这篇文章主要介绍了C#使用SqlConnection连接到SQL Server的代码示例,文中通过示例代码介绍的非常详细,对大家的学习或者工作具有一定的参考学习价值,需要的朋友们下面随着小编来一起学习学习吧
使用SqlConnection连接到SQL Server 2012
示例如下:
(1). 利用SqlConnection创建连接
public SQLServerAPI(string str_ip, string str_db, string str_user, string str_pwd)
{
m_strIp = str_ip;
m_strDb = str_db;
m_strUser = str_user;
m_strPwd = str_pwd;
//SQLServer身份验证
m_strConnection = @"Data Source=" + m_strIp;
m_strConnection += @";Initial Catalog=" + m_strDb;
m_strConnection += @";UID=" + m_strUser + ";PWD=" + m_strPwd;
m_strConnection += ";Connection Timeout=10;Pooling=true;Max Pool Size=100";
//Windows身份验证
//m_strConnection =
@"server=localhost\SQLEXPRESS;database=SQL2012Db;Trusted_Connection=SSPI;";
DisConnect();
m_Transaction = null;
m_SqlConnection = new SqlConnection(m_strConnection);
}
(2). 调用Open方法,以建立与服务器的会话。
/// <summary>
/// 尝试连接数据库
/// </summary>
private bool Connect()
{
if (m_SqlConnection == null)
return false;
try
{
m_SqlConnection.Open();
}
catch (Exception e)
{
Debug.WriteLine(e.Message);
return false;
}
return true;
}
(3). 调用Close()方法终止会话
private bool DisConnect()
{
if (m_SqlConnection == null)
return true;
try
{
m_SqlConnection.Close();
}
catch (Exception e)
{
Debug.WriteLine(e.Message);
return false;
}
return true;
许多程序员都使连接一直处于打开状态,直到程序结束为止,这通常会浪费服务器资源。与这种打开一次,永不关闭的方式相比,使用连接池,在需要时打开和关闭连接要更加高效。
如下所示,我们封装一个执行SQL存储过程的函数:
/// <summary>
/// 执行返回查询结果的存储过程
/// </summary>
/// <param name="procname">存储过程名?</param>
/// <param name="param">参数。函数正常返回时,所有类型为out的参数值也在对应位置上</param>
/// <param name="result">返回查询的结果</param>
/// <returns>0正确,其他错误</returns>
public int ExecQueryStoreProc(string procname, ref SqlParameter[] param, out DataTable result)
{
if (!Connect())
{
result = null;
return -1;
}
try
{
SqlCommand command = new SqlCommand(procname, m_SqlConnection);
command.CommandType = CommandType.StoredProcedure;
if (m_Transaction != null)
command.Transaction = m_Transaction;
SqlParameter rvalue = command.Parameters.Add(new SqlParameter("RETURN_VALUE", SqlDbType.Int));
rvalue.Direction = ParameterDirection.ReturnValue;
if (param != null)
command.Parameters.AddRange(param);
result = new DataTable();
SqlDataReader reader = command.ExecuteReader();
if (reader.HasRows)
result.Load(reader);
return Convert.ToInt32(command.Parameters["RETURN_VALUE"].Value);
}
catch (Exception)
{
result = null;
return -1;
}
finally
{
DisConnect();
}
}
上述过程就是在需要时打开和关闭连接的实现方式,另外finally块始终调用Close()方法,这并不会造成问题或者过多地浪费资源,而且能确保关闭连接。
以上所述是小编给大家介绍的SQL Server创建连接代码示例详解整合,希望对大家有所帮助,如果大家有任何疑问请给我留言,小编会及时回复大家的。在此也非常感谢大家对编程学习网网站的支持!
本文标题为:C#使用SqlConnection连接到SQL Server的代码示例
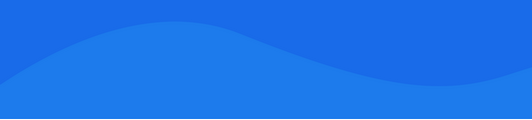
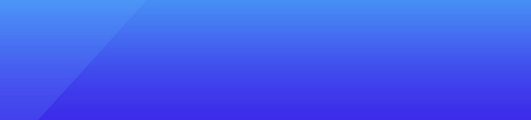
基础教程推荐
- C#控制台实现飞行棋小游戏 2023-04-22
- C#类和结构详解 2023-05-30
- 一个读写csv文件的C#类 2022-11-06
- linux – 如何在Debian Jessie中安装dotnet core sdk 2023-09-26
- ZooKeeper的安装及部署教程 2023-01-22
- C# List实现行转列的通用方案 2022-11-02
- C# 调用WebService的方法 2023-03-09
- winform把Office转成PDF文件 2023-06-14
- unity实现动态排行榜 2023-04-27
- C# windows语音识别与朗读实例 2023-04-27