本文详细讲解了C#客户端HttpClient请求认证及数据传输,文中通过示例代码介绍的非常详细。对大家的学习或工作具有一定的参考借鉴价值,需要的朋友可以参考下
一,授权认证
客户端请求服务器时,需要通过授权认证许可,方能获取服务器资源,目前比较常见的认证方式有 Basic 、JWT、Cookie。
HttpClient 是 C# 中的 HTTP/HTTPS 客户端,用于发送 HTTP 请求和接收来自通过 URI 确认的资源的 HTTP 响应。下面以具体代码做示范。
1. 基础认证示例
// Basic基础认证
public async Task Basic(string user, string password, string url)
{
// 如果认证页面是 https 的,请参考一下 jwt 认证的 HttpClientHandler
// 创建 client
HttpClient client = new HttpClient();
// 创建身份认证
// using System.Net.Http.Headers;
AuthenticationHeaderValue authentication = new AuthenticationHeaderValue(
"Basic",
Convert.ToBase64String(Encoding.UTF8.GetBytes($"{user}:{password}")
));
client.DefaultRequestHeaders.Authorization = authentication;
byte[] response = await client.GetByteArrayAsync(url);
client.Dispose();
}
可以看到 Basic 认证的安全程度非常低,多用于路由器和嵌入式设备,而且往往不会使用 HTTPS。
2. JWT 认证示例
// Jwt认证
public async Task Bearer(string token, string url)
{
// HttpClientHandler及其派生类使开发人员能够配置各种选项, 包括从代理到身份验证。
// helpLink https://docs.microsoft.com/en-us/dotnet/api/system.net.http.httpclienthandler?view=netframework-4.8
var httpclientHandler = new HttpClientHandler();
// 如果服务器有 https 证书,但是证书不安全,则需要使用下面语句
// => 也就是说,不校验证书,直接允许
httpclientHandler.ServerCertificateCustomValidationCallback = (message, cert, chain, error) => true;
using (var httpClient = new HttpClient(httpclientHandler))
{
// 创建身份认证
// System.Net.Http.Headers.AuthenticationHeaderValue;
httpClient.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", token);
await httpClient.GetAsync(url);
httpClient.Dispose();
}
}
JWT 认证,需要客户端携带 token ,token 是一段加密过的字符串,关于原理这里不多说,token 是通过客户端 header 携带的。
另外,对于测试的 Web 应用或者内网应用, HTTPS 证书可能不是公网国际认证的证书,就需要跳过认证,直接允许访问使用。
var httpclientHandler = new HttpClientHandler()
{
ServerCertificateCustomValidationCallback = (message, cert, chain, error) => true,
};
3. Cookie 示例
HttpClient 中,Cookie 有两种处理方式。
一种是已经知道 Cookie ,直接将 Cookie 存储到 HttpClient 中;另一种是还没有 Cookie ,通过账号密码登录获取到 Cookie ,自动存储到 HttpClient 对象中,接着使用当前 HttpClient 对象请求 URL。
两种方式的设定,是通过 HttpClientHandler 的 UseCookies 属性设置的。
示例
var httpclientHandler = new HttpClientHandler()
{
UseCookies = true
};
UseCookies 获取或设置一个值,该值指示处理程序是否使用 CookieContainer 属性存储服务器 Cookie,并在发送请求时使用这些 Cookie。
方式1:
// 先用账号密码登陆再请求
public async Task Cookie(string user, string password, string loginUrl, string url)
{
var httpclientHandler = new HttpClientHandler()
{
ServerCertificateCustomValidationCallback = (message, cert, chain, error) => true,
UseCookies = true
};
// 如果服务器有 https 证书,但是证书不安全,则需要使用下面语句
// => 也就是说,不校验证书,直接允许
var loginContent = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string,string>("user",user),
new KeyValuePair<string, string>("password",password)
});
using (var httpClient = new HttpClient(httpclientHandler))
{
// 先登陆
var result = await httpClient.PostAsync(loginUrl, loginContent);
// 登陆成功后,客户端会自动携带 cookie ,不需要再手动添加
//if (result.IsSuccessStatusCode)
//{
// /*
// * 如果请求成功
// */
/
本文标题为:C#客户端HttpClient请求认证及数据传输
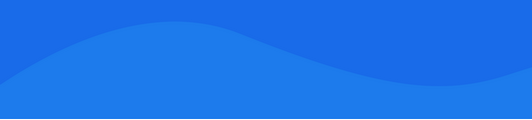
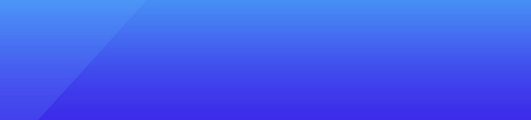
基础教程推荐
- 一个读写csv文件的C#类 2022-11-06
- C# 调用WebService的方法 2023-03-09
- C# windows语音识别与朗读实例 2023-04-27
- C# List实现行转列的通用方案 2022-11-02
- winform把Office转成PDF文件 2023-06-14
- C#类和结构详解 2023-05-30
- unity实现动态排行榜 2023-04-27
- ZooKeeper的安装及部署教程 2023-01-22
- C#控制台实现飞行棋小游戏 2023-04-22
- linux – 如何在Debian Jessie中安装dotnet core sdk 2023-09-26