Meaning of #39;const#39; last in a function declaration of a class?(类的函数声明中最后一个“const的含义?)
问题描述
const
在这样的声明中是什么意思?const
让我很困惑.
What is the meaning of const
in declarations like these? The const
confuses me.
class foobar
{
public:
operator int () const;
const char* foo() const;
};
推荐答案
当你将 const
关键字添加到方法时,this
指针本质上将变成指向 const
对象,因此您不能更改任何成员数据.(除非你使用 mutable
,稍后会详细介绍).
When you add the const
keyword to a method the this
pointer will essentially become a pointer to const
object, and you cannot therefore change any member data. (Unless you use mutable
, more on that later).
const
关键字是函数签名的一部分,这意味着您可以实现两种类似的方法,一种在对象为 const
时调用,另一种在对象为 const
时调用.不.
The const
keyword is part of the functions signature which means that you can implement two similar methods, one which is called when the object is const
, and one that isn't.
#include <iostream>
class MyClass
{
private:
int counter;
public:
void Foo()
{
std::cout << "Foo" << std::endl;
}
void Foo() const
{
std::cout << "Foo const" << std::endl;
}
};
int main()
{
MyClass cc;
const MyClass& ccc = cc;
cc.Foo();
ccc.Foo();
}
这将输出
Foo
Foo const
在非常量方法中,您可以更改实例成员,而在 const
版本中则无法做到这一点.如果你把上面例子中的方法声明改成下面的代码,你会得到一些错误.
In the non-const method you can change the instance members, which you cannot do in the const
version. If you change the method declaration in the above example to the code below you will get some errors.
void Foo()
{
counter++; //this works
std::cout << "Foo" << std::endl;
}
void Foo() const
{
counter++; //this will not compile
std::cout << "Foo const" << std::endl;
}
这并不完全正确,因为您可以将成员标记为 mutable
,然后 const
方法可以更改它.它主要用于内部计数器和东西.解决方案是下面的代码.
This is not completely true, because you can mark a member as mutable
and a const
method can then change it. It's mostly used for internal counters and stuff. The solution for that would be the below code.
#include <iostream>
class MyClass
{
private:
mutable int counter;
public:
MyClass() : counter(0) {}
void Foo()
{
counter++;
std::cout << "Foo" << std::endl;
}
void Foo() const
{
counter++; // This works because counter is `mutable`
std::cout << "Foo const" << std::endl;
}
int GetInvocations() const
{
return counter;
}
};
int main(void)
{
MyClass cc;
const MyClass& ccc = cc;
cc.Foo();
ccc.Foo();
std::cout << "Foo has been invoked " << ccc.GetInvocations() << " times" << std::endl;
}
哪个会输出
Foo
Foo const
Foo has been invoked 2 times
这篇关于类的函数声明中最后一个“const"的含义?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:类的函数声明中最后一个“const"的含义?
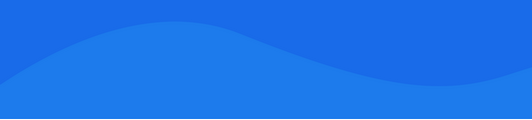
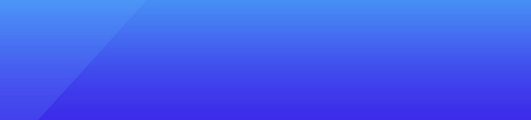
基础教程推荐
- C++使用easyX库实现三星环绕效果流程详解 2023-06-26
- C利用语言实现数据结构之队列 2022-11-22
- C语言 structural body结构体详解用法 2022-12-06
- 一文带你了解C++中的字符替换方法 2023-07-20
- C++中的atoi 函数简介 2023-01-05
- C/C++编程中const的使用详解 2023-03-26
- C++详细实现完整图书管理功能 2023-04-04
- 详解c# Emit技术 2023-03-25
- C语言基础全局变量与局部变量教程详解 2022-12-31
- 如何C++使用模板特化功能 2023-03-05