Example of polymorphism working with data structure in C++ based on php example(基于php示例的C++中数据结构的多态性示例)
问题描述
I am learning polymorphism and I am familiar with php.
I came across this excellent example from https://stackoverflow.com/a/749738/80353. reproduced below.
How do I write the same code, but in C++?
I have a problem writing it myself because I believe (I may be wrong) that the data structures in C++ are strict.
You must have all the elements inside a linkedlist or array in C++ of the same type.
So I believe that you need to store the cat and dog as their base class into the data structure.
So how do I write this php code snippet into a C++ code snippet that uses a strict data structure that it can only store elements of 1 data type?
class Animal {
var $name;
function __construct($name) {
$this->name = $name;
}
}
class Dog extends Animal {
function speak() {
return "Woof, woof!";
}
}
class Cat extends Animal {
function speak() {
return "Meow...";
}
}
$animals = array(new Dog('Skip'), new Cat('Snowball'));
foreach($animals as $animal) {
print $animal->name . " says: " . $animal->speak() . '<br>';
}
As someone not familiar with C++, create a index.cpp file and fill it up with the following
#include <iostream>
#include <vector>
#include <memory>
using namespace std;
class Animal {
public:
std::string name;
Animal (const std::string& givenName) : name(givenName) {}
virtual string speak () = 0;
virtual ~Animal() {}
};
class Dog: public Animal {
public:
Dog (const std::string& givenName) : Animal (givenName) {
}
string speak ()
{ return "Woof, woof!"; }
};
class Cat: public Animal {
public:
Cat (const std::string& givenName) : Animal (givenName) {
}
string speak ()
{ return "Meow..."; }
};
int main() {
std::vector<std::unique_ptr<Animal>> animals;
animals.push_back( std::unique_ptr<Animal>(new Dog("Skip")) );
animals.push_back( std::unique_ptr<Animal>(new Cat("Snowball")) );
for( int i = 0; i< animals.size(); ++i ) {
cout << animals[i]->name << " says: " << animals[i]->speak() << endl;
}
}
afterwards, compile the index.cpp file with the following command
c++ index.cpp -std=c++11 -stdlib=libc++
you need that because of the use of smart pointers unique_ptr in the code.
finally you can execute the compiled executable output file which should be a.out
./a.out
这篇关于基于php示例的C++中数据结构的多态性示例的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:基于php示例的C++中数据结构的多态性示例
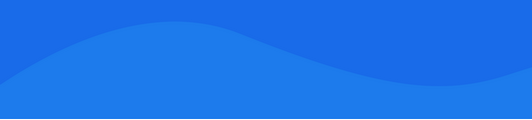
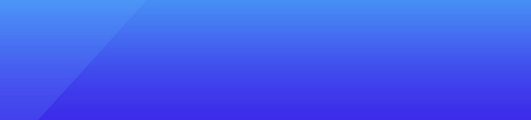
基础教程推荐
- Libpuzzle 索引数百万张图片? 2022-01-01
- 超薄框架REST服务两次获得输出 2022-01-01
- 在 PHP 中强制下载文件 - 在 Joomla 框架内 2022-01-01
- 通过 PHP SoapClient 请求发送原始 XML 2021-01-01
- WooCommerce 中选定产品类别的自定义产品价格后缀 2021-01-01
- 在多维数组中查找最大值 2021-01-01
- 在 Woocommerce 中根据运输方式和付款方式添加费用 2021-01-01
- XAMPP 服务器不加载 CSS 文件 2022-01-01
- 如何在 PHP 中的请求之间持久化对象 2022-01-01
- mysqli_insert_id 是否有可能在高流量应用程序中返回 2021-01-01