tell you the Role(s) via which each User has each Task. For example, if you had:
$user->tasks()->get()
输出将类似于:
[
{
"id": 2,
"name": "Ban spammers",
"roles_via": [
{
"id": 2,
"slug": "site-admin",
"name": "Site Administrator",
"description": "This role is meant for "site administrators", who can basically do anything except create, edit, or delete other administrators."
},
{
"id": 3,
"slug": "group-admin",
"name": "Group Administrator",
"description": "This role is meant for "group administrators", who can basically do anything with users in their same group, except other administrators of that group."
}
]
},
{
"id": 13,
"name": "Approve posts",
"roles_via": [
{
"id": 3,
"slug": "group-admin",
"name": "Group Administrator",
"description": "This role is meant for "group administrators", who can basically do anything with users in their same group, except other administrators of that group."
}
]
},
{
"id": 16,
"name": "Reboot server",
"roles_via": [
{
"id": 2,
"slug": "site-admin",
"name": "Site Administrator",
"description": "This role is meant for "site administrators", who can basically do anything except create, edit, or delete other administrators."
}
]
}
]
我的自定义关系有效地完成了这项工作,只需要几个查询,而不是其他涉及 foreach
的解决方案,后者会创建一个 n+1 查询 问题.
My custom relationship does this efficiently, with only a few queries, as opposed to other solutions involving foreach
, which would create an n+1 query problem.
这篇关于Laravel 5 雄辩的 hasManyThrough/belongsToManyThrough 关系的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:Laravel 5 雄辩的 hasManyThrough/belongsToManyThrough 关系
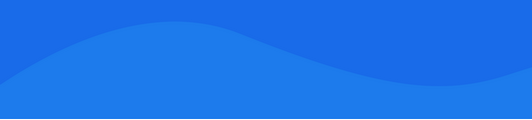
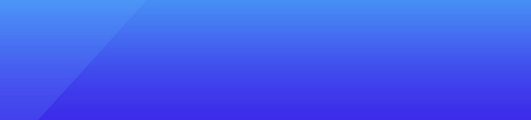
基础教程推荐
- 在 yii2 中迁移时出现异常“找不到驱动程序" 2022-01-01
- PHP 守护进程/worker 环境 2022-01-01
- Doctrine 2 - 在多对多关系中记录更改 2022-01-01
- 使用 PDO 转义列名 2021-01-01
- 如何在 Symfony 和 Doctrine 中实现多对多和一对多? 2022-01-01
- 在 CakePHP 2.0 中使用 Html Helper 时未定义的变量 2021-01-01
- 找不到类“AppHttpControllersDB",我也无法使用新模型 2022-01-01
- 如何在 XAMPP 上启用 mysqli? 2021-01-01
- phpmyadmin 错误“#1062 - 密钥 1 的重复条目‘1’" 2022-01-01
- HTTP 与 FTP 上传 2021-01-01