这篇文章主要为大家详细介绍了Unity3D使用陀螺仪控制节点旋转,文中示例代码介绍的非常详细,具有一定的参考价值,感兴趣的小伙伴们可以参考一下
本文实例为大家分享了Unity3D陀螺仪控制节点旋转的具体代码,供大家参考,具体内容如下
/********************************************************************
Desc: 陀螺仪对相机的逻辑类。
*********************************************************************/
using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using UnityEngine;
namespace Game.Gyro
{
/// <summary>
/// 职责:
/// 1.实现陀螺仪对相机的影响和操作;
/// 2.尽量重现崩坏3的主界面驾驶舱效果;
/// </summary>
class GyroCamera : MonoBehaviour
{
#region 声明
/// <summary> 陀螺仪的输入类型 </summary>
public enum EGyroInputType
{
/// <summary> RotateRate </summary>
RotateRate,
/// <summary> RotateRateUniased </summary>
RotateRateUniased,
/// <summary> UserAcceleration </summary>
UserAcceleration,
}
#endregion
#region 控制变量
public float m_gyro_max_x = 15.0f;
public float m_gyro_max_y = 15.0f;
public float m_gyro_max_z = 15.0f;
#endregion
#region 变量
/// <summary> editor开发环境下的模拟陀螺仪输入 </summary>
public Vector3 m_editor_debug_input = Vector3.zero;
/// <summary> 陀螺仪的输入参数,用以控制相机 </summary>
public Vector3 m_gyro_input = Vector3.zero;
/// <summary> 当前的摄像机角度 </summary>
public Vector3 m_cur_euler = Vector3.zero;
/// <summary> 陀螺仪数据的更新频率 </summary>
public int m_upate_rate = 30;
/// <summary> 当前陀螺仪的输入输入类型 </summary>
public EGyroInputType m_gyro_input_type = EGyroInputType.RotateRate;
/// <summary> 陀螺仪的系数 </summary>
public float m_gyro_factor = 1.0f;
private Vector3 m_camera_init_euler = Vector3.zero;
private Transform mTransform;
#endregion
#region 访问接口
/// <summary> 陀螺仪的输入参数,用以控制相机 </summary>
protected Vector3 GyroInput
{
get
{
return m_gyro_input;
}
set
{
m_gyro_input = value;
}
}
/// <summary> 陀螺仪输入数据的类型 </summary>
protected EGyroInputType GyroInputType
{
get
{
return m_gyro_input_type;
}
set
{
m_gyro_input_type = value;
}
}
/// <summary> 陀螺仪的系数 </summary>
protected float GyroFactor
{
get
{
return m_gyro_factor;
}
set
{
m_gyro_factor = value;
}
}
/// <summary> 当前的旋转角 </summary>
protected Vector3 CurEuler
{
get
{
return m_cur_euler;
}
set
{
m_cur_euler = value;
}
}
#endregion
#region Unity
// Use this for initialization
void Start()
{
Input.gyro.enabled = true;
mTransform = gameObject.transform;
CurEuler = mTransform.localEulerAngles;
m_camera_init_euler = CurEuler;
}
/// <summary> 绘制UI,方便调试 </summary>
void OnGUI()
{
//GUI.Label(GetRect(0.1f, 0.05f), "Attitude: " + Input.gyro.attitude);
//GUI.Label(GetRect(0.1f, 0.15f), "Rotation: " + Input.gyro.rotationRate);
//GUI.Label(GetRect(0.1f, 0.25f), "RotationUnbiased: " + Input.gyro.rotationRateUnbiased);
//GUI.Label(GetRect(0.1f, 0.35f), "UserAcceleration: " + Input.gyro.userAcceleration);
//// 陀螺仪的系数
//{
// string t_factor_str = GUI.TextField(GetRect(0.7f, 0.05f), "" + GyroFactor);
// GyroFactor = float.Parse(t_factor_str);
/
沃梦达教程
本文标题为:Unity3D使用陀螺仪控制节点旋转
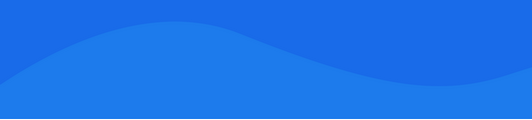
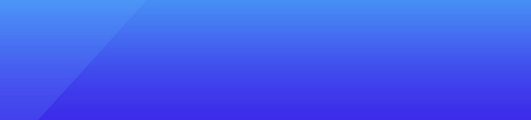
基础教程推荐
猜你喜欢
- C#控制台实现飞行棋小游戏 2023-04-22
- C# 调用WebService的方法 2023-03-09
- C# windows语音识别与朗读实例 2023-04-27
- ZooKeeper的安装及部署教程 2023-01-22
- C#类和结构详解 2023-05-30
- C# List实现行转列的通用方案 2022-11-02
- winform把Office转成PDF文件 2023-06-14
- unity实现动态排行榜 2023-04-27
- 一个读写csv文件的C#类 2022-11-06
- linux – 如何在Debian Jessie中安装dotnet core sdk 2023-09-26