这篇文章主要为大家详细介绍了unity实现简单计算器,文中示例代码介绍的非常详细,具有一定的参考价值,感兴趣的小伙伴们可以参考一下
本文实例为大家分享了unity实现简单计算器的具体代码,供大家参考,具体内容如下
using System.Text;
using UnityEngine;
using UnityEngine.UI;
using DG.Tweening;
using System;
public class Calculator : MonoBehaviour
{
public Text SpendText;
private StringBuilder spendPrice;//初始金额
private string rmbSymbol;
private float totalPrice, spendPrices;//总和,初始金额
private bool isFirstDecrease;//避免减为零后的第二次起不能为负
private bool? isPlusOrDecrease, countType;//点击加减符号,点击等号
public Button PointButton;
private int count;//限制最大输入数
private void Start()
{
spendPrice = new StringBuilder();
totalPrice = 0;
spendPrices = 0;
rmbSymbol = "<size='50'>¥</size> ";
isPlusOrDecrease = null;//true为加,false为减
countType = null;//true为加,false为减
isFirstDecrease = true;
count = 0;
}
public void PointButtonController(bool type)
{
PointButton.interactable = type;
}
public void InputNumber(int num)
{
//按钮
switch (num)
{
case 0:
if (count < 11)
{
count++;
spendPrice.Append("0");
SpendText.DOText(rmbSymbol + spendPrice.ToString(), 0.1f);
}
break;
case 1:
if (count < 11)
{
count++;
spendPrice.Append("1");
SpendText.DOText(rmbSymbol + spendPrice.ToString(), 0.1f);
}
break;
case 2:
if (count < 11)
{
count++;
spendPrice.Append("2");
SpendText.DOText(rmbSymbol + spendPrice.ToString(), 0.1f);
}
break;
case 3:
if (count < 11)
{
count++;
spendPrice.Append("3");
SpendText.DOText(rmbSymbol + spendPrice.ToString(), 0.1f);
}
break;
case 4:
if (count < 11)
{
count++;
spendPrice.Append("4");
SpendText.DOText(rmbSymbol + spendPrice.ToString(), 0.1f);
}
break;
case 5:
if (count < 11)
{
count++;
spendPrice.Append("5");
SpendText.DOText(rmbSymbol + spendPrice.ToString(), 0.1f);
}
break;
case 6:
if (count < 11)
{
count++;
spendPrice.Append("6");
SpendText.DOText(rmbSymbol + spendPrice.ToString(), 0.1f);
}
break;
case 7:
if (count < 11)
{
count++;
spendPrice.Append("7");
SpendText.DOText(rmbSymbol + spendPrice.ToString(), 0.1f);
}
break;
case 8:
if (count < 11)
{
count++;
spendPrice.Append("8");
SpendText.DOText(rmbSymbol + spendPrice.ToString(), 0.1f);
}
break;
case 9:
if (count < 11)
{
count++;
spendPrice.Append("9");
SpendText.DOText(rmbSymbol + spendPrice.ToString(), 0.1f);
}
break;
case 10:
if (count < 11)
{
count += 2;
spendPrice.Append("00");
SpendText.DOText(rmbSymbol + spendPrice.ToString(), 0.1f);
}
break;
case 11://加
isPlusOrDecrease = true;
countType = true;
count = 0;
if (!spendPrice.ToString().Equals(""))
{
if ((totalPrice + float.Parse(spendPrice.ToString()) < 99999999999) && !totalPrice.ToString().Contains("E"))
{
PointButtonController(true);
if (totalPrice != 0)//避免第一次点击加号时没反应
{
TotalCount();
}
CountNum();
}
else
{
count = 0;
PointButtonController(true);
totalPrice = 0;
spendPrice.Clear();
SpendText.DOText(rmbSymbol, 0);
isFirstDecrease = true;
}
}
break;
case 12://减
isPlusOrDecrease = false;
countType = false;
count = 0;
if (!spendPrice.ToString().Equals(""))
{
if ((totalPrice + float.Parse(spendPrice.ToString()) < 99999999999) && !totalPrice.ToString().Contains("E"))
{
PointButtonController(true);
if (totalPrice != 0)//避免第一次点击减号时没反应
{
TotalCount();
}
CountNum();
}
else
{
count = 0;
PointButtonController(true);
totalPrice = 0;
spendPrice.Clear();
SpendText.DOText(rmbSymbol, 0);
