Dynamically filter for-loop data source in Vue3(在Vue3中动态过滤for循环数据源)
问题描述
目标
我想根据搜索栏的输入筛选在for循环中使用的数据源。
示例
我有数据源[{TITLE:&Q;TITLE1&Q;},{TITLE:&Q;TITLE2&Q;}]。我在我的搜索栏中输入标题1。我只希望for循环的经过筛选的数据源显示标题为1的对象
问题
搜索输入更改(...)方法似乎起作用了,this.filteredPosts数据源似乎更改正确。但是,在HTML模板中,数据源似乎没有更新。它始终同时包含两个对象。
Home.vue
<template>
<Searchfield @searchInputChange="searchInputChange" />
<div v-for="post in filteredPosts" :key="post.id">
{{ post.title }}
</div>
</template>
<script>
import { ref } from 'vue'
import Searchfield from '../components/Searchfield.vue'
export default {
name: 'Home',
components: { Searchfield },
setup() {
let filteredPosts = ref([])
let allPosts = [
{
"id": 1,
"title": "Title1",
},
{
"id": 2,
"title": "Title2",
}
]
filteredPosts.value = [...allPosts]
return { allPosts, filteredPosts }
},
methods: {
searchInputChange(value) {
const filterPosts = this.allPosts.filter(post => post.title.toLowerCase().includes(value.toLowerCase()));
this.filteredPosts.value = [...filterPosts]
}
}
}
</script>
Searchfield.vue
<template>
<input type="text" @change="searchInputChange" placeholder="Search...">
</template>
<script>
export default {
name: 'Searchfield',
setup(props, context) {
const searchInputChange = (event) => {
context.emit('searchInputChange', event.target.value)
}
return { searchInputChange }
}
}
</script>
谢谢!
推荐答案
在setup
中使用ref时,需要使用value
属性更改它的值(或读取值),以便setup
到filteredPosts
的所有赋值都应该类似于filteredPosts.value = ....
在setup
(比如说methods
)之外,filteredPosts
是反应对象(组件实例)的正常反应属性,不需要.value
(使用它是错误的)
所有这些都非常令人困惑,这也是不建议混合使用组合API和选项API的原因之一--只使用其中之一。
在您的情况下,filteredPosts
可以是一个简单的computed
,而无需在每次更改输入时手动重新分配它...
const app = Vue.createApp({
setup(){
const searchTerm = Vue.ref('')
let allPosts = [
{
"id": 1,
"title": "Title1",
},
{
"id": 2,
"title": "Title2",
}
]
const filteredPosts = Vue.computed(() => allPosts.filter(post => post.title.toLowerCase().includes(searchTerm.value.toLowerCase())) )
return {
searchTerm,
filteredPosts
}
},
})
app.component('Searchfield', {
props: ['modelValue'],
emits: ['update:modelValue'],
setup(props, { emit }) {
const model = Vue.computed({
get: () => props.modelValue,
set: (newValue) => emit('update:modelValue', newValue)
})
return { model }
},
template: `<input type="text" v-model="model" placeholder="Search...">`
})
app.mount('#app')
<script src="https://unpkg.com/vue@3.2.23/dist/vue.global.js"></script>
<div id='app'>
<searchfield v-model="searchTerm"></searchfield>
<div v-for="post in filteredPosts" :key="post.id">
{{ post.title }}
</div>
</div>
这篇关于在Vue3中动态过滤for循环数据源的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:在Vue3中动态过滤for循环数据源
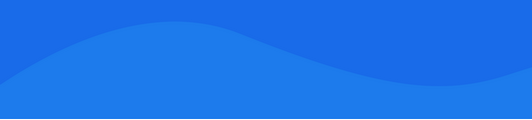
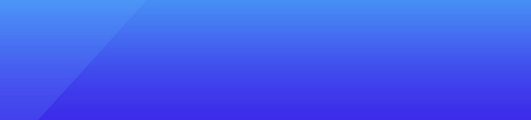
基础教程推荐
- 悬停时滑动输入并停留几秒钟 2022-01-01
- 响应更改 div 大小保持纵横比 2022-01-01
- 我什么时候应该在导入时使用方括号 2022-01-01
- 动态更新多个选择框 2022-01-01
- 角度Apollo设置WatchQuery结果为可用变量 2022-01-01
- 有没有办法使用OpenLayers更改OpenStreetMap中某些要素 2022-09-06
- Karma-Jasmine:如何正确监视 Modal? 2022-01-01
- 在 JS 中获取客户端时区(不是 GMT 偏移量) 2022-01-01
- 当用户滚动离开时如何暂停 youtube 嵌入 2022-01-01
- 在for循环中使用setTimeout 2022-01-01