Undefined reference to #39;operator delete(void*)#39;(对“操作员删除(void *)的未定义引用)
问题描述
I'm new to C++ programming, but have been working in C and Java for a long time. I'm trying to do an interface-like hierarchy in some serial protocol I'm working on, and keep getting the error:
Undefined reference to 'operator delete(void*)'
The (simplified) code follows below:
PacketWriter.h:
class PacketWriter {
public:
virtual ~PacketWriter() {}
virtual uint8_t nextByte() = 0;
}
StringWriter.h:
class StringWriter : public PacketWriter {
public:
StringWriter(const char* message);
virtual uint8_t nextByte();
}
The constructor and nextByte functions are implemented in StringWriter.cpp, but nothing else. I need to be able to delete a StringWriter from a pointer to a PacketWriter, and i've been getting various other similar errors if I define a destructor for StringWriter, virtual or not. I'm sure it's a simple issue that I'm overlooking as a newbie.
Also, I'm writing this for an AVR chip, using avr-g++ on Windows.
Thanks
If you are not linking against the standard library for some reason (as may well be the case in an embedded scenario), you have to provide your own operators new
and delete
. In the simplest case, you could simply wrap malloc
, or allocate memory from your own favourite source:
void * operator new(std::size_t n)
{
void * const p = std::malloc(n);
// handle p == 0
return p;
}
void operator delete(void * p) // or delete(void *, std::size_t)
{
std::free(p);
}
You should never have to do this if you are compiling for an ordinary, hosted platform, so if you do need to do this, you better be familiar with the intricacies of memory management on your platform.
这篇关于对“操作员删除(void *)"的未定义引用的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:对“操作员删除(void *)"的未定义引用
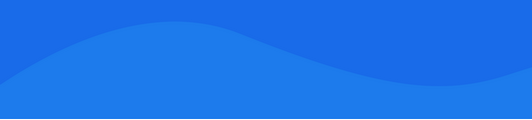
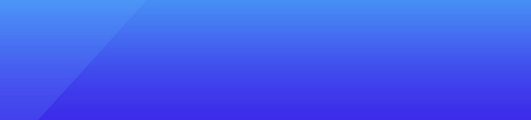
基础教程推荐
- C语言基础全局变量与局部变量教程详解 2022-12-31
- 一文带你了解C++中的字符替换方法 2023-07-20
- C语言 structural body结构体详解用法 2022-12-06
- C/C++编程中const的使用详解 2023-03-26
- 详解c# Emit技术 2023-03-25
- 如何C++使用模板特化功能 2023-03-05
- C利用语言实现数据结构之队列 2022-11-22
- C++详细实现完整图书管理功能 2023-04-04
- C++使用easyX库实现三星环绕效果流程详解 2023-06-26
- C++中的atoi 函数简介 2023-01-05