isFirstDecrease = true;
}
}
break;
case 13://点
PointButtonController(false);
spendPrice.Append(".");
SpendText.DOText(rmbSymbol + spendPrice.ToString(), 0.1f);
break;
case 14://等号
count = 0;
if (!spendPrice.ToString().Equals(""))
{
if ((totalPrice + float.Parse(spendPrice.ToString()) < 9999999999) && !totalPrice.ToString().Contains("E"))
{
PointButtonController(true);
TotalCount();
}
else
{
count = 0;
PointButtonController(true);
totalPrice = 0;
spendPrice.Clear();
SpendText.DOText(rmbSymbol, 0);
isFirstDecrease = true;
}
}
break;
case 15://清零
count = 0;
PointButtonController(true);
totalPrice = 0;
spendPrice.Clear();
SpendText.DOText(rmbSymbol, 0);
isFirstDecrease = true;
break;
default:
break;
}
}
public void CountNum()
{
if (spendPrice.ToString().StartsWith("0") || spendPrice.ToString().Equals(""))//去除开始的无效零
{
if (spendPrice.ToString().TrimStart('0') == "" || spendPrice.ToString().TrimStart('0').TrimEnd('0') == ".")//0000,00.00,0.,.0
{
spendPrices = 0;
}
else
{
spendPrices = float.Parse((float.Parse(spendPrice.ToString().TrimStart('0'))).ToString("f2"));
}
}
else
{
spendPrices = float.Parse((float.Parse(spendPrice.ToString())).ToString("f2"));
}
if (isPlusOrDecrease == true && totalPrice != 0 && spendPrices != 0)
{
totalPrice += spendPrices;
spendPrice.Clear();
SpendText.DOText(rmbSymbol + totalPrice.ToString(), 0.1f);
isPlusOrDecrease = null;
}
else if (isPlusOrDecrease == true && totalPrice == 0 && spendPrices != 0 && spendPrices != 0)
{
totalPrice = spendPrices;
spendPrice.Clear();
}
if (isPlusOrDecrease == false && totalPrice == 0 && spendPrices != 0 && isFirstDecrease)
{
totalPrice = spendPrices;
spendPrice.Clear();
isFirstDecrease = false;
}
else if (isPlusOrDecrease == false && spendPrices != 0)
{
totalPrice -= spendPrices;
spendPrice.Clear();
SpendText.DOText(rmbSymbol + totalPrice.ToString(), 0.1f);
isPlusOrDecrease = null;
}
}
public void TotalCount()
{
if (spendPrice.ToString().StartsWith("0") || spendPrice.ToString().Equals(""))
{
if (spendPrice.ToString().TrimStart('0') == "" || spendPrice.ToString().TrimStart('0').TrimEnd('0') == ".")
{
spendPrices = 0;
}
else
{
spendPrices = float.Parse((float.Parse(spendPrice.ToString().TrimStart('0'))).ToString("f2"));
}
}
else
{
spendPrices = float.Parse((float.Parse(spendPrice.ToString())).ToString("f2"));
}
if (spendPrices != 0)
{
if (countType == true)
{
totalPrice += spendPrices;
}
else if (countType == false)
{
totalPrice -= spendPrices;
}
spendPrice.Clear();
SpendText.DOText(rmbSymbol + totalPrice.ToString(), 0.1f);
countType = null;
}
}
//将科学计数法转化为普通数字
private Decimal ChangeDataToD(string strData)
{
Decimal dData = 0.0M;
if (strData.Contains("E"))
{
dData = Decimal.Parse(strData, System.Globalization.NumberStyles.Float);
}
return dData;
}
}
以上就是本文的全部内容,希望对大家的学习有所帮助,也希望大家多多支持得得之家。
沃梦达教程
本文标题为:unity实现简单计算器
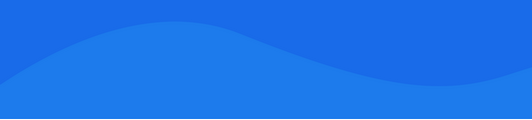
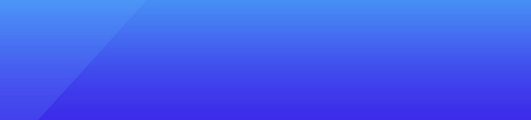
基础教程推荐
猜你喜欢
- 一个读写csv文件的C#类 2022-11-06
- C# windows语音识别与朗读实例 2023-04-27
- C#类和结构详解 2023-05-30
- C# 调用WebService的方法 2023-03-09
- winform把Office转成PDF文件 2023-06-14
- ZooKeeper的安装及部署教程 2023-01-22
- unity实现动态排行榜 2023-04-27
- C#控制台实现飞行棋小游戏 2023-04-22
- linux – 如何在Debian Jessie中安装dotnet core sdk 2023-09-26
- C# List实现行转列的通用方案 2022-11-02