这篇文章主要介绍了C# 模拟浏览器并自动操作的实例代码,文中讲解非常细致,帮助大家更好的理解和学习,感兴趣的朋友可以了解下
本文主要讲解通过WebBrowser控件打开浏览页面,并操作页面元素实现自动搜索功能,仅供学习分享使用,如有不足之处,还请指正。
涉及知识点
- WebBrowser:用于在WinForm窗体中,模拟浏览器,打开并导航网页。
- HtmlDocument:表示一个Html文档的页面。每次加载都会是一个全新的页面。
- GetElementById(string id):通过ID或Name获取一个Html中的元素。
- HtmlElement:表示一个Html标签元素。
- BackgroundWorker 后台执行独立操作的进程。
设计思路
主要采用异步等待的方式,等待页面加载完成,流程如下所示:
示例效果图
如下所示:加载完成后,自动输入【天安门】并点击搜索。
核心代码
加载新的页面,如下所示:
string url = "https://www.so.com/";
this.wb01.ScriptErrorsSuppressed = true;
this.wb01.Navigate(url);
注意:this.wb01.ScriptErrorsSuppressed = true;用于是否弹出异常脚本代码错误框
获取元素并赋值,如下所示:
string search_id = "input";
string search_value = "天安门";
string btn_id = "search-button";
HtmlDocument doc = this.wb01.Document;
HtmlElement search = doc.GetElementById(search_id);
search.SetAttribute("value", search_value);
HtmlElement btn = doc.GetElementById(btn_id);
btn.InvokeMember("click");
示例整体代码,如下所示:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace DemoExplorer
{
public partial class FrmExplorer : Form
{
private bool isLoadOk = false;
private BackgroundWorker bgWork;
public FrmExplorer()
{
InitializeComponent();
}
private void FrmExplorer_Load(object sender, EventArgs e)
{
bgWork = new BackgroundWorker();
bgWork.DoWork += bgWork_DoWork;
bgWork.RunWorkerCompleted += bgWork_RunWorkerCompleted;
string url = "https://www.so.com/";
this.wb01.ScriptErrorsSuppressed = true;
this.wb01.Navigate(url);
bgWork.RunWorkerAsync();
}
private void bgWork_RunWorkerCompleted(object sender, RunWorkerCompletedEventArgs e)
{
string search_id = "input";
string search_value = "天安门";
string btn_id = "search-button";
HtmlDocument doc = this.wb01.Document;
HtmlElement search = doc.GetElementById(search_id);
search.SetAttribute("value", search_value);
HtmlElement btn = doc.GetElementById(btn_id);
btn.InvokeMember("click");
}
private void bgWork_DoWork(object sender, DoWorkEventArgs e)
{
compWait();
}
private void compWait()
{
while (!isLoadOk)
{
Thread.Sleep(500);
}
}
private void wb01_DocumentCompleted(object sender, WebBrowserDocumentCompletedEventArgs e)
{
this.wb01.Document.Window.Error += new HtmlElementErrorEventHandler(Window_Error);
if (this.wb01.ReadyState == WebBrowserReadyState.Complete)
{
isLoadOk = true;
}
else
{
isLoadOk = false;
}
}
private void Window_Error(object sender, HtmlElementErrorEventArgs e)
{
e.Handled = true;
}
}
}
另外一种实现方式(MSHTML)
什么是MSHTML?
MSHTML是windows提供的用于操作IE浏览器的一个COM组件,该组件封装了HTML语言中的所有元素及其属性,通过其提供的标准接口,可以访问指定网页的所有元素。
涉及知识点
InternetExplorer 浏览器对象接口,其中DocumentComplete是文档加载完成事件。
HTMLDocumentClass Html文档对象类,用于获取页面元素。
IHTMLElement 获取页面元素,通过setAttribute设置属性值,和click()触发事件。
示例核心代码
如下所示:
namespace AutoGas
{
public class Program
{
private static bool isLoad = false;
public static void Main(string[] args)
{
string logUrl = ConfigurationManager.AppSettings["logUrl"]; //登录Url
string uid = ConfigurationManager.AppSettings["uid"];//用户名ID
string pid = ConfigurationManager.AppSettings["pid"];//密码ID
string btnid = ConfigurationManager.AppSettings["btnid"];//按钮ID
string uvalue = ConfigurationManager.AppSettings["uvalue"];//用户名
string pvalue = ConfigurationManager.AppSettings["pvalue"];//密码
InternetExplorer ie = new InternetExplorerClass();
ie.DocumentComplete += Ie_DocumentComplete;
object c = null;
ie.Visible = true;
ie.Navigate(logUrl, ref c, ref c, ref c, ref c);
ie.FullScreen = true;
compWait();
try
{
HTMLDocumentClass doc = (HTMLDocumentClass)ie.Document;
IHTMLElement username = doc.getElementById(uid);
IHTMLElement password = doc.getElementById(pid);
IHTMLElement btn = doc.getElementById(btnid);
//如果有session,则自动登录,不需要输入账号密码
if (username != null && password != null && btn != null)
{
username.setAttribute("value", uvalue);
password.setAttribute("value", pvalue);
btn.click();
}
}
catch (Exception ex) {
}
}
public static void compWait() {
while (!isLoad)
{
Thread.Sleep(200);
}
}
/// <summary>
///
/// </summary>
/// <param name="pDisp"></param>
/// <param name="URL"></param>
private static void Ie_DocumentComplete(object pDisp, ref object URL)
{
isLoad = true;
}
}
}
以上就是C# 模拟浏览器并自动操作的实例代码的详细内容,更多关于C# 模拟浏览器并自动操作的资料请关注得得之家其它相关文章!
本文标题为:C# 模拟浏览器并自动操作的实例代码
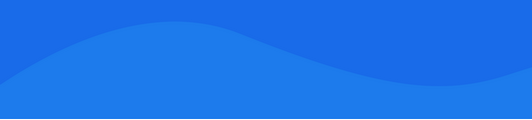
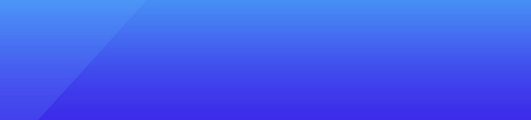
基础教程推荐
- C# List实现行转列的通用方案 2022-11-02
- C# 调用WebService的方法 2023-03-09
- C# windows语音识别与朗读实例 2023-04-27
- ZooKeeper的安装及部署教程 2023-01-22
- 一个读写csv文件的C#类 2022-11-06
- C#控制台实现飞行棋小游戏 2023-04-22
- linux – 如何在Debian Jessie中安装dotnet core sdk 2023-09-26
- C#类和结构详解 2023-05-30
- winform把Office转成PDF文件 2023-06-14
- unity实现动态排行榜 2023-04-27