When should I use #39;self#39; over #39;$this#39;?(我什么时候应该使用 self 而不是 $this?)
问题描述
In PHP 5, what is the difference between using self
and $this
?
When is each appropriate?
Short Answer
Use
$this
to refer to the current object. Useself
to refer to the current class. In other words, use$this->member
for non-static members, useself::$member
for static members.
Full Answer
Here is an example of correct usage of $this
and self
for non-static and static member variables:
<?php
class X {
private $non_static_member = 1;
private static $static_member = 2;
function __construct() {
echo $this->non_static_member . ' '
. self::$static_member;
}
}
new X();
?>
Here is an example of incorrect usage of $this
and self
for non-static and static member variables:
<?php
class X {
private $non_static_member = 1;
private static $static_member = 2;
function __construct() {
echo self::$non_static_member . ' '
. $this->static_member;
}
}
new X();
?>
Here is an example of polymorphism with $this
for member functions:
<?php
class X {
function foo() {
echo 'X::foo()';
}
function bar() {
$this->foo();
}
}
class Y extends X {
function foo() {
echo 'Y::foo()';
}
}
$x = new Y();
$x->bar();
?>
Here is an example of suppressing polymorphic behaviour by using self
for member functions:
<?php
class X {
function foo() {
echo 'X::foo()';
}
function bar() {
self::foo();
}
}
class Y extends X {
function foo() {
echo 'Y::foo()';
}
}
$x = new Y();
$x->bar();
?>
The idea is that
$this->foo()
calls thefoo()
member function of whatever is the exact type of the current object. If the object is oftype X
, it thus callsX::foo()
. If the object is oftype Y
, it callsY::foo()
. But with self::foo(),X::foo()
is always called.
From http://www.phpbuilder.com/board/showthread.php?t=10354489:
By http://board.phpbuilder.com/member.php?145249-laserlight
这篇关于我什么时候应该使用 'self' 而不是 '$this'?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:我什么时候应该使用 'self' 而不是 '$this'?
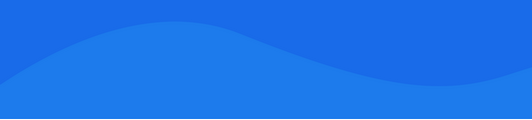
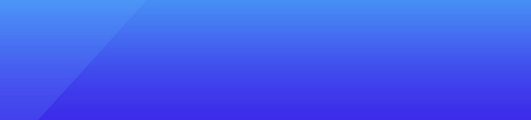
基础教程推荐
- 如何在 PHP 中的请求之间持久化对象 2022-01-01
- Libpuzzle 索引数百万张图片? 2022-01-01
- 在 PHP 中强制下载文件 - 在 Joomla 框架内 2022-01-01
- WooCommerce 中选定产品类别的自定义产品价格后缀 2021-01-01
- 在多维数组中查找最大值 2021-01-01
- 通过 PHP SoapClient 请求发送原始 XML 2021-01-01
- XAMPP 服务器不加载 CSS 文件 2022-01-01
- 超薄框架REST服务两次获得输出 2022-01-01
- mysqli_insert_id 是否有可能在高流量应用程序中返回 2021-01-01
- 在 Woocommerce 中根据运输方式和付款方式添加费用 2021-01-01