这篇文章主要介绍了c#操作sql server2008 的界面实例代码,非常不错,具有参考借鉴价值,需要的朋友可以参考下
先是查询整张表,用到combobox选择查询哪张表,最后用DataGridView显示
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace WindowsFormsApplication2
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void dataGridView1_CellContentClick(object sender, DataGridViewCellEventArgs e)
{
}
private void Form1_Load(object sender, EventArgs e)
{
this.dataGridView1.RowHeadersVisible = false;
this.dataGridView1.AllowUserToAddRows = false;
this.dataGridView1.ReadOnly = true;
this.dataGridView1.SelectionMode = DataGridViewSelectionMode.FullRowSelect;
// this.comboBox1.SelectedIndex =0;
string sql = "select * from student";
DataTable table = SqlManage.TableSelect(sql);
this.dataGridView1.DataSource = table;
comboBox1.Items.Add("学生表");
comboBox1.Items.Add("教师表");
}
private void comboBox1_SelectedIndexChanged(object sender, EventArgs e)
{
string sql = "";
switch (this.comboBox1.SelectedIndex)
{
case 0:
sql = "select id as 学生号,name as 姓名,sage as 年龄 from student";
break;
case 1:
sql = "select t_id as 教师号,t_name as 姓名,T_age as 年龄 from teacher";
break;
default:
break;
}
DataTable table = SqlManage.TableSelect(sql);
this.dataGridView1.DataSource = table;
}
}
}
然后是修改表格,这个比较简单,用到textbox和button
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace WindowsFormsApplication2
{
public partial class Form2 : Form
{
public Form2()
{
InitializeComponent();
}
private void button4_Click(object sender, EventArgs e)
{
this.Close();
}
private void button1_Click(object sender, EventArgs e)
{
string sql = string.Format("insert into teacher values('{0}','{1}','{2}')",
this.textBox1.Text, this.textBox2.Text, this.textBox3.Text);
SqlManage.TableChange(sql);
}
private void button2_Click(object sender, EventArgs e)
{
string sql = string.Format("update teacher set ('{0}',''{1}'','{2}')",
this.textBox1.Text, this.textBox2.Text, this.textBox3.Text);
SqlManage.TableChange(sql);
}
private void button3_Click(object sender, EventArgs e)
{
string sql = string.Format("delete from teacher where t_id='{0}'",
this.textBox1.Text);
SqlManage.TableChange(sql);
}
private void Form2_Load(object sender, EventArgs e)
{
}
}
}
按条件查询表格,这个是核心,用到radiobutt,combobox,,button, DataGridView
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace WindowsFormsApplication2
{
public partial class Form3 : Form
{
public Form3()
{
InitializeComponent();
}
private void dataGridView1_CellContentClick(object sender, DataGridViewCellEventArgs e)
{
}
private void Form3_Load(object sender, EventArgs e)
{
this.comboBox1.Enabled = false;
this.comboBox2.Enabled = false;
this.comboBox3.Enabled = false;
this.comboBox4.Enabled = false;
//初始化教师编号
string sql = "select t_id from teacher";
DataTable table = SqlManage.TableSelect(sql);
string t_id;
foreach (DataRow row in table.Rows)
{
t_id = row["t_id"].ToString();
this.comboBox1.Items.Add(t_id);
}
if (table.Rows.Count > 0)
{
this.comboBox1.SelectedIndex = 0;
}
//初始化教师姓名
string sql_name = "select t_name from teacher";
table.Clear();
table = SqlManage.TableSelect(sql_name);
