这篇文章主要为大家详细介绍了C#实现餐饮管理系统,具有一定的参考价值,感兴趣的小伙伴们可以参考一下
本文实例为大家分享了C#实现餐饮管理系统的具体代码,供大家参考,具体内容如下
此系统采用C#语言的Winfrom和ADO.NET技术搭建的简单的CS系统。
部分代码:
frmBook.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using DAL;
namespace Catering
{
public partial class frmBook : Form
{
public frmBook()
{
InitializeComponent();
}
public void getData()
{
string Filter = " WHERE 1 = 1 ";
if (txtName.Text.Trim() != "")
{
Filter += " AND Name Like '%" + txtName.Text + "%'";
}
BookEntity book = new BookEntity();
DataTable dt = book.Query(Filter);
this.dataGridView1.DataSource = dt;
for (int i = 1; i < this.dataGridView1.Columns.Count; i++)
{
this.dataGridView1.Columns[i].ReadOnly = true;
}
}
private void frmBook_Load(object sender, EventArgs e)
{
getData();
}
//全选
private void btnChose_Click(object sender, EventArgs e)
{
bool b = false;
if (btnChose.Text == "全 选")
{
b = true;
btnChose.Text = "取消全选";
}
else
{
b = false;
btnChose.Text = "全 选";
}
for (int i = 0; i < this.dataGridView1.Rows.Count; i++)
{
dataGridView1.Rows[i].Cells[0].Value = b;
}
this.dataGridView1.EndEdit();
this.dataGridView1.CurrentCell = null;
}
//删除
private void btnDelete_Click(object sender, EventArgs e)
{
dataGridView1.EndEdit();
dataGridView1.CurrentCell = null;
DataTable dt = (DataTable)this.dataGridView1.DataSource;
DataRow[] drs = dt.Select("选择=1");
if (drs.Length == 0)
{
MessageBox.Show("请选择要删除的记录!");
return;
}
foreach (DataRow dr in drs)
{
BookEntity book = new BookEntity();
book.Id = Convert.ToInt32(dr["编号"].ToString());
book.Delete();
}
MessageBox.Show("删除成功!");
getData();
}
//查询
private void btnSearch_Click(object sender, EventArgs e)
{
getData();
}
//双击修改
private void dataGridView1_MouseDoubleClick(object sender, MouseEventArgs e)
{
if (this.dataGridView1.CurrentRow.Index > -1)
{
frmBookEdit frm = new frmBookEdit();
frm.StartPosition = FormStartPosition.CenterScreen;
frm.IdNo = dataGridView1.CurrentRow.Cells[1].Value.ToString();
if (frm.ShowDialog() == DialogResult.OK)
{
getData();
}
}
}
}
}
frmControl.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using DAL;
namespace Catering
{
public partial class frmControl : Form
{
public frmControl()
{
InitializeComponent();
}
private void frmControl_Load(object sender, EventArgs e)
{
#region 生成餐桌信息
TableNoEntity table = new TableNoEntity();
DataTable dt = table.Query(" ORDER BY DispalyIndex ");
int x = 46;
int y = 66;
int width = 150;
int height = 95;
//动态生成餐台
for (int i = 0; i < dt.Rows.Count; i = i + 5)
{
for (int j = 0; j < 5 && (i + j) < dt.Rows.Count; j++)
{
DataRow dr = dt.Rows[i + j];
//生成餐台图片控件
PictureBox pictureBox = new PictureBox();
pictureBox.ContextMenuStrip = this.contextMenuStrip1;
pictureBox.Image = Image.FromFile(Application.StartupPath + "\\res\\绿.gif");
//判断是否有预定
BookEntity book = new BookEntity();
DataTable dat = book.Query(" where TableNo ='" + dr["TableNo"] + "' AND BookTime>getdate()");
if (dat.Rows.Count > 0)
{
