How to get leaf nodes of a tree using Python?(如何使用Python获取树的叶节点?)
问题描述
我是 OOP 的新手,所以在阅读本文时请记住这一点.
Hi there I am new to OOP so have this in mind while you are reading this.
我有一个简单的 Python 树实现(见下面的代码).
I have a simple Python tree implementation(see below code).
class TreeNode(object):
def __init__(self, data):
self.data = data
self.children = []
def add_child(self, obj):
self.children.append(obj)
class Tree:
def __init__(self):
self.root = TreeNode('ROOT')
def preorder_trav(self, node):
if node is not None:
print node.data
if len(node.children) == 0:
print "("+ node.data + ")"
for n in node.children:
self.preorder_trav(n)
if __name__ == '__main__':
tr = Tree()
n1 = tr.root
n2 = TreeNode("B")
n3 = TreeNode("C")
n4 = TreeNode("D")
n5 = TreeNode("E")
n6 = TreeNode("F")
n1.add_child(n2)
n1.add_child(n3)
n2.add_child(n4)
n2.add_child(n5)
n3.add_child(n6)
tr.preorder_trav(n1)
我现在需要的是实现一种让叶节点恢复的方法.术语叶节点是指没有子节点的节点.
What I need now is to implement a method for getting Leaf Nodes back. By the term leaf node I mean a node that has no children.
我想知道如何制作 get_leaf_nodes() 方法.
I am wondering how to make a get_leaf_nodes() method.
我想到的一些解决方案是
Some solutions come to my mind are
- 在
__init__
方法中创建一个self.leaf_nodes = []
.通过这样做,我知道它只会被这个树实例看到. - 在
__init__
方法之上创建一个类成员leaf_nodes = []
.通过这样做,我知道所有树实例都将能够看到 Leaf_nodes 列表.
- Making a
self.leaf_nodes = []
inside the__init__
method. By making this I know it will be seen only by this tree instance. - Making a class member
leaf_nodes = []
above__init__
method. By making this I know all tree instances will be able to see leaf_nodes list.
上述解决方案将导致我在我的类中创建一个 Leaf_nodes 列表,以便 get_leaf_nodes()
方法可以使用.我正在寻找的是只有一个 get_leaf_nodes()
方法,它将在我的树上进行计算并返回一个列表.
The above solutions will cause me to create a leaf_nodes list inside my class so the get_leaf_nodes()
method could use. What I am looking for is to only have a get_leaf_nodes()
method that will do the computation on my tree and will return a list.
例如在 C 中,我们会调用 malloc()
,然后我们可以返回指向调用 get_leaf_nodes()
的函数的指针.
For example in C we would call malloc()
and then we could return the pointer to the function that called the get_leaf_nodes()
.
推荐答案
在python中你可以使用一个内部函数来收集叶子节点,然后返回它们的列表.
In python you can use an internal function to collect the leaf nodes and then return the list of them.
def get_leaf_nodes(self):
leafs = []
def _get_leaf_nodes( node):
if node is not None:
if len(node.children) == 0:
leafs.append(node)
for n in node.children:
_get_leaf_nodes(n)
_get_leaf_nodes(self.root)
return leafs
如果您想要更干净的 OOP 方法,您可以为叶子集合创建一个额外的私有方法:
If you want a more clean OOP approach you can create an extra private method for the collection of leafs:
def get_leaf_nodes(self):
leafs = []
self._collect_leaf_nodes(self.root,leafs)
return leafs
def _collect_leaf_nodes(self, node, leafs):
if node is not None:
if len(node.children) == 0:
leafs.append(node)
for n in node.children:
self._collect_leaf_nodes(n, leafs)
这是我在 Java 中的做法.
This is the way I'd do it in Java.
这篇关于如何使用Python获取树的叶节点?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何使用Python获取树的叶节点?
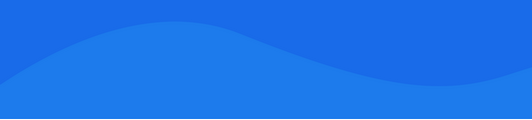
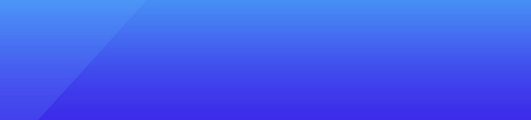
基础教程推荐
- 如何在海运重新绘制中自定义标题和y标签 2022-01-01
- 使用PyInstaller后在Windows中打开可执行文件时出错 2022-01-01
- Dask.array.套用_沿_轴:由于额外的元素([1]),使用dask.array的每一行作为另一个函数的输入失败 2022-01-01
- 在 Python 中,如果我在一个“with"中返回.块,文件还会关闭吗? 2022-01-01
- 用于分类数据的跳跃记号标签 2022-01-01
- Python kivy 入口点 inflateRest2 无法定位 libpng16-16.dll 2022-01-01
- 如何让 python 脚本监听来自另一个脚本的输入 2022-01-01
- 线程时出现 msgbox 错误,GUI 块 2022-01-01
- 何时使用 os.name、sys.platform 或 platform.system? 2022-01-01
- 筛选NumPy数组 2022-01-01