NLTK tree data structure, finding a node, it#39;s parent or children(NLTK 树数据结构,找到一个节点,它是父节点还是子节点)
问题描述
I am using nltk's Tree data structure to work with parsetree strings.
from nltk.tree import Tree
parsed = Tree('(ROOT (S (NP (PRP It)) (VP (VBZ is) (ADJP (RB so) (JJ nice))) (. .)))')
The data structure, however, seems to be limited. Is it possible to get a node by it's string value and then navigate to top or bottom?
For example suppose you want to get the node with string value 'nice' and then see what's its parent, children, etc. Is it achievable via nltk's Tree?
For NLTK 3.0, you want to use the ParentedTree subclass.
http://www.nltk.org/api/nltk.html#nltk.tree.ParentedTree
Using the sample tree you've given, create a ParentedTree and search for the node you want:
from nltk.tree import ParentedTree
ptree = ParentedTree.fromstring('(ROOT (S (NP (PRP It))
(VP (VBZ is) (ADJP (RB so) (JJ nice))) (. .)))')
leaf_values = ptree.leaves()
if 'nice' in leaf_values:
leaf_index = leaf_values.index('nice')
tree_location = ptree.leaf_treeposition(leaf_index)
print tree_location
print ptree[tree_location]
You can iterate through the tree directly to get the child subtrees. The parent() method is used to find the parent tree for the given subtree.
Here's an example using a deeper tree for child and parent:
from nltk.tree import ParentedTree
ptree = ParentedTree.fromstring('(ROOT (S (NP (JJ Congressional)
(NNS representatives)) (VP (VBP are) (VP (VBN motivated)
(PP (IN by) (NP (NP (ADJ shiny) (NNS money))))))) (. .))')
def traverse(t):
try:
t.label()
except AttributeError:
return
else:
if t.height() == 2: #child nodes
print t.parent()
return
for child in t:
traverse(child)
traverse(ptree)
这篇关于NLTK 树数据结构,找到一个节点,它是父节点还是子节点的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:NLTK 树数据结构,找到一个节点,它是父节点还是
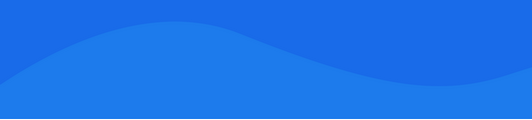
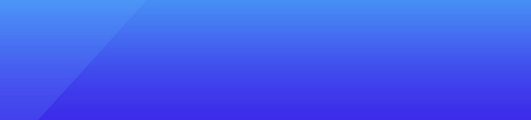
基础教程推荐
- 线程时出现 msgbox 错误,GUI 块 2022-01-01
- 如何在海运重新绘制中自定义标题和y标签 2022-01-01
- Python kivy 入口点 inflateRest2 无法定位 libpng16-16.dll 2022-01-01
- 如何让 python 脚本监听来自另一个脚本的输入 2022-01-01
- 在 Python 中,如果我在一个“with"中返回.块,文件还会关闭吗? 2022-01-01
- 何时使用 os.name、sys.platform 或 platform.system? 2022-01-01
- 用于分类数据的跳跃记号标签 2022-01-01
- 使用PyInstaller后在Windows中打开可执行文件时出错 2022-01-01
- 筛选NumPy数组 2022-01-01
- Dask.array.套用_沿_轴:由于额外的元素([1]),使用dask.array的每一行作为另一个函数的输入失败 2022-01-01