What is the purpose of the return statement? How is it different from printing?(return 语句的目的是什么?它与印刷有何不同?)
问题描述
什么是return语句的简单基本解释,在Python中如何使用?
What is the simple basic explanation of what the return statement is, how to use it in Python?
它和print
语句有什么区别?
推荐答案
print()
函数在控制台中写入,即打印"一个字符串.return
语句使你的函数退出并将一个值返回给它的调用者.一般来说,函数的重点是接受输入并返回一些东西.return
语句在函数准备好向其调用者返回值时使用.
The print()
function writes, i.e., "prints", a string in the console. The return
statement causes your function to exit and hand back a value to its caller. The point of functions in general is to take in inputs and return something. The return
statement is used when a function is ready to return a value to its caller.
例如,这是一个同时使用 print()
和 return
的函数:
For example, here's a function utilizing both print()
and return
:
def foo():
print("hello from inside of foo")
return 1
现在您可以运行调用 foo 的代码,如下所示:
Now you can run code that calls foo, like so:
if __name__ == '__main__':
print("going to call foo")
x = foo()
print("called foo")
print("foo returned " + str(x))
如果您将其作为脚本(例如 .py
文件)而不是在 Python 解释器中运行,您将获得以下输出:
If you run this as a script (e.g. a .py
file) as opposed to in the Python interpreter, you will get the following output:
going to call foo
hello from inside foo
called foo
foo returned 1
我希望这会让它更清楚.解释器将返回值写入控制台,这样我就能明白为什么有人会感到困惑.
I hope this makes it clearer. The interpreter writes return values to the console so I can see why somebody could be confused.
下面是来自解释器的另一个例子,它证明了这一点:
Here's another example from the interpreter that demonstrates that:
>>> def foo():
... print("hello from within foo")
... return 1
...
>>> foo()
hello from within foo
1
>>> def bar():
... return 10 * foo()
...
>>> bar()
hello from within foo
10
可以看到,当 bar()
调用 foo()
时,1 并没有写入控制台.相反,它用于计算从 bar()
返回的值.
You can see that when foo()
is called from bar()
, 1 isn't written to the console. Instead it is used to calculate the value returned from bar()
.
print()
是一个会导致副作用的函数(它在控制台中写入一个字符串),但会从下一条语句继续执行.return
使函数停止执行并将值返回给调用它的任何对象.
print()
is a function that causes a side effect (it writes a string in the console), but execution resumes with the next statement. return
causes the function to stop executing and hand a value back to whatever called it.
这篇关于return 语句的目的是什么?它与印刷有何不同?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:return 语句的目的是什么?它与印刷有何不同?
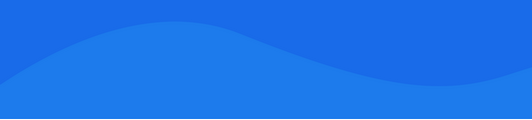
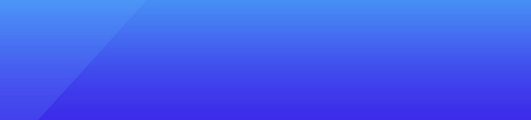
基础教程推荐
- Python kivy 入口点 inflateRest2 无法定位 libpng16-16.dll 2022-01-01
- 使用PyInstaller后在Windows中打开可执行文件时出错 2022-01-01
- Dask.array.套用_沿_轴:由于额外的元素([1]),使用dask.array的每一行作为另一个函数的输入失败 2022-01-01
- 如何让 python 脚本监听来自另一个脚本的输入 2022-01-01
- 筛选NumPy数组 2022-01-01
- 线程时出现 msgbox 错误,GUI 块 2022-01-01
- 如何在海运重新绘制中自定义标题和y标签 2022-01-01
- 何时使用 os.name、sys.platform 或 platform.system? 2022-01-01
- 用于分类数据的跳跃记号标签 2022-01-01
- 在 Python 中,如果我在一个“with"中返回.块,文件还会关闭吗? 2022-01-01