string t_name;
foreach (DataRow row in table.Rows)
{
t_name= row["t_name"].ToString();
this.comboBox2.Items.Add(t_name);
}
if (table.Rows.Count > 0)
{
this.comboBox2.SelectedIndex = 0;
}
//初始化学生
string sql_id = "select id from student";
table.Clear();
table = SqlManage.TableSelect(sql_id);
string s_id;
foreach (DataRow row in table.Rows)
{
s_id = row["id"].ToString();
this.comboBox3.Items.Add(s_id);
}
if (table.Rows.Count > 0)
{
this.comboBox3.SelectedIndex = 0;
}
//初始化学生
string sql_sname = "select name from student";
table.Clear();
table = SqlManage.TableSelect(sql_sname);
string t_sname;
foreach (DataRow row in table.Rows)
{
t_sname = row["name"].ToString();
this.comboBox4.Items.Add(t_sname);
}
if (table.Rows.Count > 0)
{
this.comboBox4.SelectedIndex = 0;
}
}
private void button2_Click(object sender, EventArgs e)
{
this.Close();
}
private void button1_Click(object sender, EventArgs e)
{
string sql = "";
if (this.radioButton1.Checked)
{
sql = string.Format("select t_id as 教师编号,t_name as 教师姓名,t_age as 年龄 from teacher where t_id = '{0}'",
this.comboBox1.Text);
}
else if (this.radioButton2.Checked)
{
sql = string.Format("select t_id as 教师编号,t_name as 教师姓名,t_age as 年龄 from teacher where t_name = '{0}'",
this.comboBox2.Text);
}
else if (this.radioButton3.Checked)
{
sql = string.Format("select id as 学生编号,name as 学生姓名,sage as 年龄 from student where id = '{0}'",
this.comboBox3.Text);
}
else if (this.radioButton4.Checked)
{
sql = string.Format("select id as 学生编号,name as 学生姓名,sage as 年龄 from student where name = '{0}'",
this.comboBox4.Text);
}
DataTable table = SqlManage.TableSelect(sql);
if (table.Rows.Count > 0)
{
this.dataGridView1.DataSource = table;
}
else
{
MessageBox.Show("没有相关内容");
}
}
private void radioButton1_CheckedChanged(object sender, EventArgs e)
{
if (this.radioButton1.Checked)
{
this.comboBox1.Enabled = true;
}
else
{
this.comboBox1.Enabled = false;
}
}
private void radioButton2_CheckedChanged(object sender, EventArgs e)
{
if (this.radioButton2.Checked)
{
this.comboBox2.Enabled = true;
}
else
{
this.comboBox2.Enabled = false;
}
}
private void radioButton3_CheckedChanged(object sender, EventArgs e)
{
if (this.radioButton3.Checked)
{
this.comboBox3.Enabled = true;
}
else
{
this.comboBox3.Enabled = false;
}
}
private void radioButton4_CheckedChanged(object sender, EventArgs e)
{
if (this.radioButton4.Checked)
{
this.comboBox4.Enabled = true;
}
else
{
this.comboBox4.Enabled = false;
}
}
}
}
以上所述是小编给大家介绍的c#操作sql server2008 的界面实例代码,希望对大家有所帮助,如果大家有任何疑问请给我留言,小编会及时回复大家的。在此也非常感谢大家对编程学习网网站的支持!
沃梦达教程
本文标题为:c#操作sql server2008 的界面实例代码
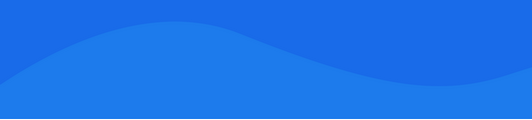
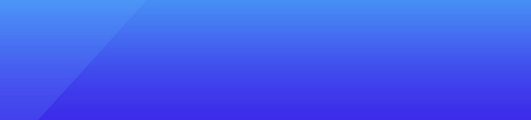
基础教程推荐
猜你喜欢
- C#类和结构详解 2023-05-30
- C#控制台实现飞行棋小游戏 2023-04-22
- winform把Office转成PDF文件 2023-06-14
- linux – 如何在Debian Jessie中安装dotnet core sdk 2023-09-26
- unity实现动态排行榜 2023-04-27
- C# 调用WebService的方法 2023-03-09
- ZooKeeper的安装及部署教程 2023-01-22
- C# List实现行转列的通用方案 2022-11-02
- 一个读写csv文件的C#类 2022-11-06
- C# windows语音识别与朗读实例 2023-04-27