Best way to INSERT many values in mysqli?(在 mysqli 中插入许多值的最佳方法?)
问题描述
I'm looking for a SQL-injection-secure technique to insert a lot of rows (ca. 2000) at once with PHP and MySQLi.
I have an array with all the values that have to be include.
Currently I'm doing that:
<?php
$array = array("array", "with", "about", "2000", "values");
foreach ($array as $one)
{
$query = "INSERT INTO table (link) VALUES ( ?)";
$stmt = $mysqli->prepare($query);
$stmt ->bind_param("s", $one);
$stmt->execute();
$stmt->close();
}
?>
I tried call_user_func_array(), but it caused a stackoverflow.
What is faster method to do this (like inserting them all at once?), but still secure against SQL-injections (like a prepared statement) and stackoverflows?
Thank you!
You should be able to greatly increase the speed by putting your inserts inside a transaction. You can also move your prepare and bind statements outside of your loop.
$array = array("array", "with", "about", "2000", "values");
$query = "INSERT INTO table (link) VALUES (?)";
$stmt = $mysqli->prepare($query);
$stmt ->bind_param("s", $one);
$mysqli->query("START TRANSACTION");
foreach ($array as $one) {
$stmt->execute();
}
$stmt->close();
$mysqli->query("COMMIT");
I tested this code with 10,000 iterations on my web server.
Without transaction: 226 seconds.
With transaction: 2 seconds.
Or a two order of magnitude speed increase
, at least for that test.
这篇关于在 mysqli 中插入许多值的最佳方法?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:在 mysqli 中插入许多值的最佳方法?
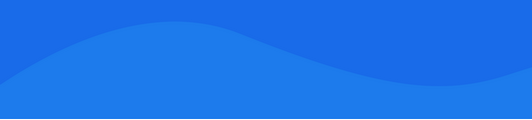
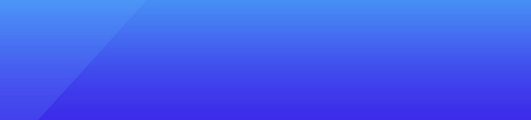
基础教程推荐
- mysqli_insert_id 是否有可能在高流量应用程序中返回 2021-01-01
- 在 PHP 中强制下载文件 - 在 Joomla 框架内 2022-01-01
- Libpuzzle 索引数百万张图片? 2022-01-01
- 通过 PHP SoapClient 请求发送原始 XML 2021-01-01
- 在 Woocommerce 中根据运输方式和付款方式添加费用 2021-01-01
- 如何在 PHP 中的请求之间持久化对象 2022-01-01
- 超薄框架REST服务两次获得输出 2022-01-01
- 在多维数组中查找最大值 2021-01-01
- WooCommerce 中选定产品类别的自定义产品价格后缀 2021-01-01
- XAMPP 服务器不加载 CSS 文件 2022-01-01