Read data from a file and create a tree using anytree in python(从文件中读取数据并在 python 中使用 anytree 创建一棵树)
问题描述
有没有办法从文件中读取数据并使用 anytree 构造一棵树?
父子A1A2A2 A21我可以使用静态值进行如下操作.但是,我想通过使用 anytree 从文件中读取数据来自动执行此操作.
<预><代码>>>>从 anytree 导入节点,RenderTree>>>A = 节点(A")>>>A1 = 节点(A1",父 = A)>>>A2 = 节点(A2",父 = A)>>>A21 = 节点(A21",父 = A2)
输出是
A├── A1└── A2└── A21
这假设条目的顺序是这样的:父节点总是事先作为另一个节点的子节点引入(根除外).
考虑到这一点,我们可以遍历这些行,拆分它们(我使用了 split
,正则表达式也可以)并创建新节点.
关于如何通过名称获取对父级的引用,我想出了两个解决方案:
首先,使用anytreesfind_by_attr
from anytree import Node, RenderTree, find_by_attrwith open('input.txt', 'r') as f:行 = f.readlines()[1:]root = Node(lines[0].split(" ")[0])对于线中线:line = line.split(" ")Node("".join(line[1:]).strip(), parent=find_by_attr(root, line[0]))对于 RenderTree(root) 中的 pre, _, 节点:print("%s%s" % (pre, node.name))
<小时>
第二,在我们创建它们时将它们缓存在字典中:
from anytree import Node, RenderTree, find_by_attrwith open('input.txt', 'r') as f:行 = f.readlines()[1:]root = Node(lines[0].split(" ")[0])节点 = {}节点[root.name] = 根对于线中线:line = line.split(" ")name = "".join(line[1:]).strip()节点[名称] = 节点(名称,父节点=节点[行[0]])对于 RenderTree(root) 中的 pre, _, 节点:print("%s%s" % (pre, node.name))
<小时>
input.txt
父子A1A2A2 A21
<小时>
输出:
A├── A1└── A2└── A21
Is there a way to read data from a file and construct a tree using anytree?
Parent Child
A A1
A A2
A2 A21
I can do it with static values as follows. However, I want to automate this by reading the data from a file with anytree.
>>> from anytree import Node, RenderTree
>>> A = Node("A")
>>> A1 = Node("A1", parent=A)
>>> A2 = Node("A2", parent=A)
>>> A21 = Node("A21", parent=A2)
Output is
A
├── A1
└── A2
└── A21
This assumes that the entries are in such an order that a parent node was always introduced as a child of another node beforehand (root excluded).
With that in mind, we can then iterate over the lines, split them (I used split
, regex would work too) and create the new nodes.
For how to get a reference to the parent by name, I came up with two solutions:
First, find the parent by name using anytrees find_by_attr
from anytree import Node, RenderTree, find_by_attr
with open('input.txt', 'r') as f:
lines = f.readlines()[1:]
root = Node(lines[0].split(" ")[0])
for line in lines:
line = line.split(" ")
Node("".join(line[1:]).strip(), parent=find_by_attr(root, line[0]))
for pre, _, node in RenderTree(root):
print("%s%s" % (pre, node.name))
Second, just cache them in a dict while we create them:
from anytree import Node, RenderTree, find_by_attr
with open('input.txt', 'r') as f:
lines = f.readlines()[1:]
root = Node(lines[0].split(" ")[0])
nodes = {}
nodes[root.name] = root
for line in lines:
line = line.split(" ")
name = "".join(line[1:]).strip()
nodes[name] = Node(name, parent=nodes[line[0]])
for pre, _, node in RenderTree(root):
print("%s%s" % (pre, node.name))
input.txt
Parent Child
A A1
A A2
A2 A21
Output:
A
├── A1
└── A2
└── A21
这篇关于从文件中读取数据并在 python 中使用 anytree 创建一棵树的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:从文件中读取数据并在 python 中使用 anytree 创建一
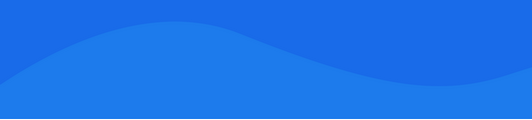
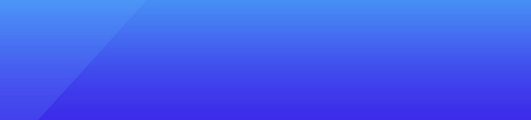
基础教程推荐
- Dask.array.套用_沿_轴:由于额外的元素([1]),使用dask.array的每一行作为另一个函数的输入失败 2022-01-01
- 如何在海运重新绘制中自定义标题和y标签 2022-01-01
- 线程时出现 msgbox 错误,GUI 块 2022-01-01
- 筛选NumPy数组 2022-01-01
- 何时使用 os.name、sys.platform 或 platform.system? 2022-01-01
- 使用PyInstaller后在Windows中打开可执行文件时出错 2022-01-01
- 如何让 python 脚本监听来自另一个脚本的输入 2022-01-01
- Python kivy 入口点 inflateRest2 无法定位 libpng16-16.dll 2022-01-01
- 用于分类数据的跳跃记号标签 2022-01-01
- 在 Python 中,如果我在一个“with"中返回.块,文件还会关闭吗? 2022-01-01