How do I write a sequence of promises in Python?(如何用Python编写一系列承诺?)
本文介绍了如何用Python编写一系列承诺?的处理方法,对大家解决问题具有一定的参考价值,需要的朋友们下面随着小编来一起学习吧!
问题描述
是否可以仅使用Python 3.6.1 Standard Library编写promise(或任务)序列?
例如,JavaScript中的序列承诺写成:
const SLEEP_INTERVAL_IN_MILLISECONDS = 200;
const alpha = function alpha (number) {
return new Promise(function (resolve, reject) {
const fulfill = function() {
return resolve(number + 1);
};
return setTimeout(fulfill, SLEEP_INTERVAL_IN_MILLISECONDS);
});
};
const bravo = function bravo (number) {
return new Promise(function (resolve, reject) {
const fulfill = function() {
return resolve(Math.ceil(1000*Math.random()) + number);
};
return setTimeout(fulfill, SLEEP_INTERVAL_IN_MILLISECONDS);
});
};
const charlie = function charlie (number) {
return new Promise(function (resolve, reject) {
return (number%2 == 0) ? reject(number) : resolve(number);
});
};
function run() {
return Promise.resolve(42)
.then(alpha)
.then(bravo)
.then(charlie)
.then((number) => {
console.log('success: ' + number)
})
.catch((error) => {
console.log('error: ' + error);
});
}
run();
具有异步处理结果的每个函数also returns a Promise,将由紧随其后的承诺解决/拒绝。
我知道promises-2.01b
和asyncio 3.4.3
这样的库,我正在寻找Python STL解决方案。因此,如果需要导入非STL库,我更喜欢使用RxPython。
推荐答案
下面是一个使用Asyncio和async/await
语法的类似程序:
import asyncio
import random
async def alpha(x):
await asyncio.sleep(0.2)
return x + 1
async def bravo(x):
await asyncio.sleep(0.2)
return random.randint(0, 1000) + x
async def charlie(x):
if x % 2 == 0:
return x
raise ValueError(x, 'is odd')
async def run():
try:
number = await charlie(await bravo(await alpha(42)))
except ValueError as exc:
print('error:', exc.args[0])
else:
print('success:', number)
if __name__ == '__main__':
loop = asyncio.get_event_loop()
loop.run_until_complete(run())
loop.close()
编辑:如果您对反应流感兴趣,可以考虑使用aiostream。
这里有一个简单的示例:
import asyncio
from aiostream import stream, pipe
async def main():
# This stream computes 11² + 13² in 1.5 second
xs = (
stream.count(interval=0.1) # Count from zero every 0.1 s
| pipe.skip(10) # Skip the first 10 numbers
| pipe.take(5) # Take the following 5
| pipe.filter(lambda x: x % 2) # Keep odd numbers
| pipe.map(lambda x: x ** 2) # Square the results
| pipe.accumulate() # Add the numbers together
)
print('11² + 13² = ', await xs)
if __name__ == '__main__':
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
loop.close()
documentation中有更多示例。
免责声明:我是项目维护者。
这篇关于如何用Python编写一系列承诺?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
沃梦达教程
本文标题为:如何用Python编写一系列承诺?
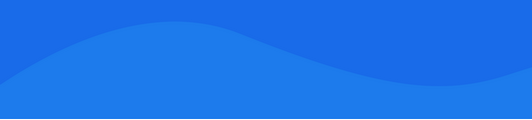
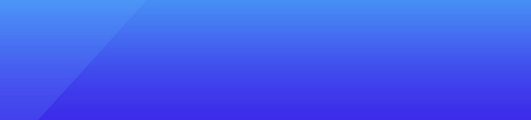
基础教程推荐
猜你喜欢
- 动态更新多个选择框 2022-01-01
- 在for循环中使用setTimeout 2022-01-01
- 我什么时候应该在导入时使用方括号 2022-01-01
- 悬停时滑动输入并停留几秒钟 2022-01-01
- Karma-Jasmine:如何正确监视 Modal? 2022-01-01
- 有没有办法使用OpenLayers更改OpenStreetMap中某些要素 2022-09-06
- 响应更改 div 大小保持纵横比 2022-01-01
- 角度Apollo设置WatchQuery结果为可用变量 2022-01-01
- 当用户滚动离开时如何暂停 youtube 嵌入 2022-01-01
- 在 JS 中获取客户端时区(不是 GMT 偏移量) 2022-01-01