这篇文章主要为大家详细介绍了UGUI实现卡片椭圆方向滚动效果,文中示例代码介绍的非常详细,具有一定的参考价值,感兴趣的小伙伴们可以参考一下
本文实例为大家分享了UGUI实现卡片椭圆方向滚动的具体代码,供大家参考,具体内容如下
搭建简单的场景
运行效果
卡片移动动画通过插件DoTween实现
控制脚本:
using UnityEngine;
using System.Collections;
using UnityEngine.UI;
using DG.Tweening;
public class CardMove : MonoBehaviour {
GameObject[] sprites;
int halfSize;
Vector2 screenCenterPos;
public float startAngle;//中间卡牌的角度
public float deltaAngle;//相邻卡牌的角度差值
public float moveSpeed;//移动动画的速度
public Vector3 center;//椭圆中心点
public float A = 1;//long axis
public float B = 1;//short axis
int cardcount;
// Use this for initialization
void Start () {
init ();
}
// Update is called once per frame
void Update () {
}
/// <summary>
/// 初始化卡牌显示位置
/// </summary>
void init(){
screenCenterPos = new Vector2 (Screen.width*0.5f,Screen.height*0.5f);
cardcount = transform.childCount;
halfSize = (cardcount - 1) / 2;
sprites=new GameObject[cardcount];
for (int i = 0; i < cardcount; i++) {
sprites [i] = transform.GetChild (i).gameObject;
setPosition (i,false);
setDeeps (i);
}
}
/// <summary>
/// 椭圆的半长轴为A,半短轴为B,计算椭圆上一点的位置
/// x=A*cos(angle),y=B*sin(angle)
/// </summary>
/// <param name="index">Index.</param>
/// <param name="userTweener">是否使用tween动画.</param>
void setPosition(int index,bool userTweener=true){
//计算每一张卡片在椭圆上相对中间卡牌的角度
float angle = 0;
if(index<halfSize){//left
angle=startAngle-(halfSize-index)*deltaAngle;
}else if(index>halfSize){//right
angle = startAngle + (index - halfSize) * deltaAngle;
}else{//medim
angle=startAngle;
}
//通过卡牌的角度,计算对应的位置
float xpos = A*Mathf.Cos((angle/180)*Mathf.PI);//+center.x;
float ypos = B*Mathf.Sin((angle/180)*Mathf.PI);//+center.y;
Debug.Log ("index="+index+",xpos="+xpos+",ypos="+ypos);
Vector2 pos = new Vector2 (xpos,ypos);
// Debug.Log ("screenPos="+screenPos+",wordPos="+wordPos);
//通过doTween控制卡片移动动画
if(!userTweener){
sprites [index].GetComponent<Image> ().rectTransform.DOMove(new Vector2(screenCenterPos.x+pos.x,screenCenterPos.y+pos.y),0f);
}else
sprites [index].GetComponent<Image> ().rectTransform.DOMove(new Vector2(screenCenterPos.x+pos.x,screenCenterPos.y+pos.y),1f);
}
/// <summary>
/// 计算每一张卡片的层级
/// </summary>
/// <param name="index">Index.</param>
void setDeeps(int index){
int deep = 0;
if (index < halfSize) {//左侧卡牌层级,从左侧到中间,层级依此递增
deep=index;
} else if (deep > halfSize) {//右侧卡牌层级,从中间到右侧,层级依此递减
deep=sprites.Length-(index+1);
} else {
deep = halfSize;
}
sprites [index].GetComponent<RectTransform> ().SetSiblingIndex (deep);
}
/// <summary>
/// 左侧按钮点击,向左移动
/// </summary>
public void OnLeftBtnClick(){
int length = sprites.Length;
GameObject temp=sprites[0];
for (int i = 0; i < length; i++) {//移动卡片在数组中的位置,依此向前移动一位
if (i == length - 1)
sprites [i] = temp;
else
sprites [i] = sprites [i + 1];
}
for (int i = 0; i < length; i++) {//跟新数组卡片需要显示的位置和层级
setPosition (i);
setDeeps (i);
}
}
/// <summary>
/// 右侧按钮点击,向右移动
/// </summary>
public void RightBtnClick(){
int length = sprites.Length;
GameObject temp=sprites[length-1];
for (int i = length-1; i >=0; i--) {
if (i == 0)
sprites [i] = temp;
else
sprites [i] = sprites [i - 1];
}
for (int i = 0; i < length; i++) {
setPosition (i);
setDeeps (i);
}
}
}
源码下载:地址
以上就是本文的全部内容,希望对大家的学习有所帮助,也希望大家多多支持编程学习网。
沃梦达教程
本文标题为:Unity UGUI实现卡片椭圆方向滚动
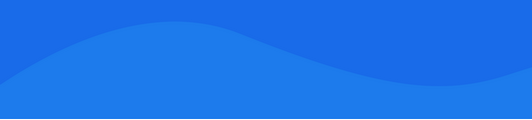
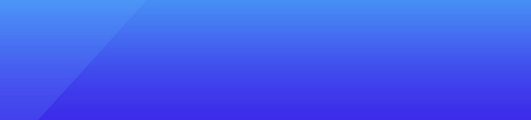
基础教程推荐
猜你喜欢
- unity实现动态排行榜 2023-04-27
- C# List实现行转列的通用方案 2022-11-02
- 一个读写csv文件的C#类 2022-11-06
- C# 调用WebService的方法 2023-03-09
- C#类和结构详解 2023-05-30
- ZooKeeper的安装及部署教程 2023-01-22
- linux – 如何在Debian Jessie中安装dotnet core sdk 2023-09-26
- C#控制台实现飞行棋小游戏 2023-04-22
- C# windows语音识别与朗读实例 2023-04-27
- winform把Office转成PDF文件 2023-06-14