How to force child same virtual function call its parent virtual function first(如何强制子同一个虚函数先调用其父虚函数)
问题描述
伙计们,我有一个案例,需要子类在调用它的覆盖虚函数之前首先调用它的父虚函数.
guys, I have a case that needs the child class needs to call its parent virtual function at first before call its override virtual function.
BaseClass::Draw()
{
}
ChildClass::Draw()
{
BaseClass::Draw(); // BaseClass Draw must be called first.
}
GrandChildClass::Draw()
{
ChildClass::Draw(); // ChildClass Draw must be called first.
}
我想对客户隐藏这种行为.这上面有规律吗?
I want to hide this behavior from clients. Is there pattern on this?
谢谢.
推荐答案
对于简单的情况,您可以使用第二个私有成员函数来实现可覆盖的行为:
For simple cases you can use a second, private member function for the overrideable behavior:
class Base {
public:
void Draw() {
// Base-class functionality here
OverrideableDraw();
}
private:
virtual void OverrideableDraw() { }
};
class Derived : public Base {
private:
virtual void OverrideableDraw() {
// Derived-class functionality here
}
};
<小时>
对于更复杂的层次结构(例如,您有多个继承级别),这是不可能的,因为任何派生类都可以覆盖任何虚拟成员函数(C++ 中没有 final
).通常可以安全地假设每个派生类都在做正确的事情.虽然我能想到有几次因为派生类搞砸了重写而遇到了问题,但这些情况通常很容易调试.
For more complex hierarchies (e.g. where you have multiple levels of inheritance), this isn't possible since any derived class can override any virtual member function (there is no final
in C++). Usually it is safe to assume that each derived class is doing the right thing. While I can think of a few times that I've run into issues because a derived class screwed up overriding, those cases were usually pretty straightforward to debug.
如果你真的很担心并且真的想保证首先执行基类覆盖,你可以使用这样的东西,尽管这非常昂贵(至少这种幼稚的实现非常昂贵):
If you are really worried about it and really want to guarantee that base-class overrides are executed first, you could use something like this, though this is quite expensive (at least this naive implementation is quite expensive):
#include <functional>
#include <iostream>
#include <vector>
class Base {
public:
Base() {
RegisterDrawCallback(std::bind(&Base::DrawCallback, this));
}
void Draw() {
for (auto it(drawCallbacks_.begin()); it != drawCallbacks_.end(); ++it)
(*it)();
}
protected:
typedef std::function<void(void)> DrawCallbackType;
typedef std::vector<DrawCallbackType> DrawSequence;
void RegisterDrawCallback(DrawCallbackType f) {
drawCallbacks_.push_back(f);
}
private:
void DrawCallback() { std::cout << "Base" << std::endl; }
DrawSequence drawCallbacks_;
};
class Derived : public Base {
public:
Derived() {
RegisterDrawCallback(std::bind(&Derived::DrawCallback, this));
}
private:
void DrawCallback() { std::cout << "Derived" << std::endl; }
};
class DerivedDerived : public Derived {
public:
DerivedDerived() {
RegisterDrawCallback(std::bind(&DerivedDerived::DrawCallback, this));
}
private:
void DrawCallback() { std::cout << "DerivedDerived" << std::endl; }
};
[这只是一种选择;其他人可能会想出一个更优雅的解决方案.就我个人而言,我只是确保虚拟成员函数有良好的文档记录并保留它.]
[This is just one option; someone else can probably come up with a far more elegant solution. Personally, I'd just make sure the virtual member functions are well-documented and leave it at that.]
这篇关于如何强制子同一个虚函数先调用其父虚函数的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何强制子同一个虚函数先调用其父虚函数
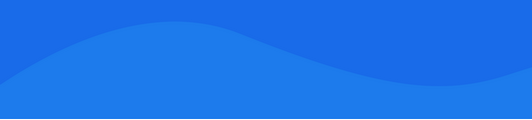
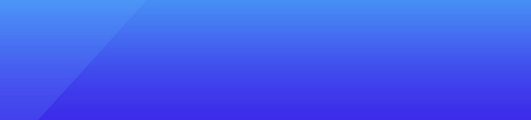
基础教程推荐
- C语言访问数组元素 1970-01-01
- C++定义类对象 1970-01-01
- 初始化变量和赋值运算符 1970-01-01
- C++ #define 1970-01-01
- 明确指定任何或所有枚举数的整数值 1970-01-01
- end() 能否成为 stl 容器的昂贵操作 2022-10-23
- C++按值调用 1970-01-01
- 分别使用%o和%x以八进制或十六进制格式显示整 1970-01-01
- 使用scanf()读取字符串 1970-01-01
- C++输入/输出运算符重载 1970-01-01