How to get click handler on animated canvas dot on MapBox map?(如何在MapBox地图上的动画画布点上获得点击处理程序?)
问题描述
我正在查看MapBox中的this demo:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Add an animated icon to the map</title>
<meta name="viewport" content="initial-scale=1,maximum-scale=1,user-scalable=no">
<link href="https://api.mapbox.com/mapbox-gl-js/v2.3.1/mapbox-gl.css" rel="stylesheet">
<script src="https://api.mapbox.com/mapbox-gl-js/v2.3.1/mapbox-gl.js"></script>
<style>
body { margin: 0; padding: 0; }
#map { position: absolute; top: 0; bottom: 0; width: 100%; }
</style>
</head>
<body>
<div id="map"></div>
<script>
mapboxgl.accessToken = 'pk.thekey.12345';
var map = new mapboxgl.Map({
container: 'map',
style: 'mapbox://styles/mapbox/streets-v9'
});
var size = 200;
// This implements `StyleImageInterface`
// to draw a pulsing dot icon on the map.
var pulsingDot = {
width: size,
height: size,
data: new Uint8Array(size * size * 4),
// When the layer is added to the map,
// get the rendering context for the map canvas.
onAdd: function() {
var canvas = document.createElement('canvas');
canvas.width = this.width;
canvas.height = this.height;
this.context = canvas.getContext('2d');
},
// Call once before every frame where the icon will be used.
render: function() {
var duration = 1000;
var t = (performance.now() % duration) / duration;
var radius = (size / 2) * 0.3;
var outerRadius = (size / 2) * 0.7 * t + radius;
var context = this.context;
// Draw the outer circle.
context.clearRect(0, 0, this.width, this.height);
context.beginPath();
context.arc(
this.width / 2,
this.height / 2,
outerRadius,
0,
Math.PI * 2
);
context.fillStyle = 'rgba(255, 200, 200,' + (1 - t) + ')';
context.fill();
// Draw the inner circle.
context.beginPath();
context.arc(
this.width / 2,
this.height / 2,
radius,
0,
Math.PI * 2
);
context.fillStyle = 'rgba(255, 100, 100, 1)';
context.strokeStyle = 'white';
context.lineWidth = 2 + 4 * (1 - t);
context.fill();
context.stroke();
// Update this image's data with data from the canvas.
this.data = context.getImageData(
0,
0,
this.width,
this.height
).data;
// Continuously repaint the map, resulting
// in the smooth animation of the dot.
map.triggerRepaint();
// Return `true` to let the map know that the image was updated.
return true;
}
};
map.on('load', function() {
map.addImage('pulsing-dot', pulsingDot, {
pixelRatio: 2
});
map.addSource('dot-point', {
'type': 'geojson',
'data': {
'type': 'FeatureCollection',
'features': [{
'type': 'Feature',
'geometry': {
'type': 'Point',
'coordinates': [0, 0] // icon position [lng, lat]
}
}]
}
});
map.addLayer({
'id': 'layer-with-pulsing-dot',
'type': 'symbol',
'source': 'dot-point',
'layout': {
'icon-image': 'pulsing-dot'
}
});
});
</script>
</body>
</html>
如何使我可以处理单击点,并在您将光标悬停在其上方时使其成为指针?
推荐答案
事情实际上并不像看起来那么难。正如其他人建议的那样,您可以使用一些技巧来实现这一点-但这并不是必需的,因为所需的功能还没有内置到Mapbox本身!
如果您在API reference中查找它自己的map
对象,您会注意到有几个有趣的事件:
单击、鼠标输入和鼠标离开
通常将这些绑定到实际地图上,并添加如下内容:
map.on('click', function() {
console.log('clicked');
});
现在,您可能会问自己,这对您有什么好处。如果我们回顾一下您提供的示例代码,我们可以看到动画圆点被添加到它自己的图层layer-with-pulsing-dot
上的地图中。
API引用没有真正提到的一件事是,.on()
方法有一个额外的第二个参数,它让您为特定层指定一个ID。
所以我们要做的就是向layer-with-pulsing-dot
层添加一些监听器。
单击事件当然很明显,mouseenter
和mouseleave
事件用于将鼠标光标转换为指针并将其转换回来。
尝试在map.addLayer({ ... });
后添加此挡路代码
map.on('click', 'layer-with-pulsing-dot', function(e) {
alert('Someone clicked long:' + e.lngLat.lng + ' lat:' + e.lngLat.lat)
});
map.on('mouseenter', 'layer-with-pulsing-dot', function() {
map.getCanvas().style.cursor = 'pointer';
});
map.on('mouseleave', 'layer-with-pulsing-dot', function() {
map.getCanvas().style.cursor = '';
});
这篇关于如何在MapBox地图上的动画画布点上获得点击处理程序?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何在MapBox地图上的动画画布点上获得点击处理程序?
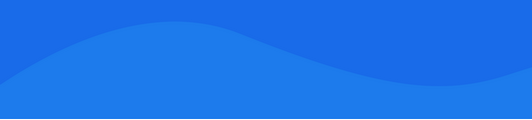
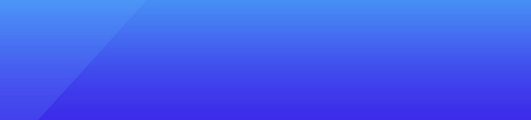
基础教程推荐
- 我什么时候应该在导入时使用方括号 2022-01-01
- 在 JS 中获取客户端时区(不是 GMT 偏移量) 2022-01-01
- 响应更改 div 大小保持纵横比 2022-01-01
- 在for循环中使用setTimeout 2022-01-01
- 角度Apollo设置WatchQuery结果为可用变量 2022-01-01
- 有没有办法使用OpenLayers更改OpenStreetMap中某些要素 2022-09-06
- 动态更新多个选择框 2022-01-01
- Karma-Jasmine:如何正确监视 Modal? 2022-01-01
- 悬停时滑动输入并停留几秒钟 2022-01-01
- 当用户滚动离开时如何暂停 youtube 嵌入 2022-01-01