jQuery image hover effect(jQuery图像悬停效果)
问题描述
I'm trying to achieve this effect with jQuery.
I wrote some of the code, but it's buggy (move to the bottom-right corder and you'll see).
check it out
Basically, if there's an already-built jQuery plugin that you know of that does this, I'd be very happy using it, if not, any help with my formula would be appreciated. This is what I get for not paying attention in Maths classes :)
Thanks in advance.
Maikel
Overall I think this is what you're looking for:
$.fn.sexyImageHover = function() {
var p = this, // parent
i = this.children('img'); // image
i.load(function(){
// get image and parent width/height info
var pw = p.width(),
ph = p.height(),
w = i.width(),
h = i.height();
// check if the image is actually larger than the parent
if (w > pw || h > ph) {
var w_offset = w - pw,
h_offset = h - ph;
// center the image in the view by default
i.css({ 'margin-top':(h_offset / 2) * -1, 'margin-left':(w_offset / 2) * -1 });
p.mousemove(function(e){
var new_x = 0 - w_offset * e.offsetX / w,
new_y = 0 - h_offset * e.offsetY / h;
i.css({ 'margin-top':new_y, 'margin-left':new_x });
});
}
});
}
You can test it here.
Notable changes:
new_x
andnew_y
should be divided by the images height/width, not the container's height/width, which is wider.this
is already a jQuery object in a$.fn.plugin
function, no need to wrap it.i
andp
were also jQuery objects, no need to keep wrapping them
- no need to bind
mousemove
onmouseenter
(which rebinds) themousemove
will only occur when you're inside anyway.
这篇关于jQuery图像悬停效果的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:jQuery图像悬停效果
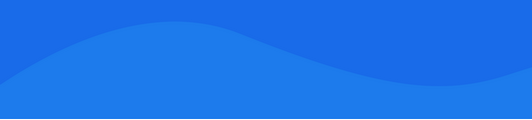
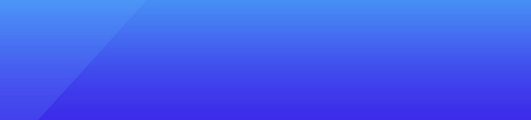
基础教程推荐
- 我什么时候应该在导入时使用方括号 2022-01-01
- 当用户滚动离开时如何暂停 youtube 嵌入 2022-01-01
- 在 JS 中获取客户端时区(不是 GMT 偏移量) 2022-01-01
- 动态更新多个选择框 2022-01-01
- 角度Apollo设置WatchQuery结果为可用变量 2022-01-01
- 在for循环中使用setTimeout 2022-01-01
- 响应更改 div 大小保持纵横比 2022-01-01
- 有没有办法使用OpenLayers更改OpenStreetMap中某些要素 2022-09-06
- 悬停时滑动输入并停留几秒钟 2022-01-01
- Karma-Jasmine:如何正确监视 Modal? 2022-01-01