运算符重载的方法是定义一个重载运算符的函数,在需要执行被重载的运算符时,系统就自动调用该函数,以实现相应的运算。也就是说,运算符重载是通过定义函数实现的
运算符重载
运算符重载概念:对已有的运算符重新进行定义,赋予其另一种功能,以适应不同的数据类型
加号运算符重载
作用:实现两个自定义数据类型相加的运算
#include <iostream>
using namespace std;
class Person {
public:
// 构造函数
Person(int num1, int num2){
this->num1 = num1;
this->num2 = num2;
}
// 加法函数
Person operator+(const Person &p){
Person temp(0, 0);
temp.num1 = this->num1 + p.num1;
temp.num2 = this->num2 + p.num2;
return temp;
}
// 打印输出
void printMessage() {
cout << "num1 = " << num1 << " num2 = " << num2 << endl;
}
private:
int num1;
int num2;
};
void func() {
Person p1(1, 2);
Person p2(3, 4);
Person p3 = p1.operator+(p2); // 可以简化为 Person = p1 + p2;
p3.printMessage(); // num1 = 4 num2 = 6
Person p4 = p3 + p2;
p4.printMessage(); // num1 = 7 num2 = 10
}
int main() {
func();
system("pause");
return 0;
}
左移运算符重载
作用:输出自定义数据类型
第一种:成员函数(对象 << cout)
#include <iostream>
using namespace std;
class Person {
public:
// 构造函数
Person(int num1, int num2){
this->num1 = num1;
this->num2 = num2;
}
// 左移运算符 p.operator<<(cout); 简化版本 p << cout
void operator <<(ostream &cout) {
cout << "num1 = " << num1 << " num2 = " << num2 << endl;
}
private:
int num1;
int num2;
};
void func() {
Person p1(1, 2);
p1.operator<<(cout); // 简化为 p1 << cout
Person p2(3, 4);
p2 << cout;
}
int main() {
func();
// outputs:num1 = 1 num2 = 2
// num1 = 3 num2 = 4
system("pause");
return 0;
}
第二种:全局函数(cout << 对象)
#include <iostream>
using namespace std;
class Person {
// 友元
friend ostream& operator <<(ostream &cout, Person &p);
public:
// 构造函数
Person(int num1, int num2){
this->num1 = num1;
this->num2 = num2;
}
private:
int num1;
int num2;
};
// 左移运算符 p.operator<<(cout); 简化版本 p << cout
ostream& operator <<(ostream &cout, Person &p) {
cout << "num1 = " << p.num1 << " num2 = " << p.num2;
return cout;
}
void func() {
Person p1(1, 2);
operator<<(cout, p1); // 简化为 cout << p1;
cout << endl;
cout << p1 << " 你是猪!" << endl;
}
int main() {
func();
// outputs:num1 = 1 num2 = 2
// num1 = 1 num2 = 2 你是猪!
system("pause");
return 0;
}
递增运算符重载
通过重载递增运算符,实现自己的整型数据
#include <iostream>
using namespace std;
class MyInteger {
//friend ostream& operator <<(ostream &cout, MyInteger &p);
friend ostream& operator <<(ostream &cout, MyInteger p);
public:
// 构造函数
MyInteger(){
this->m_num = 0;
}
// 重载前置递增运算符
MyInteger& operator++() {
++this->m_num;
return *this;
}
// 重载后置递增运算符
MyInteger operator++(int) { // int 是占位参数,用于区分前置和后置递增
MyInteger temp = *this;
++this->m_num;
return temp;
}
private:
int m_num;
};
// 用于前置递增,左移运算符 p.operator<<(cout); 简化版本 p << cout
//ostream& operator <<(ostream &cout, MyInteger &p) {
// cout << "m_num = " << p.m_num;
// return cout;
/
沃梦达教程
本文标题为:C++类与对象之运算符重载详解
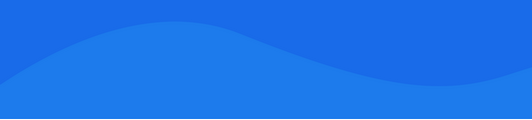
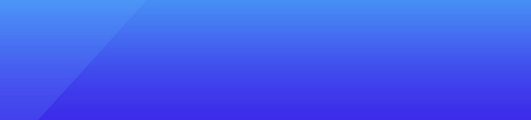
基础教程推荐
猜你喜欢
- C利用语言实现数据结构之队列 2022-11-22
- C++详细实现完整图书管理功能 2023-04-04
- C++使用easyX库实现三星环绕效果流程详解 2023-06-26
- C/C++编程中const的使用详解 2023-03-26
- 如何C++使用模板特化功能 2023-03-05
- C语言 structural body结构体详解用法 2022-12-06
- 详解c# Emit技术 2023-03-25
- 一文带你了解C++中的字符替换方法 2023-07-20
- C++中的atoi 函数简介 2023-01-05
- C语言基础全局变量与局部变量教程详解 2022-12-31