How to parse JSON and access results(如何解析 JSON 并访问结果)
本文介绍了如何解析 JSON 并访问结果的处理方法,对大家解决问题具有一定的参考价值,需要的朋友们下面随着小编来一起学习吧!
问题描述
I am using CURL to send a request. The response dataType is json
. How can I parse this data and insert it into the database?
<?php
$url = 'http://sms2.cdyne.com/sms.svc/SimpleSMSsendWithPostback? PhoneNumber=18887477474&Message=test&LicenseKey=LICENSEKEY';
$cURL = curl_init();
curl_setopt($cURL, CURLOPT_URL, $url);
curl_setopt($cURL, CURLOPT_HTTPGET, true);
curl_setopt($cURL, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json',
'Accept: application/json'
));
$result = curl_exec($cURL);
curl_close($cURL);
print_r($result);
?>
JSON Output:
{
"Cancelled": false,
"MessageID": "402f481b-c420-481f-b129-7b2d8ce7cf0a",
"Queued": false,
"SMSError": 2,
"SMSIncomingMessages": null,
"Sent": false,
"SentDateTime": "/Date(-62135578800000-0500)/"
}
解决方案
If your $result
variable is a string json like, you must use json_decode
function to parse it as an object or array:
$result = '{"Cancelled":false,"MessageID":"402f481b-c420-481f-b129-7b2d8ce7cf0a","Queued":false,"SMSError":2,"SMSIncomingMessages":null,"Sent":false,"SentDateTime":"/Date(-62135578800000-0500)/"}';
$json = json_decode($result, true);
print_r($json);
OUTPUT
Array
(
[Cancelled] =>
[MessageID] => 402f481b-c420-481f-b129-7b2d8ce7cf0a
[Queued] =>
[SMSError] => 2
[SMSIncomingMessages] =>
[Sent] =>
[SentDateTime] => /Date(-62135578800000-0500)/
)
Now you can work with $json
variable as an array:
echo $json['MessageID'];
echo $json['SMSError'];
// other stuff
References:
- json_decode - PHP Manual
这篇关于如何解析 JSON 并访问结果的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
沃梦达教程
本文标题为:如何解析 JSON 并访问结果
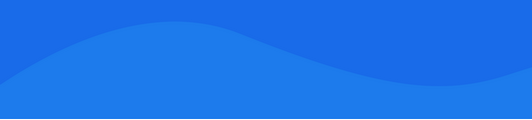
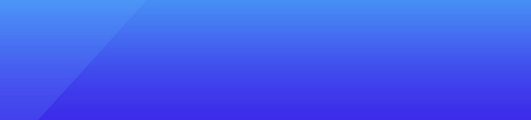
基础教程推荐
猜你喜欢
- WooCommerce 中选定产品类别的自定义产品价格后缀 2021-01-01
- 在 Woocommerce 中根据运输方式和付款方式添加费用 2021-01-01
- 在多维数组中查找最大值 2021-01-01
- 在 PHP 中强制下载文件 - 在 Joomla 框架内 2022-01-01
- 通过 PHP SoapClient 请求发送原始 XML 2021-01-01
- 如何在 PHP 中的请求之间持久化对象 2022-01-01
- 超薄框架REST服务两次获得输出 2022-01-01
- Libpuzzle 索引数百万张图片? 2022-01-01
- XAMPP 服务器不加载 CSS 文件 2022-01-01
- mysqli_insert_id 是否有可能在高流量应用程序中返回 2021-01-01