pictureBox.Image = Image.FromFile(Application.StartupPath + "\\res\\黄.gif");
}
//判断是否在使用
OrdersEntity orders = new OrdersEntity();
DataTable dats = orders.Query(" where TableNo ='" + dr["TableNo"] + "' and PayORnot ='否'");
if (dats.Rows.Count > 0)
{
pictureBox.Image = Image.FromFile(Application.StartupPath + "\\res\\红.gif");
}
pictureBox.Location = new System.Drawing.Point(x, y);
pictureBox.Name = "pictureBox_" + dr["TableNo"].ToString();
pictureBox.Size = new System.Drawing.Size(width, height);
pictureBox.TabStop = false;
pictureBox.Visible = true;
pictureBox.SendToBack();
this.Controls.Add(pictureBox);
//生成餐台说明信息
Label lbl = new Label();
lbl.Name = "lbl_" + dr["TableNo"].ToString();
lbl.Text = dr["TableNo"].ToString() + " " + dr["SitCount"].ToString() + "座位";
lbl.Font = new Font("宋体", 10);
lbl.BackColor = Color.Transparent;
lbl.Location = new Point(25, 30);
pictureBox.Controls.Add(lbl);
x = x + 200;
}
y = y + 150;
x = 46;
}
#endregion
}
private void 预定ToolStripMenuItem_Click(object sender, EventArgs e)
{
string Name = contextMenuStrip1.SourceControl.Name;
string[] str = Name.Split('_');
frmBookEdit frm = new frmBookEdit();
frm.StartPosition = FormStartPosition.CenterScreen;
frm.TopMost = true;
frm.Id = str[1];
if (frm.ShowDialog() == DialogResult.OK)
{
((PictureBox)contextMenuStrip1.SourceControl).Image = Image.FromFile(Application.StartupPath + "\\res\\黄.gif");
}
}
private void 结账ToolStripMenuItem_Click(object sender, EventArgs e)
{
string Name = contextMenuStrip1.SourceControl.Name;
string[] str = Name.Split('_');
frmPayEdit frm = new frmPayEdit();
frm.StartPosition = FormStartPosition.CenterScreen;
//frm.TopMost = true;
frm.Id = str[1];
if (frm.ShowDialog() == DialogResult.OK)
{
((PictureBox)contextMenuStrip1.SourceControl).Image = Image.FromFile(Application.StartupPath + "\\res\\绿.gif");
}
}
private void 退订ToolStripMenuItem_Click(object sender, EventArgs e)
{
}
private void 点菜ToolStripMenuItem1_Click(object sender, EventArgs e)
{
string Name = contextMenuStrip1.SourceControl.Name;
string[] str = Name.Split('_');
frmOrdersEdit frm = new frmOrdersEdit();
frm.StartPosition = FormStartPosition.CenterScreen;
frm.TopMost = true;
frm.Id = str[1];
if (frm.ShowDialog() == DialogResult.OK)
{
((PictureBox)contextMenuStrip1.SourceControl).Image = Image.FromFile(Application.StartupPath + "\\res\\红.gif");
}
}
private void btnRefesh_Click(object sender, EventArgs e)
{
}
}
}
源码下载:C#实现餐饮管理系统
以上就是本文的全部内容,希望对大家的学习有所帮助,也希望大家多多支持编程学习网。
沃梦达教程
本文标题为:C#实现餐饮管理系统
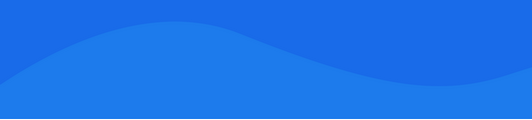
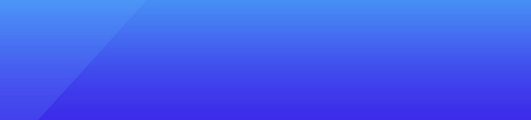
基础教程推荐
猜你喜欢
- winform把Office转成PDF文件 2023-06-14
- linux – 如何在Debian Jessie中安装dotnet core sdk 2023-09-26
- C# 调用WebService的方法 2023-03-09
- ZooKeeper的安装及部署教程 2023-01-22
- unity实现动态排行榜 2023-04-27
- C#控制台实现飞行棋小游戏 2023-04-22
- C# windows语音识别与朗读实例 2023-04-27
- C#类和结构详解 2023-05-30
- 一个读写csv文件的C#类 2022-11-06
- C# List实现行转列的通用方案 2022-11-02