How do I receive multipart form data in Azure function?(如何在 Azure 函数中接收多部分表单数据?)
本文介绍了如何在 Azure 函数中接收多部分表单数据?的处理方法,对大家解决问题具有一定的参考价值,需要的朋友们下面随着小编来一起学习吧!
问题描述
我想在 Azure Function 中接收包含图像和文本的多部分数据.
我可以在 C# 和节点中看到很多示例
I want to receive multipart data containing images and texts in Azure Function.
I could see a lot of examples in C# and nodeNode link but Could not find anything in python.
Could not find anything in official docs as well Docs
解决方案
I have tried the below code and it works for me:
import logging
import json
import io
from PIL import Image
from azure.storage.blob import BlobServiceClient,BlobClient
import azure.functions as func
def main(req: func.HttpRequest) -> func.HttpResponse:
logging.info('Python HTTP trigger function processed a request.')
name = req.form['name']
interests =json.dumps(req.form["interests"], indent=2)
imagefile = req.files["file"]
filename = imagefile.filename
filestream = imagefile.stream
filestream.seek(0)
blob = BlobClient.from_connection_string(conn_str= "<your connection string>", container_name="<container name>", blob_name="<blob name>")
blob.upload_blob(filestream.read(), blob_type="BlockBlob")
if name:
return func.HttpResponse(f"Hello, {name}. Created profile with your interests : {interests}. Uploaded your profile image file :{filename} to the blob. This function executed successfully.")
else:
return func.HttpResponse(
"This HTTP triggered function executed successfully. Pass a name in the query string or in the request body for a personalized response.",
status_code=200
)
However, you can also use PIL in this case to get the bytes from the image . Then the code looks like :
import logging
import json
import io
from PIL import Image
from azure.storage.blob import BlobServiceClient,BlobClient
import azure.functions as func
def main(req: func.HttpRequest) -> func.HttpResponse:
logging.info('Python HTTP trigger function processed a request.')
name = req.form['name']
interests =json.dumps(req.form["interests"], indent=2)
filename = req.files["file"].filename
image = Image.open(req.files["file"])
imgByteIO = io.BytesIO()
image.save(imgByteIO, format=image.format)
imgByteArr = imgByteIO.getvalue()
blob = BlobClient.from_connection_string(conn_str= "<your connection string>", container_name="<container name>", blob_name="<blob name>")
blob.upload_blob(imgByteArr)
if name:
return func.HttpResponse(f"Hello, {name}. Created profile with your interests : {interests}. Uploaded your profile image file :{filename} to the blob. This function executed successfully.")
else:
return func.HttpResponse(
"This HTTP triggered function executed successfully. Pass a name in the query string or in the request body for a personalized response.",
status_code=200
)
Here is the postman request:
这篇关于如何在 Azure 函数中接收多部分表单数据?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
沃梦达教程
本文标题为:如何在 Azure 函数中接收多部分表单数据?
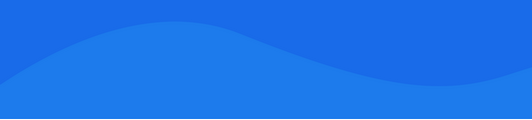
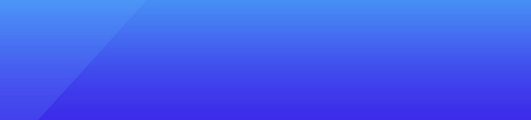
基础教程推荐
猜你喜欢
- 在 Python 中,如果我在一个“with"中返回.块,文件还会关闭吗? 2022-01-01
- Python kivy 入口点 inflateRest2 无法定位 libpng16-16.dll 2022-01-01
- Dask.array.套用_沿_轴:由于额外的元素([1]),使用dask.array的每一行作为另一个函数的输入失败 2022-01-01
- 使用PyInstaller后在Windows中打开可执行文件时出错 2022-01-01
- 线程时出现 msgbox 错误,GUI 块 2022-01-01
- 用于分类数据的跳跃记号标签 2022-01-01
- 如何在海运重新绘制中自定义标题和y标签 2022-01-01
- 筛选NumPy数组 2022-01-01
- 何时使用 os.name、sys.platform 或 platform.system? 2022-01-01
- 如何让 python 脚本监听来自另一个脚本的输入 2022-01-01