Generic function to flatten a container of containers(扁平化容器容器的通用函数)
问题描述
我试图更好地掌握迭代器和泛型函数.我认为编写一个转换 container1 < 的函数将是一个有用的练习.容器2<类型>>
到 container3 <type>
.例如,它应该能够转换 vector<;双端队列<int>>
到 list<int>
.
I am trying to get a better hold on iterators and generic functions. I thought it would be a useful exercise to write a function that converts container1 < container2 <type> >
to container3 <type>
. For example, it should be able to convert vector< deque<int> >
to list<int>
.
我认为所有容器访问都应该通过迭代器,就像 <algorithm>
中的函数一样.
I figured all the container access should be through iterators, like the functions in <algorithm>
.
这是我的代码:
#include <iterator>
#include <algorithm>
// COCiter == Container of Containers Iterator
// Oiter == Output Iterator
template <class COCiter, class Oiter>
void flatten (COCiter start, COCiter end, Oiter dest)
{
using namespace std;
while (start != end) {
dest = copy(start->begin(), start()->end(), dest);
++start;
}
}
但是当我尝试在下面的代码中调用它时:
But when I try to call it in the following code:
int main ()
{
using namespace std;
vector< vector<string> > splitlines;
vector<string> flat;
/* some code to fill SPLITLINES with vectors of strings */
flatten(splitlines.begin(), splitlines.end(), back_inserter(flat));
}
我收到一条巨大的 C++ 模板错误消息,undefined reference to void flatten<...模板页面...
I get a huge C++ template error message, undefined reference to void flatten< ... pages of templates ...
我觉得我的代码写得太容易了,我必须需要更多的东西来确保内部容器中的数据类型与输出容器中的数据类型匹配.但我不知道该怎么办.
I feel like my code was too easy to write, and I must need some more stuff to ensure that the data type in the inner containers matches the data type in the output container. But I don't know what to do.
推荐答案
我发现了问题.感谢 SFINAE(替换失败不是错误),您的编译器找不到正确的模板,因为您试图通过键入 在
(可能是错字).试试这个:start
上调用 operator()
start()
I found the issue. Thanks to SFINAE (Substitution failure is not an error) your compiler couldn't find the correct template because you are trying to call operator()
on start
by typing start()
(probably a typo). Try this:
#include <iterator>
#include <algorithm>
// COCiter == Container of Containers Iterator
// Oiter == Output Iterator
template <class COCiter, class Oiter>
void flatten (COCiter start, COCiter end, Oiter dest) {
while (start != end) {
dest = std::copy(start->begin(), start->end(), dest);
++start;
}
}
这篇关于扁平化容器容器的通用函数的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:扁平化容器容器的通用函数
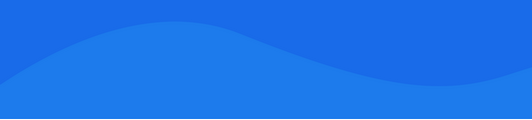
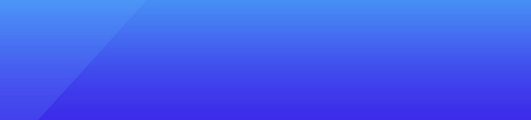
基础教程推荐
- 分别使用%o和%x以八进制或十六进制格式显示整 1970-01-01
- C++输入/输出运算符重载 1970-01-01
- C++定义类对象 1970-01-01
- C语言访问数组元素 1970-01-01
- C++按值调用 1970-01-01
- end() 能否成为 stl 容器的昂贵操作 2022-10-23
- 使用scanf()读取字符串 1970-01-01
- 初始化变量和赋值运算符 1970-01-01
- 明确指定任何或所有枚举数的整数值 1970-01-01
- C++ #define 1970-01-01