What is the best way to password protect folder/page using php without a db or username(在没有数据库或用户名的情况下使用 php 密码保护文件夹/页面的最佳方法是什么)
问题描述
What is the best way to password protect folder using php without a database or user name but using. Basically I have a page that will list contacts for organization and need to password protect that folder without having account for every user . Just one password that gets changes every so often and distributed to the group. I understand that it is not very secure but never the less I would like to know how to do this. In the best way.
It would be nice if the password is remembered for a while once user entered it correctly.
I am doing approximately what David Heggie suggested, except without cookies. It does seem insecure as hell, but it is probably better having a bad password protection then none at all.
This is for internal site where people would have hell of a time remembering their login and password and would never go through sign up process... unless it is really easy they would not use the system at all.
I wanted to see other solutions to this problem.
With user base consisting of not very tech savvy people what are other ways to do this.
Edit: SHA1 is no longer considered secure. Stored password hashes should also be salted. There are now much better solutions to this problem.
You could use something like this:
//access.php
<?php
//put sha1() encrypted password here - example is 'hello'
$password = 'aaf4c61ddcc5e8a2dabede0f3b482cd9aea9434d';
session_start();
if (!isset($_SESSION['loggedIn'])) {
$_SESSION['loggedIn'] = false;
}
if (isset($_POST['password'])) {
if (sha1($_POST['password']) == $password) {
$_SESSION['loggedIn'] = true;
} else {
die ('Incorrect password');
}
}
if (!$_SESSION['loggedIn']): ?>
<html><head><title>Login</title></head>
<body>
<p>You need to login</p>
<form method="post">
Password: <input type="password" name="password"> <br />
<input type="submit" name="submit" value="Login">
</form>
</body>
</html>
<?php
exit();
endif;
?>
Then on each file you want to protect, put at the top:
<?php
require('access.php');
?>
secret text
It isn't a very nice solution, but it might do what you want
Edit
You could add a logout.php page like:
<?php
session_start();
$_SESSION['loggedIn'] = false;
?>
You have logged out
这篇关于在没有数据库或用户名的情况下使用 php 密码保护文件夹/页面的最佳方法是什么的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:在没有数据库或用户名的情况下使用 php 密码保护文件夹/页面的最佳方法是什么
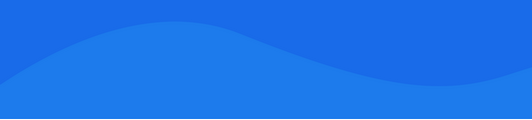
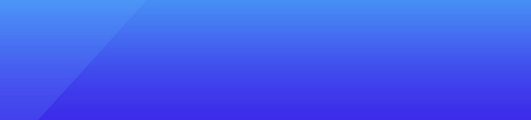
基础教程推荐
- 超薄框架REST服务两次获得输出 2022-01-01
- Libpuzzle 索引数百万张图片? 2022-01-01
- XAMPP 服务器不加载 CSS 文件 2022-01-01
- mysqli_insert_id 是否有可能在高流量应用程序中返回 2021-01-01
- 在多维数组中查找最大值 2021-01-01
- 在 PHP 中强制下载文件 - 在 Joomla 框架内 2022-01-01
- WooCommerce 中选定产品类别的自定义产品价格后缀 2021-01-01
- 在 Woocommerce 中根据运输方式和付款方式添加费用 2021-01-01
- 通过 PHP SoapClient 请求发送原始 XML 2021-01-01
- 如何在 PHP 中的请求之间持久化对象 2022-01-01